Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial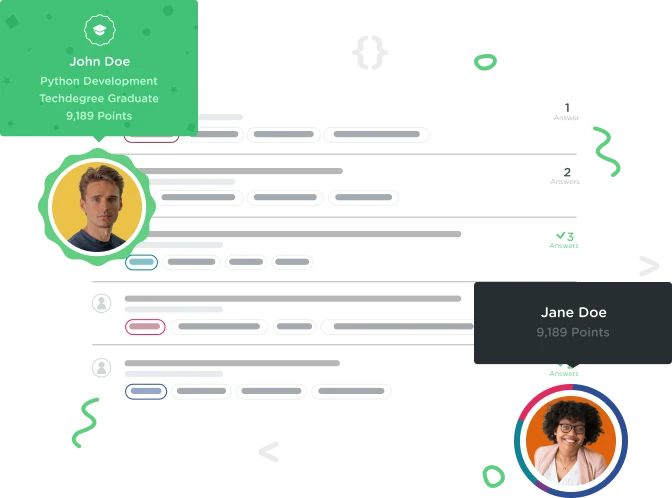

Sam Morpeth
8,756 PointsTemplate Literal Practice - A step further
Hi guys,
So I'm trying to take the card building script a bit further by letting users add their own cards. But I'm having a few problems. Number 1 being that after I continue the loop and add another card it replaces the initial one. I have a feeling because it's just looping through and resetting the values, but I have no idea how to fix it. Maybe with for loops?
The other problem being that the "hair colour, eye colour and favourite game" values are "undefined" in the new user created cards. They show up fine in the objects I pre-built such as "sam" and "kate", but for some reason are undefined in the user created cards.
Note: I know that I'm using a mix of "var, let and const" for variables, but it's because I don't fully understand them yet and I'm trying replace/add them wherever I can understand them. Also any other way to refactor stuff would you be really helpful.
Here's the code I'm using:
var newPeople = {};
let createNewPerson;
const createProfile = () => {
let createNewPerson = prompt(`Would you like to create a new profile card for someone? (Y/N)`);
while (createNewPerson === `y` || createNewPerson === `Y`) {
const name = prompt(`What's their name?`);
newPeople["name"] = name;
var height = prompt(`How tall are they?`);
newPeople[`height`] = height;
var eyeColour = prompt(`What colour eyes do they have?`);
newPeople[`eyecolour`] = eyeColour;
var hairColour = prompt(`What colour hair do they have?`);
newPeople["haircolour"] = hairColour;
var moons = prompt(`How many moons orbit them?`);
newPeople["moons"] = moons;
var favouriteGame = prompt(`What's their favourite game?`);
newPeople["favouritegame"] = favouriteGame;
var hobbies = prompt(`What hobbies do they do/like?`);
newPeople["hobbies"] = hobbies;
people.push(newPeople);
let createNewPerson = prompt(`Would you like to add another person? (Y/N)`);
if (createNewPerson === `N` || createNewPerson === `n`) {
break;
}
}
}
const createPersonHTML = (person) => {
return `
<div class="card">
<div>
<img src="https://placeimg.com/640/480/nature" alt="${person.name}">
</div>
<h2> ${person.name} </h2>
<p> ${person.hobbies} </p>
<h3>Planet Profile</h3>
<ul>
<li><strong>Height: </strong> ${person.height} </li>
<li><strong>Moons: </strong> ${person.moons} </li>
<li><strong>Eye colour: </strong> ${person.eyeColour} </li>
<li><strong>Favourite game: </strong> ${person.favouriteGame} </li>
<li><strong>Hair colour: </strong> ${person.hairColour} </li>
</ul>
<p> </p>
</div>`;
}
const people = [
{
name: `Sam`,
height: `165cm`,
eyeColour: `blue`,
hairColour: `brown`,
moons: `2`,
favouriteGame: `Pokemon Gold`,
hobbies: `playing guitar, cooking, running, playing videogames, programming`
},
{
name: `Kate`,
height: `177cm`,
eyeColour: `blue`,
hairColour: `blonde`,
moons: `8`,
favouriteGame: `tag`,
hobbies: `immitating bird calls, crashing birthday parties`
}
];
createProfile();
document.querySelector('body').innerHTML = people.map(person => createPersonHTML(person)).join('');
``
2 Answers
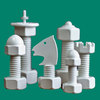
Steven Parker
230,274 PointsThe code creates a single global object named "newPeople" and then keeps repopulating it in the loop, which causes the data to be overwritten. You can fix that by creating a new object inside the loop for each person.
Then the undefined values are caused by setting different property names than the ones that will be printed out. For example, the code sets "newPeople['eyecolour']
" (with a lower-case "c"), but the value printed is "${person.eyeColour}
" (with a capital "C"). Use the same name (with same capitalization) in both places to fix that.
And if a variable is assigned and then referenced only once with no code in between, you can probably refactor the code to eliminate the variable completely. Example:
var hobbies = prompt(`What hobbies do they do/like?`);
newPeople["hobbies"] = hobbies;
// the above can be replaced by:
newPeople["hobbies"] = prompt(`What hobbies do they do/like?`);

Sam Morpeth
8,756 PointsEverything you said worked perfectly! Thanks so much!