Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial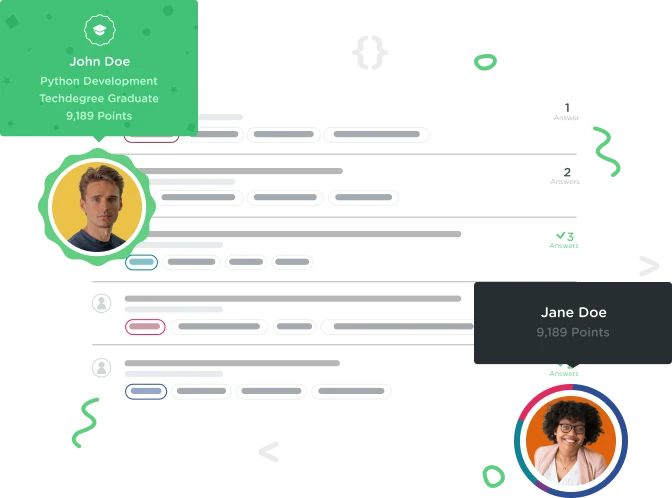
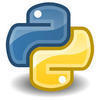
Gerald Wells
12,763 PointsTemplates not found in django
when i first started going through the django course work I don't think I ever had to define where my templates where located, they were simply in a template directory located with in the project. When I restarted my Project I had to tell django in settings.py where my templates where located and now I am having an issue calling templates with in the app itself. Any thoughts as to what I am doing wrong? The Current path to the template i am trying to pull is /home/jdubs/Projects/time_clock/drivers/templates/drivers
urls.py inside of app
from django.conf.urls import url
from django.contrib import admin
from . import views
urlpatterns = [
url(r'^clockin/$', views.clock_in_view, name="clockin"),
]
urls.py in project trunk
"""time_clock URL Configuration
The `urlpatterns` list routes URLs to views. For more information please see:
https://docs.djangoproject.com/en/1.9/topics/http/urls/
Examples:
Function views
1. Add an import: from my_app import views
2. Add a URL to urlpatterns: url(r'^$', views.home, name='home')
Class-based views
1. Add an import: from other_app.views import Home
2. Add a URL to urlpatterns: url(r'^$', Home.as_view(), name='home')
Including another URLconf
1. Add an import: from blog import urls as blog_urls
2. Import the include() function: from django.conf.urls import url, include
3. Add a URL to urlpatterns: url(r'^blog/', include(blog_urls))
"""
from django.conf.urls import include, url
from django.contrib import admin
from . import views
urlpatterns = [
url(r'^admin/', admin.site.urls),
url(r'^contact/$', views.contact_view, name='contact'),
url(r'^drivers/', include('drivers.urls')),
url(r'^$', views.hello_world),
]
views.py:
def contact_view(request):
form = forms.ContactForm()
if request.method == 'POST':
form = forms.ContactForm(request.POST)
if form.is_valid():
send_mail(
'Email from {}'.format(form.cleaned_data['name']),
form.cleaned_data['message'],
'{name} <{email}>'.format(**form.cleaned_data),
['G@gmail.com']
)
messages.add_message(request, messages.SUCCESS, "Message sent!")
return HttpResponseRedirect(reverse('contact'))
return render(request, 'contactform.html', {'form': form})
settings.py
TEMPLATES = [
{
'BACKEND': 'django.template.backends.django.DjangoTemplates',
'DIRS': [
'/home/jdubs/Projects/time_clock/time_clock/templates',
'/home/jdubs/Projects/time_clock/drivers/templates/drivers',
],
'APP_DIRS': True,
'OPTIONS': {
'context_processors': [
'django.template.context_processors.debug',
'django.template.context_processors.request',
'django.contrib.auth.context_processors.auth',
'django.contrib.messages.context_processors.messages',
],
},
},
]
6 Answers
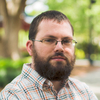
Kenneth Love
Treehouse Guest TeacherDjango, by default, automatically looks for templates in a templates
directory in each app. So project/app1/templates/
is automatically loaded. Most of the time you'd use project/app1/templates/app1/
so that your templates are namespaced.
If you want to have some project-wide templates, you can add extra directories to the DIRS
key in the TEMPLATES
setting, like you've done (you should really use the BASE_DIR
variable, though, to make it easier to find the paths).
Unless your paths are wrong, or need to end in a slash (I couldn't find yay or nay on this), I don't see any settings that are wrong. What errors are you experiencing?
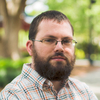
Kenneth Love
Treehouse Guest TeacherOK, /drivers/clockin.html
isn't a valid URL. This is true because none of your URLs end in .html
.
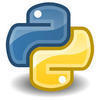
Gerald Wells
12,763 PointsI thought I was supposed to use the path to the view, and the view redirected to the template.html?
Could you please give me an example to run off of so I understand better?
Thank you very much as well for your quick reply!
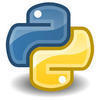
Gerald Wells
12,763 PointsI rewrote my url to state:
"""time_clock URL Configuration
The `urlpatterns` list routes URLs to views. For more information please see:
https://docs.djangoproject.com/en/1.9/topics/http/urls/
Examples:
Function views
1. Add an import: from my_app import views
2. Add a URL to urlpatterns: url(r'^$', views.home, name='home')
Class-based views
1. Add an import: from other_app.views import Home
2. Add a URL to urlpatterns: url(r'^$', Home.as_view(), name='home')
Including another URLconf
1. Add an import: from blog import urls as blog_urls
2. Import the include() function: from django.conf.urls import url, include
3. Add a URL to urlpatterns: url(r'^blog/', include(blog_urls))
"""
from django.conf.urls import include, url
from django.contrib import admin
from . import views
urlpatterns = [
url(r'^admin/', admin.site.urls),
url(r'^contact/$', views.contact_view, name='contact'),
url(r'^drivers/clockin.html', include('drivers.urls')),
url(r'^$', views.clock_in_or_out),
]
Page not found (404)
Request Method: GET
Request URL: http://127.0.0.1:8000/drivers/clockin.html
Using the URLconf defined in time_clock.urls, Django tried these URL patterns, in this order:
^admin/
^contact/$ [name='contact']
^drivers/clockin.html ^clockin/$ [name='clockin']
^$
The current URL, drivers/clockin.html, didn't match any of these.
You're seeing this error because you have DEBUG = True in your Django settings file. Change that to False, and Django will display a standard 404 page.
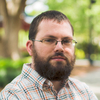
Kenneth Love
Treehouse Guest TeacherURLs don't point to templates. URLs point to views. Some views return a response that includes a rendered template. Since you're using a form, are you on Django Forms already? If so, you might want to revisit Django Basics for some of this.
If you go to /drivers/clockin/
(using the first set of URLs you posted), you should get your clock_in_view
view. You wouldn't use .html
in this because it's not in the URL route you created. The format of the template isn't important to the URL or, really, even the view at all.
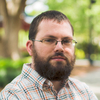
Kenneth Love
Treehouse Guest TeacherWith your new URL, it would be /drivers/clockin.html/clockin/
which doesn't make any sense at all.
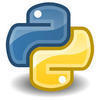
Gerald Wells
12,763 PointsI fixed it to the path you are stating, but I cant get it to call any of my templates that are inside my app project, its only allowing me to pull my contact form inside my projects views. I have one view located there and that is contact_view.
also could you give me an example on how to use BASE_DIr, maybe thats my issue?
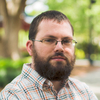
Kenneth Love
Treehouse Guest TeacherWe used it in Django Basics. os.path.join(BASE_DIR, 'templates')
will give you /project/templates/
as a templates directory.
You don't call templates, though. You use them in views when you render()
.
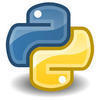
Gerald Wells
12,763 PointsWhat video was that? I need to go back and watch it.
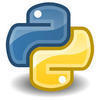
Gerald Wells
12,763 PointsSo I went back through django basics created a new dir inside my home dir and started a new project and everything is working fine... Knock on wood.
I have a theory as to why it wasn't working. I had multiple projects inside of a dir. Do you think because I didn't use virtualenv it was messing up how django works?
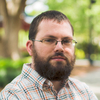
Kenneth Love
Treehouse Guest TeacherThat shouldn't affect it at all.
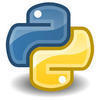
Gerald Wells
12,763 Pointshmm, well everything is fixed, I had to redo the whole project, thank you for telling me to redo Django Basics, I understand the structure a lot better now.

Brent Lageson
4,452 PointsI know this is an old post but I was searching for an answer because I was having the exact same issue. I originally had created a templates folder on the root of the project then built a folder in that templates for my app. I was then trying to move the templates into the app where they should be but left the file structure there, so django was looking in the original location and not finding it. Once I removed the original folder it started working.
Gerald Wells
12,763 PointsGerald Wells
12,763 PointsIt's throwing a 404 and stating that the template could not be found in any of my specified urls.
Kenneth Love
Treehouse Guest TeacherKenneth Love
Treehouse Guest TeacherOK. Copy and paste the stacktrace from the debug output? There should be a handy-dandy link to copy it all quickly.
Gerald Wells
12,763 PointsGerald Wells
12,763 Points