Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial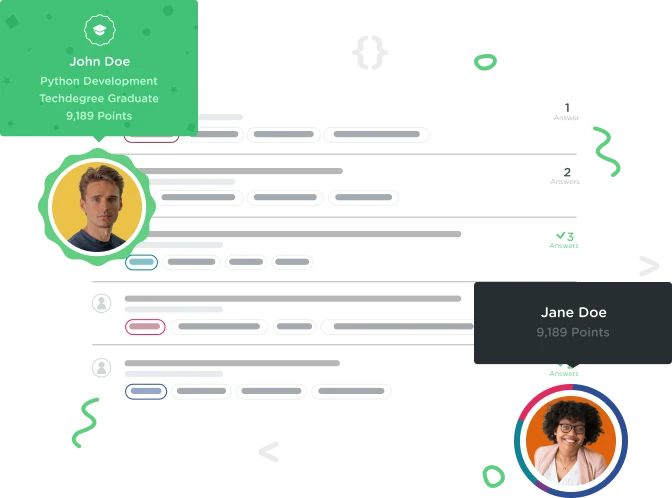
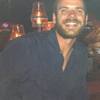
Aaron Brown
2,551 PointsTerminal output is different from Workspaces output
Hello!
I am working on my Terminal opposed to the workspaces, and I should note that I am using Python3.
When Kenneth inputs azog = monster.Goblin()
he gets assignment of azog to the Goblin subclass of Monster. However, I get:
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
NameError: name 'monster' is not defined
But when I leave out '.monster' and just insert: azog = Goblin
, the assignment works just fine and I get:
<class '__main__.Goblin'>
What's going on? Here's my code just to make sure I didn't make some mistake somewhere.
import random
COLORS = ['yellow', 'red', 'blue', 'green']
class Monster:
min_hit_points = 1
max_hit_points = 1
min_experience = 1
max_experience = 1
weapon = 'sword'
sound = 'roar'
def __init__(self, **kwargs):
self.hit_points = random.randint(self.min_hit_points, self.max_hit_points)
self.experience = random.randint(self.min_experience, self.max_experience)
self.color = random.choice(COLORS)
for key, value in kwargs.items():
setattr(self, key, value)
def battlecry(self):
return self.sound.upper()
# passing the Monster class inside of parentheses, tells Python that Goblin is a subclass of monster, which means Goblin has all the attrs of Monsters unless modified within Goblin
class Goblin(Monster):
max_hit_points = 3
max_experience = 2
sound = 'squeak'
class Troll(Monster):
min_hit_points = 3
max_hit_points = 5
min_experience = 2
max_experience = 6
sound = 'growl'
class Dragon(Monster):
min_hit_points = 5
max_hit_points = 10
min_experience = 6
max_experience = 10
sound = 'raaaaaaaaaaaar'
Thank you! I look forward to any input, and please let me know if this is not clear.
Best, Aaron
1 Answer

Trevor Skelton
1,101 PointsIn your post you say Kenneth typed 'azog = monster.Goblin()'. I watched the video and saw him type 'azog = Goblin()'. If you use 'from monster import Goblin'. 'azog = Goblin()' should work. The reason you would use monster.Goblin() was to get the class out of the monster file by just typing 'import monster'. The way you import changes the line. e.g.
from monster import Goblin azog = Goblin()
or
import monster azog = monster.Goblin()
Aaron Brown
2,551 PointsAaron Brown
2,551 PointsThanks, Trevor!
Actually. I ended up figuring this out a few minutes after I posted. Why does it call differently depending on how I import? I feel this is probably an important concept to understand when moving forward with projects.
Aaron
Trevor Skelton
1,101 PointsTrevor Skelton
1,101 PointsI found the best answer I could find for you. I tried to think of a way to explain it, but this guy has a better explanation. I hope this helps with your question.
Link is here
Aaron Brown
2,551 PointsAaron Brown
2,551 PointsHey, Trevor! Thanks for that link. It was incredibly helpful.