Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial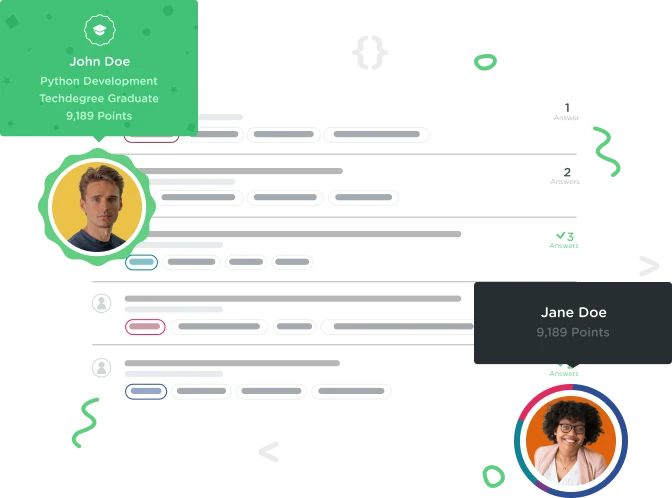

amyyxt
2,227 PointsTernary If Operation
Hello!
I was able to solve the Method Interactivity challenge using an if-else block, but when I tried to write basically the same code using the ternary if operator, it doesn't work. I tried it out in Workspace, and what comes back is the message "SyntaxError: invalid syntax".
The same message has occurred when I use "pass," "break," and "continue" with the ternary if operator. Can you explain why the error occurs when using the ternary if operator with these keywords?
Thanks!
class Student:
name = "Your Name"
def praise(self):
return "You inspire me, {}".format(self.name)
def reassurance(self):
return "Chin up, {}. You'll get it next time!".format(self.name)
def feedback(self, grade):
(return self.praise()) if grade > 50 else (return self.reassurance())
2 Answers
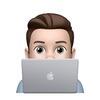
Cameron S
20,537 PointsOkay, I must have ignored the title of your post so I didn't realize you were asking about a ternary option- sorry about that! to put it simply, they way you wrote your return statement is "invalid syntax" for python. I am not 100% certain on this but I believe, originally, ternary options were not included in python because Guido Van Rossum found them to be difficult to read, etc. which goes against python's design choices (to make code expressive and easy to read).
To make the function more expressive try something like this:
def feedback(self, grade):
return [self.praise() if grade > 50 else self.reassurance()]
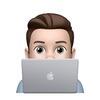
Cameron S
20,537 PointsThe logic and syntax is incorrect. What you need to do is to start off with your if statement to begin to workout the problem.
Start by making sure grade is greater than 50. IF it is, return the praise()
method.
Then return the reassurance()
method outside of the if
block as it will only happen if the if
block is false.
Below is my code:
def feedback(self, grade):
if grade > 50:
return self.praise()
return self.reassurance()

amyyxt
2,227 PointsHi Cameron,
Thanks for responding!
I initially wrote code similar to yours and solved the challenge, but I wanted to try it out with the ternary if operator. When I tried the ternary if operator, it didn't work. (The ternary if operator allows single-line if-else expressions and is handy alternative for some single-statement if-else blocks. It was introduced/mentioned briefly in a previous video.)
I know it's a syntax error because the error message said so. What I don't understand is the specific problem or the details of the error. Since the same error message pops up for other keyword statements, such as pass, break, and continue, I suspect the error involves keyword statements being a problem, but I don't know why and want to understand that.
amyyxt
2,227 Pointsamyyxt
2,227 PointsAh, I see! The brackets make it much clearer how Python reads that line, which was my problem.
And I think I'd have to agree with von Rossum's sentiment. The reason why I was trying to use a ternary if operator is that I see them every once in a while in Python, and I'd never seen them before (I have very limited C++ and MATLAB experience), so I thought I'd familiarize myself with the ternary if operator.