Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial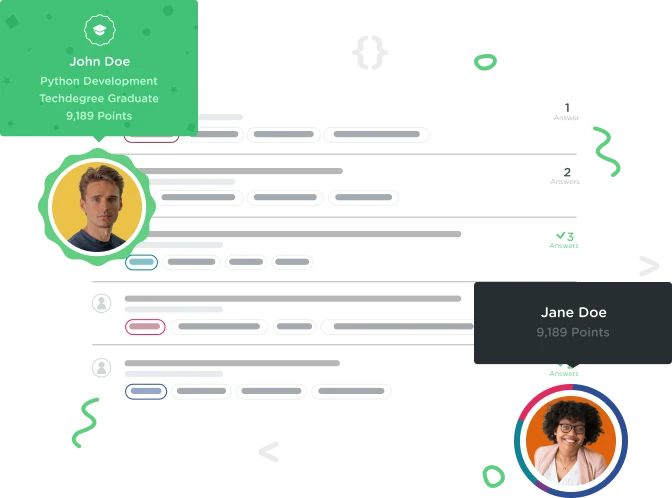
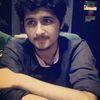
Prakhar Choudhary
10,305 PointsTests Passed, yet on submission it throws the message "Bummer! Try Again"
My code is successfully passing all the tests in the app_tests.py
. However, the challenge is throwing the message "Bummer! Try Again". I have also commented out the app.run(debug=DEBUG, host=HOST, port=PORT)
from flask import Flask, g, render_template, flash, redirect, url_for
from flask_bcrypt import check_password_hash
from flask_login import (LoginManager, login_user,
logout_user, login_required, current_user)
import forms
import models
DEBUG = True
PORT = 8000
HOST = '0.0.0.0'
app = Flask(__name__)
app.secret_key = 'youdontknowmysecretkey'
login_manager = LoginManager()
login_manager.init_app(app)
login_manager.login_view = 'login'
@login_manager.user_loader
def load_user(userid):
try:
return models.User.get(models.User.id == userid)
except models.DoesNotExist:
return None
@app.before_request
def before_request():
"""Connect to the database before each request."""
g.db = models.db
g.db.get_conn()
g.user = current_user
@app.after_request
def after_request(response):
"""Close the database connection after each request."""
g.db.close
return response
@app.route('/register', methods=('GET', 'POST'))
def register():
form = forms.SignUpForm()
if form.validate_on_submit():
flash("Yay, you registered!", "success")
models.User.create_user(
email = form.email.data,
password = form.password.data
)
return redirect(url_for('index'))
return render_template('register.html', form=form)
@app.route('/login', methods=('GET', 'POST'))
def login():
form = forms.LoginForm()
if form.validate_on_submit():
try:
user = models.User.get(models.User.email == form.email.data)
except models.DoesNotExist:
flash("Email is incompatible", "error")
else:
if check_password_hash(user.password, form.password.data):
login_user(user)
flash("You are logged in", "success")
return redirect(url_for('index'))
else:
flash("Password does not match", "error")
return render_template('login.html', form=form)
@app.route('/logout')
@login_required
def logout():
logout_user()
flash("You've been logged out! Come back soon!", "success")
return redirect(url_for('index'))
@app.route('/taco', methods=('GET','POST'))
@login_required
def taco():
form = forms.TacoForm()
if form.validate_on_submit():
models.Taco.create(user = g.user._get_current_object(),
protein = form.protein.data,
shell = form.shell.data,
cheese = form.cheese.data,
extras = form.extras.data.strip()
)
flash("Your taco has been added!!", "success")
return redirect(url_for('index'))
return render_template('taco.html', form=form)
@app.route('/')
def index():
try:
user = g.user
tacos = user.get_tacos()
return render_template('index.html', tacos=tacos)
except:
return render_template('index.html', tacos=None)
if __name__== '__main__':
models.initialize()
try:
with models.db.transaction():
models.User.create_user(
email = 'billy@example.com',
password = 'password'
)
except ValueError:
pass
#app.run(debug=DEBUG, host=HOST, port=PORT)
from flask_login import UserMixin
from flask_bcrypt import generate_password_hash
from peewee import *
db = SqliteDatabase('tacos.db')
class User(UserMixin, Model):
email = CharField(unique=True)
password = CharField(max_length=100)
class Meta:
database = db
def get_tacos(self):
return Taco.select().where(Taco.user == self)
def get_stream(self):
return Taco.select().where(
(Taco.user == self)
)
@classmethod
def create_user(cls, email, password):
try:
cls.create(
email = email,
password = generate_password_hash(password))
except IntegrityError:
raise ValueError("User already exists")
class Taco(Model):
protein = CharField()
shell = CharField()
cheese = BooleanField(default=True)
extras = TextField()
user = ForeignKeyField(
rel_model = User,
related_name = 'tacos'
)
class Meta:
database = db
def initialize():
db.connect()
db.create_tables([User, Taco], safe=True)
db.close()
from flask_wtf import FlaskForm
from wtforms import StringField, PasswordField, TextAreaField, BooleanField
from wtforms.validators import (DataRequired, Regexp, ValidationError, Email,
Length, EqualTo)
from models import User
def email_exists(form, field):
if User.select().where(User.email == field.data).exists():
raise ValidationError('User with that email already exists.')
class SignUpForm(FlaskForm):
email = StringField(
'Email',
validators=[
DataRequired(),
Email(),
email_exists
])
password = PasswordField(
'Password',
validators=[
DataRequired(),
Length(min=2),
EqualTo('password2', message='Passwords must match')
])
password2 = PasswordField(
'Confirm Password',
validators=[DataRequired()]
)
class LoginForm(FlaskForm):
email = StringField(
'Email',
validators=[
DataRequired(),
Email()
])
password = PasswordField(
'Password',
validators=[
DataRequired(),
])
class TacoForm(FlaskForm):
protein = StringField('proteins', validators=[DataRequired()])
shell = StringField('shell', validators=[DataRequired()])
cheese = BooleanField('cheese?', validators=[DataRequired()])
extras = StringField('extras')
<!doctype html>
<html>
<head>
<title>Tacocat</title>
<link rel="stylesheet" href="/static/css/normalize.css">
<link rel="stylesheet" href="/static/css/skeleton.css">
<link rel="stylesheet" href="/static/css/tacocat.css">
</head>
<body>
<header>
<div class="row">
<div class="grid-33">
<a href="{{ url_for('index') }}" class="icon-logo"></a>
</div>
<div class="grid-33">
<!-- Say Hi -->
<h1>Hello {% if current_user.is_authenticated %} {{ current_user.username }}{% endif %}!</h1>
</div>
<div class="grid-33">
<!-- Log in/Log out -->
{% if current_user.is_authenticated %}
<a href="{{ url_for('logout') }}" class="icon-power" title="Log out">Log Out</a>
{% else %}
<a href="{{ url_for('login') }}" class="icon-power" title="Log in">Log In</a>
<a href="{{ url_for('register') }}" class="icon-profile" title="SignUp">Sign up</a>
{% endif %}
</div>
</div>
</header>
{% with messages=get_flashed_messages() %}
{% if messages %}
<div class="messages">
{% for message in messages %}
<div class="message">
{{ message }}
</div>
{% endfor %}
</div>
{% endif %}
{% endwith %}
<div class="container">
<div class="row">
<div class="u-full-width">
<nav class="menu">
<!-- menu goes here -->
{% if current_user.is_authenticated %}
<a href="{{ url_for('taco') }}">add a new taco</a>
{% endif %}
</nav>
{% block content %}{% endblock %}
</div>
</div>
<footer>
<p>An MVP web app made with Flask on <a href="http://teamtreehouse.com">Treehouse</a>.</p>
</footer>
</div>
</body>
</html>
{% extends 'layout.html' %}
{% block content %}
<h2>Tacos</h2>
{% if tacos %}
<table class="u-full-width">
<thead>
<tr>
<th>Protein</th>
<th>Cheese?</th>
<th>Shell</th>
<th>Extras</th>
</tr>
</thead>
<tbody>
{% for taco in tacos %}
<tr>
<th>{{ taco.protein }}</th>
<th>{{ taco.cheese }}</th>
<th>{{ taco.shell }}</th>
<th>{{ taco.extras }}</th>
</tr>
{% endfor %}
</tbody>
</table>
{% else %}
<p>No tacos yet!</p>
{% endif %}
{% endblock %}
1 Answer
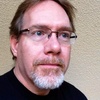
Chris Freeman
Treehouse Moderator 68,423 PointsIt looks like there is an indention error, line 41, tacocat.py: "def register():
". Also depending on which version the workspaces you are using, FlaskForm
may not be available. The challenge can be solved using flask_wtf
class Form
.
With these two fixed test_logged_out_menu fails with the error in workspaces:
treehouse:~/workspace$ python app_tests.py
........F..
======================================================================
FAIL: test_logged_out_menu (__main__.UserViewsTestCase)
----------------------------------------------------------------------
Traceback (most recent call last):
File "app_tests.py", line 113, in test_logged_out_menu
self.assertIn("sign up", rv.get_data(as_text=True).lower())
AssertionError: 'sign up' not found in '<!doctype html>\n<html>\n <head>\n <title>tacocat</title>\n <link rel="stylesheet" href="/static/css/normalize.css">
\n <link rel="stylesheet" href="/static/css/skeleton.css">\n <link rel="stylesheet" href="/static/css/tacocat.css">\n </head>\n <body>\n <header>\n
<div class="row">\n <div class="grid-33">\n <a href="/" class="icon-logo"></a>\n </div>\n <div class="gr
id-33">\n <!-- say hi -->\n <h1>hello !</h1>\n </div>\n <div class="grid-33">\n <!-- log in/log out
-->\n \n <a href="/logout" class="icon-power" title="log out">log out</a>\n \n\n </div>\n </div>\n
</header>\n \n \n \n\n <div class="container">\n <div class="row">\n <div class="u-full-width">\n <nav class="menu">\n
<!-- menu goes here -->\n \n <a href="/taco">add a new taco</a>\n \n </nav>\n \n<h2>tacos</h2>\n \n <p>no
tacos yet!</p>\n \n\n </div>\n </div>\n <footer>\n <p>an mvp web app made with flask on <a href="http://teamtreehouse.com">treehouse</a
>.</p>\n </footer>\n </div>\n </body>\n</html>'
----------------------------------------------------------------------
Ran 11 tests in 13.257s
This error is sometimes caused by a version difference between the challenge checker module versions and your test environment versions.
In layout.html
, try adding parentheses to the function call current_user.authenticated()
. This fixes the above error. I think there is a mention of this error in one of the Teacher's Notes
Post back if you have more questions! Good Luck!!