Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial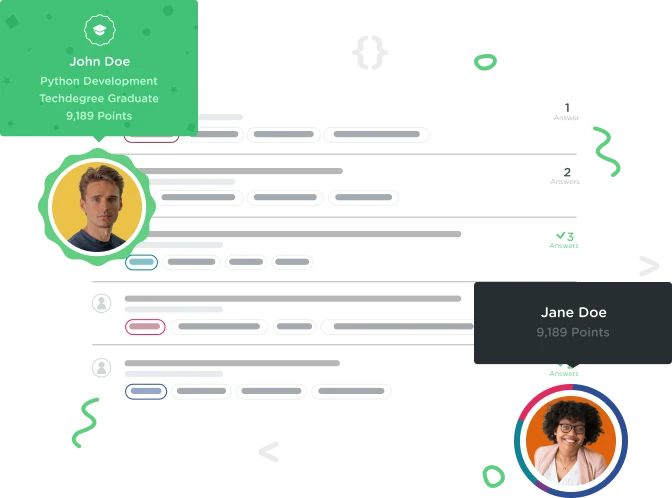
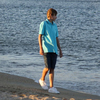
Tom Coomer
1,331 PointsText Field max length on Mac OSX
I have created an app for iOS that uses the code below to limit the length of a string in the text field to 1. I am now trying to create the app to run on Mac but cannot seem to work out how to do the same. Thanks (MAXLENGTH is = 1)
- (BOOL)textField:(UITextField *) textField shouldChangeCharactersInRange:(NSRange)range replacementString:(NSString *)string {
NSUInteger oldLength = [textField.text length];
NSUInteger replacementLength = [string length];
NSUInteger rangeLength = range.length;
NSUInteger newLength = oldLength - rangeLength + replacementLength;
BOOL returnKey = [string rangeOfString: @"\n"].location != NSNotFound;
return newLength <= MAXLENGTH || returnKey;
}
1 Answer
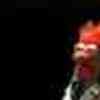
James Andrews
7,245 PointsThe differences is that iOS uses different interface libraries than OS X. If you go to the your .xib file click on a text field and go to the Identity Inspector. You'll notice that it inherits from NSTextfield instead of UITextfield. NSTextfield is part of Appkit and if you look at the docs it doesn't have any methods or attributes. Therefore you need to capture the stringValue as an NSString and then get the length of the NSString.
so something like this would probably fix your problem, but I can't say for certain as I haven't tested this.
NSString *textfieldString = [textField stringValue];
NSUInteger oldLength = [textfieldString length];
NSUInteger replacementLength = [string length];
NSUInteger rangeLength = range.length;
NSUInteger newLength = oldLength - rangeLength + replacementLength;
BOOL returnKey = [string rangeOfString: @"\n"].location != NSNotFound;
return newLength <= MAXLENGTH || returnKey;
Tom Coomer
1,331 PointsTom Coomer
1,331 PointsExcellent. Thank you. I will try it when I get back to my computer and let you know. Thanks again.
Tom Coomer
1,331 PointsTom Coomer
1,331 PointsHow should I link this to the Text field? Should it be an IBAction?
James Andrews
7,245 PointsJames Andrews
7,245 Pointsno same as iOS textfields go to an Outlet not an action. actions are for buttons etc..
Tom Coomer
1,331 PointsTom Coomer
1,331 PointsOkay. Thanks. I was wondering if you also know how I would clear a textfield when it becomes in focus (first responder)? Thanks
James Andrews
7,245 PointsJames Andrews
7,245 PointsNot sure, maybe set a delegate for it. I've very little Objective C experience making Mac apps. I had learned a long time ago and gave up because I didn't have the time to dedicate it. What I gave you I got from a google search ;-)
Tom Coomer
1,331 PointsTom Coomer
1,331 PointsAh. Right. Ill keep looking. Thanks again.