Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial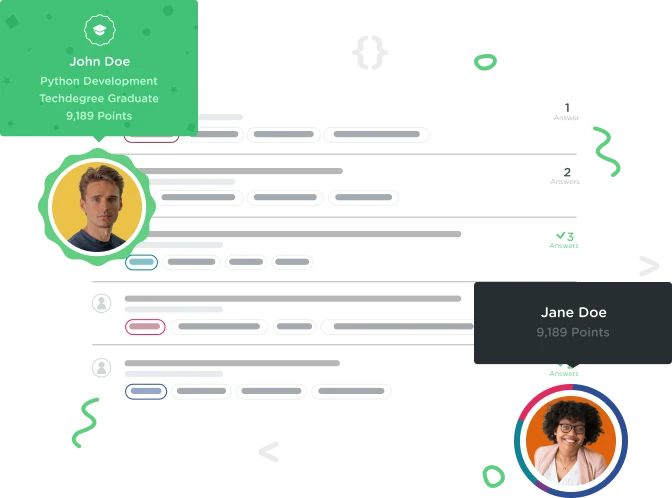
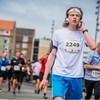
Bastian Petersson
9,164 PointsText field not moving
When I run the app and click on the text field it still doesn't work. I have no errors. Can anyone else spot the problem?
import UIKit
class ViewController: UIViewController {
@IBOutlet var nameTextField: UITextField!
@IBOutlet var textFieldBottumConstraint: NSLayoutConstraint!
override func viewDidLoad() {
super.viewDidLoad()
NotificationCenter.default.addObserver(self, selector: #selector(ViewController.keyboardWillShow), name: Notification.Name.UIKeyboardWillHide, object: nil)
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
override func prepare(for segue: UIStoryboardSegue, sender: Any?) {
if segue.identifier == "startAdventure" {
do {
if let name = nameTextField.text {
if name == "" {
throw AdventureError.nameNotProvided
} else {
guard let pageController = segue.destination as? PageController else {
return }
pageController.page = Adventure.story(withName: name)
}
}
} catch AdventureError.nameNotProvided {
let alertController = UIAlertController(title: "Name Not Provided", message: "Provide a name to start the story", preferredStyle: .alert)
let action = UIAlertAction(title: "OK", style: .default, handler: nil)
alertController.addAction(action)
present(alertController, animated: true, completion: nil)
}catch let error {
fatalError("\(error.localizedDescription)")
}
}
}
@objc func keyboardWillShow(_ notification: Notification) {
if let info = notification.userInfo, let keyboardFrame = info["UIKeyboardFrameEndUserInfoKey"] as? NSValue {
let frame = keyboardFrame.cgRectValue
textFieldBottumConstraint.constant = frame.size.height + 10
UIView.animate(withDuration: 0.8) {
self.view.layoutIfNeeded()
}
}
}
deinit {
NotificationCenter.default.removeObserver(self)
}
}
2 Answers
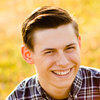
Caleb Kleveter
Treehouse Moderator 37,862 PointsThe only difference I saw between your code and Pasan's was the #selector
literal for the keyboard notification:
NotificationCenter.default.addObserver(
self,
selector: #selector(ViewController.keyboardWillShow), // <== This argument
name: Notification.Name.UIKeyboardWillHide,
object: nil
)
Pasan's implementation has the parameter list of the method:
#selector(ViewController.keyboardWillShow(_:)),
Hope that fixes it!
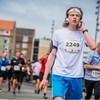
Bastian Petersson
9,164 PointsThis was exactly what was wrong. I was able to figure it out myself. But thanks for the help anyway!?