Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial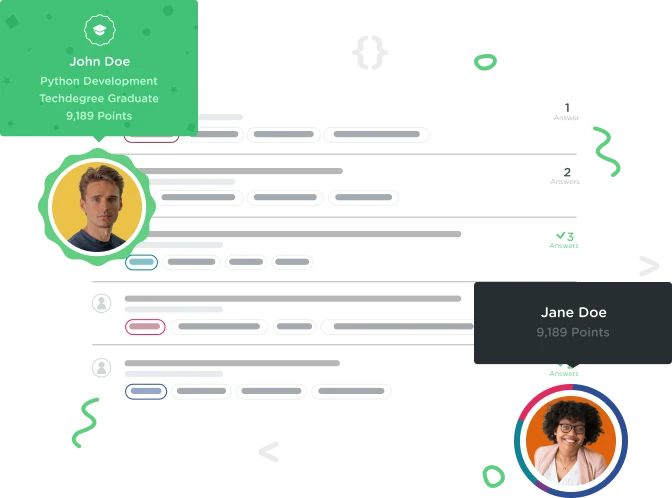
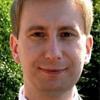
Tomasz Necek
Courses Plus Student 7,028 PointsText from the user into .txt file. Here is my code....
Hi! I'm trying to save what the user say for the "How are you" question into .txt file + date. The programme creates .txt file but empty. Can you help ?
import java.util.Scanner;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.io.FileNotFoundException;
import java.io.PrintWriter;
public class notes
{
public static void main(String[] args) throws FileNotFoundException
{
Scanner in = new Scanner(System.in);
System.out.print("How are you? ");
String answer = in.nextLine();
System.out.print(answer);
String timeStamp = new SimpleDateFormat("yyyyMMdd_HHmmss").format(Calendar.getInstance().getTime());
System.out.print(timeStamp);
String nazwaPliku = "d:\\javunia.txt";
PrintWriter out = new PrintWriter(nazwaPliku);
in.nextLine();
out.close();
}
}
1 Answer
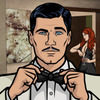
Jakob Wozniak
17,896 PointsI might be a noob, but here's how I'd go about this:
1) I would use a BufferedReader instead of a Scanner. I've never used a Scanner, so I don't know how it works. I'd declare a buffered reader in the fields section. "private BufferedReader mReader = new BufferedReader(new InputStreamReader(System.in))"
2) I would try to separate your methods/functions a little more: i.e., have a method that asks the user how they're doing and assigns their answer to a variable, have a method that adds the date to the variable, have a method that saves the variable to the text file.
3) I don't know the method you're using to write/save the text. The one I've used looks like this:
public void exportTo(String filename){
try (
FileOutputStream fos = new FileOutputStream(filename);
PrintWriter writer = new PrintWriter(fos);
) {
writer.printf("%s | %s", yourFileForTheInput, yourFileForTheDate);
} catch (IOException ioe) {
System.out.printf("Problem saving %s %n", filename);
ioe.printStackTrace();
}
}
4) Apart from that, I'd try taking the Java course on building a Karaoke Machine. It covers saving, input, and all kinds of stuff.
I hope this helps! Good luck!