Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial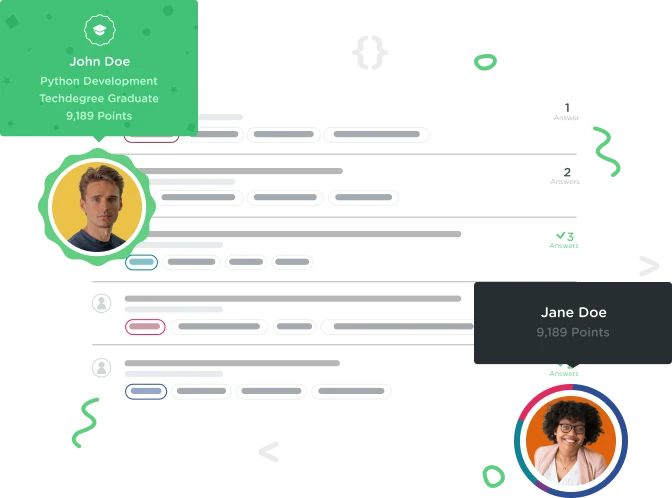
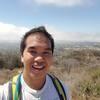
Ben Junya
12,365 PointsText label is blank when you press the button
Hey Everyone!
I'm having a problem when I press the button, the text just disappears from the iOS Simulator, and honestly, I don't know what's going on or what to do. Can someone give me a hand? Here's my code:
PViewController.h
//
// PMViewController.h
// CrystalBall
//
// Created by Ben Junya on 7/16/14.
// Copyright (c) 2014 Prism-Mobile. All rights reserved.
//
#import <UIKit/UIKit.h>
@class PMCrystalBall;
@interface PMViewController : UIViewController
@property (strong, nonatomic) IBOutlet UILabel *predictionLabel;
@property (strong, nonatomic) PMCrystalBall *crystalBall;
- (IBAction)buttonPressed;
@end
PMViewController.m
//
// PMViewController.m
// CrystalBall
//
// Created by Ben Junya on 7/16/14.
// Copyright (c) 2014 Prism-Mobile. All rights reserved.
//
#import "PMViewController.h"
#import "PMCrystalBall.h"
@interface PMViewController ()
@end
@implementation PMViewController
- (void)viewDidLoad
{
[super viewDidLoad];
self.crystalBall = [[PMCrystalBall alloc] init];
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
- (IBAction)buttonPressed {
self.predictionLabel.text = [self.crystalBall randomPrediction];
}
@end
PMCrystalBall.h
//
// PMCrystalBall.h
// CrystalBall
//
// Created by Ben Junya on 7/18/14.
// Copyright (c) 2014 Prism-Mobile. All rights reserved.
//
#import <Foundation/Foundation.h>
@interface PMCrystalBall : NSObject {
NSArray *_predictions;
}
@property (strong, nonatomic, readonly) NSArray *predictions;
@property (strong, nonatomic) NSString *randomPrediction;
-(NSString*) randomPrediciton;
@end
PMCrystalBall.m
//
// PMCrystalBall.m
// CrystalBall
//
// Created by Ben Junya on 7/18/14.
// Copyright (c) 2014 Prism-Mobile. All rights reserved.
//
#import "PMCrystalBall.h"
@implementation PMCrystalBall
-(NSArray*) predictions {
if (_predictions == nil) {
_predictions = [[NSArray alloc] initWithObjects:
@"It is certain",
@"It is decidedly so",
@"All signs say yes",
@"The stars are not aligned",
@"My reply is no",
@"It is doubtful",
@"Better not tell you now",
@"Concentrate and ask again",
@"Unable to answer now", nil];
}
return _predictions;
}
-(NSString*) randomPrediciton {
int random = arc4random_uniform(self.predictions.count);
return [self.predictions objectAtIndex:random];
}
@end
3 Answers

Stone Preston
42,016 Pointslooks like you mispelled your randomPrediction method name in your crystal ball implementation file
-(NSString*) randomPrediciton {
int random = arc4random_uniform(self.predictions.count);
return [self.predictions objectAtIndex:random];
}
change it to
-(NSString*) randomPrediction {
int random = arc4random_uniform(self.predictions.count);
return [self.predictions objectAtIndex:random];
}
also change it it in your header file:
-(NSString*) randomPrediction;
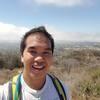
Ben Junya
12,365 PointsThis STILL is not solved. I haven't gotten any help... What's going on Treehouse?
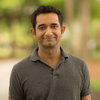
Amit Bijlani
Treehouse Guest TeacherYou might have a breakpoint set in your code. Try Command+Y
to disable all breakpoints and run your program.
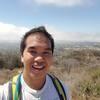
Ben Junya
12,365 PointsHey Amit Bijlani that unfortunately didn't work. Any other suggestions?
Do you need me to resubmit code?

Mrunal Kharod
10,657 PointsI am having the same issue, when 'predict' button is pressed the labels disappear from the view, though the code is exactly the same. has anyone came across the same issue? does anyone found the solution?
Ben Junya
12,365 PointsBen Junya
12,365 PointsHey Preston, Thanks for being quick with a response!
I'm still having the same problem though. The name is changed and all, but in the simulator, it still comes up as blank whenever I press the button :(
Ben Junya
12,365 PointsBen Junya
12,365 PointsHey Stone Preston - it's still causing problems. The text label is still blank. Do you know what's going on?