Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial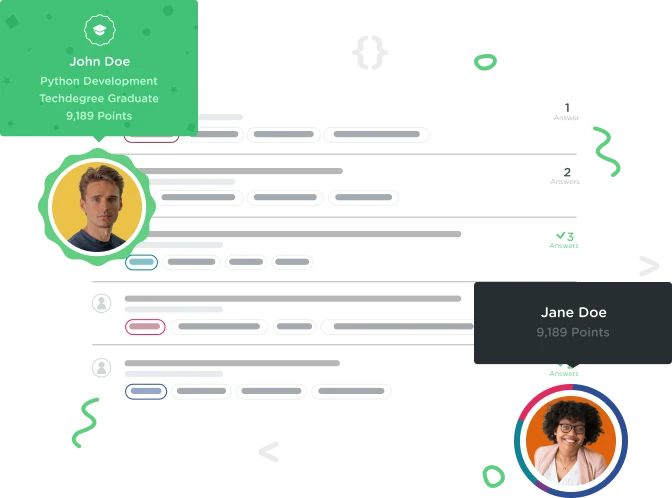

Dennis de Vries
9,440 PointsText-input not transferred after hitting Add-button: Todo-list problem
Hey there,
Hope to get some help with my code on the Todo list, as I am currently completely lost.
Problem is: when I add a new task, the text I put in is not transferred to the incomplete task list. So whatever I fill in, if I hit the 'add' button, It'll just add an empty listitem with a checkbox.
Here's my code:
//Problem: user interaction doesn't provide desired results
//Solution: add interactivity so the user can manage daily tasks
var taskInput = document.getElementById("new-task"); //new-task
var addButton = document.getElementsByTagName("button")[0]; //first button
var inCompleteTasksHolder = document.getElementById("incomplete-tasks"); //incomplete-task ul
var completedTasksHolder = document.getElementById("completed-tasks"); //completed-tasks ul
//New Task list item
var createNewTaskElement = function(taskString) {
var listItem = document.createElement("li");
//input (checkbox)
var checkbox = document.createElement("input");
//label
var label = document.createElement("label");
//input (text)
var edit = document.createElement("input");
//button.(edit)
var editButton = document.createElement("button");
//button.(delete)
var deleteButton = document.createElement("button");
//Each element needs modifying
checkbox.type = "checkbox";
edit.type = "text";
editButton.innertext = "Edit";
editButton.className = "edit";
deleteButton.innertext = "Delete";
deleteButton.className = "delete";
label.innertext = taskString;
//Each element needs appending
listItem.appendChild(checkbox);
listItem.appendChild(label);
listItem.appendChild(edit);
listItem.appendChild(editButton);
listItem.appendChild(deleteButton);
return listItem;
};
//Add a new task
var addTask = function () {
console.log("Add task...");
//When button is pressed
//Create new list item with the text from the #new-task:
var listItem = createNewTaskElement(taskInput.value);
//Append listItem to inCompleteTaskHolder
inCompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
}
//Edit an existing task
var editTask = function () {
console.log("Edit task...");
//When the Edit button is pressed
//if the class of the parent is .editMode
//Switch from .editMode
//label text become input's value
//else
//Switch to .editMode
//input value becomes label's text
//toggle .editMode on the parent
}
//Mark a task as complete
var taskCompleted = function() {
console.log("Complete task...");
//append the task item to the #completed-tasks
var listItem = this.parentNode;
completedTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskIncomplete);
}
//Mark task as incomplete
var taskIncomplete = function() {
console.log("Incomplete task...");
//append the task item to the #incomplete-tasks
var listItem = this.parentNode;
inCompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
}
//Delete an existing task
var deleteTask = function() {
console.log("Delete task...");
var listItem = this.parentNode;
var ul = listItem.parentNode;
//remove parent list item fomr ul
ul.removeChild(listItem);
}
var bindTaskEvents = function(taskListItem, checkboxEventHandler) {
console.log("Bind list item events");
//select taskListItem children
var checkbox = taskListItem.querySelector("input[type=checkbox]");
var editButton = taskListItem.querySelector("button.edit");
var deleteButton = taskListItem.querySelector("button.delete");
//bind editTask to edit button
editButton.onclick = editTask;
//bind deleteTask to deletebutton
deleteButton.onclick = deleteTask;
//bind checkboxEventHandler to the checkbox
checkbox.onchange = checkboxEventHandler;
};
//Set the click handler to the addTask function
addButton.onclick = addTask;
//cycle over incompleteTaskHolder ul list items
for (var i = 0; i< inCompleteTasksHolder.children.length; i++) {
//bind events to list items children (taskcompleted)
bindTaskEvents(inCompleteTasksHolder.children[i], taskCompleted);
};
//cycle over completedTaskHolder ul list items
for (var i = 0; i< completedTasksHolder.children.length; i++) {
//bind events to list items children (taskincompleted)
bindTaskEvents(completedTasksHolder.children[i], taskIncomplete);
};
There's no error messages in the console, so that isn't helpfull. There might be a type somewhere but I've checked, re-checked and re-re-re-rechecked and now I'm seeing stars.
Any help would be appreciated, thanks!
Dennis
5 Answers
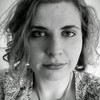
Grace Kelly
33,990 PointsHi Dennis, in your code you are writing innerText incorrectly ("innertext"). You need to use camel case when using innerText, like so:
//Each element needs modifying
checkbox.type = "checkbox";
edit.type = "text";
editButton.innerText = "Edit";
editButton.className = "edit";
deleteButton.innerText = "Delete";
deleteButton.className = "delete";
label.innerText = taskString;
Hope that helps!!
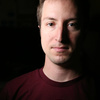
Yohanan Braun-Feder
6,101 Pointsi'm having the same issue but I am using camel case... what do you think might be the problem?
// Problem: User Interaction doesnt provide desired results.
// Solution: Add interactivity so the user can manage daily tasks.
var taskInput = document.getElementById("new-task"); //new-task
var addButton = document.getElementsByTagName("button")[0]; // first button
var incompleteTasksHolder = document.getElementById("incomplete-tasks");
var completedTasksHolder = document.getElementById("completed-tasks");
//New Task Li
var createNewTaskElement = function(taskString) {
// create list item
var listItem = document.createElement("li");
//input (checkbox)
var checkBox = document.createElement("input"); //checkbox
//label
var label = document.createElement("label");
//input (text)
var editInput = document.createElement("input"); //text
//button.edit
var editButton = document.createElement("button");
//button.delete
var deleteButton = document.createElement("button");
//Each element needs modifying and appending
checkBox.type = "checkbox";
editInput.type = "text";
editButton.innerText = "Edit";
editButton.className = "edit";
deleteButton.innerText = "Delete";
deleteButton.className = "delete";
label.innerText = taskString;
listItem.appendChild(checkBox);
listItem.appendChild(label);
listItem.appendChild(editInput);
listItem.appendChild(editButton);
listItem.appendChild(deleteButton);
return listItem
}
// add a new task
var addTask = function () {
console.log("add task...");
//create a new list item with the text from #new-task
var listItem = createNewTaskElement(taskInput.value);
// append li to incompleteTasksHolder
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
}
// edit existing task
var editTask = function () {
console.log("edit task...");
//when the edit button is pressed
//if the class of the parent is .editMode
//switch from .editMode
//label text become the input's value
//else
//switch to .editMode
//input value becomes the label's text
}
// delete existing task
var deleteTask = function () {
console.log("delete task...");
var listItem = this.parentNode;
var ul =listItem.parentNode;
//remove the parent li from the ul
ul.removeChild(listItem);
}
//mark task as complete
var taskCompleted = function () {
console.log("task Complete...");
//append the task li to the #completed-tasks
var listItem = this.parentNode;
completedTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskIncomplete);
}
//mark task as incomplete
var taskIncomplete = function () {
console.log("task Incomplete...");
//append the task li to the #incomplete-tasks
var listItem = this.parentNode;
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
}
var bindTaskEvents = function (taskListItem, checkBoxEventHandler) {
console.log("bind li events");
//select taskListItem's children
var checkBox = taskListItem.querySelector("input[type=checkbox]");
var editButton = taskListItem.querySelector("button.edit");
var deleteButton = taskListItem.querySelector("button.delete");
//bind editTask to edit button
editButton.onclick = editTask;
//bind deleteTask to delete button
deleteButton.onclick = deleteTask;
//bind checkBoxEventHandler the checkbox
checkBox.onchange = checkBoxEventHandler;
}
//set the click handler to the addtask function
addButton.onclick = addTask;
//cycle over incompleteTasksHolder ul list items
for ( i = 0; i < incompleteTasksHolder.children.length; i++ ){
//bind events to list item's children (taskCompleted)
bindTaskEvents(incompleteTasksHolder.children[i], taskCompleted);
}
//cycle over completedTasksHolder ul list items
for ( i = 0; i < completedTasksHolder.children.length; i++ ){
//bind events to list item's children (taskInomplete)
bindTaskEvents(completedTasksHolder.children[i], taskIncomplete);
}
console doesn't return any errors or report any bugs. when i finish typing in a new task and the input text box loses focus, the text becomes grey and the task created has an empty label.
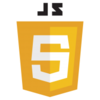
Ygor Fonseca
6,929 PointsI have this problem using firefox, in chrome it work pretty well!
Must be a bug in firefox, I don't know...

Matthew Carr
11,220 PointsIn Firefox, switch to using .innerHTML(); instead of .innerText();

rthvrxuktt
15,680 PointsYep, I had that problem here too on Firefox.
Dennis de Vries
9,440 PointsDennis de Vries
9,440 PointsUuuugh, I was afraid it would be something silly like that. Thanks so much for spotting it so quickly, Grace!
I'll go cry in corner now. ;)
Grace Kelly
33,990 PointsGrace Kelly
33,990 Pointshaha no worries Dennis :D these things happen to me all the time!!
james flynn
3,618 Pointsjames flynn
3,618 PointsThanks! I was missing the <code>label.innerText = taskString;</code>, as well!