Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial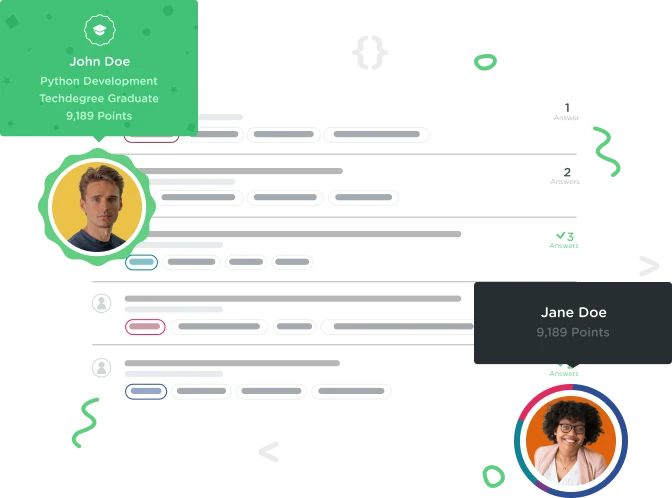
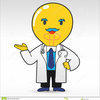
Cameron Hastings
3,439 PointsTHE ABSOLUTE WORST! (only 1 out of 900 can fix this problem)... please help.
I'm on the last task. It has to do with the Main class. I left the original TODO comments in.
I've been at this for too long. Rewatching the video didn't help. Not sure what to do... please help.
public class Forum {
private String topic;
public Forum(String topic) {
this.topic = new topic();
}
public String getTopic() {
return topic;
}
public void addPost(ForumPost post) {
System.out.printf("A new post in %s topic from %s %s about %s is available",
topic,
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle()
);
}
}
public class User {
private String firstName;
private String lastName;
public User(String firstName, String lastName) {
this.firstName = new firstName();
this.lastName = new lastName();
}
public String getFirstName() {
return firstName;
}
public String getLastName() {
return lastName;
}
}
public class ForumPost {
private User author;
private String title;
private String description;
public ForumPost (User author, String title, String description) {
this.author = new author();
this.title = new title();
this.description = new description();
}
public User getAuthor() {
return author;
}
public String getTitle() {
return title;
}
public String getDescription() {
return description;
}
}
public class Main {
public static void main(String[] args) {
System.out.println("Beginning forum example");
if (args.length < 2) {
System.out.println("Usage: java Main <first name> <last name>");
System.err.println("<first name> and <last name> are required");
System.exit(1);
}
Forum forum = new Forum("Java");
// TODO: pass in the first name and last name that are in the args parameter
User author = new User("ASDFASDFASF!!", "WTF!");
// TODO: initialize the forum post with the user created above and a title and description of your choice
ForumPost post = new ForumPost(author, "beenatthisfor", "onepointfivehours");
forum.addPost(post);
}
}
1 Answer

Seth Kroger
56,413 PointsIf you take a look at this bit at the top of main():
if (args.length < 2) {
System.out.println("Usage: java Main <first name> <last name>");
System.err.println("<first name> and <last name> are required");
System.exit(1);
}
We're finally using that String array called args that main() always has as a parameter. It requires 2 command line arguments when it's started from console/terminal, a first name and a last name. When you create a User:
// TODO: pass in the first name and last name that are in the args parameter
User author = new User("ASDFASDFASF!!", "WTF!");
Notice that the TODO asks you to use the values passed in through args.
Cameron Hastings
3,439 PointsCameron Hastings
3,439 PointsThank you for your help.
With what you said, I have tried all of these:
User author = new User(String[0] args, String[1] args);
User author = new User(args[0], args[1]); <---- thought this would do it, after seeing many examples of it being used.
User author = new User(String[0], String[1]); <---- nope.
User author = new User(args.firstName(), args.lastName()); <---- nope.
User author = new User(args(0), args(1)); <---- (╯°□°)╯︵ ┻━┻
User author = new User(String(0), String(1)); <---- ┬─┬ ノ( ゜-゜ノ)
At this point, I'm not sure what else to do. Am I even close?
I have wasted half of my Wednesday trying to figure this out...
Seth Kroger
56,413 PointsSeth Kroger
56,413 PointsOK, I see there are other issues with your User, Forum and ForumPost constructors. You had uncommented the part about addPost() as if you were on the last step but there is no way to pass the first step with what you wrote. My bad for glancing and skimming. If you change it to this:
and likewise with Forum and ForumPost, then new User(args[0], args[1]) should work.
Seth Kroger
56,413 PointsSeth Kroger
56,413 PointsOK, let me amend that again. After checking your code, the challenge actually accepts what you wrote but it should not. Running that it through javac gives a compiler error for each
this.topic = new topic();
, etc. it encounters because topic is a variable not a class and doesn't need to be created since it is passed in.Cameron Hastings
3,439 PointsCameron Hastings
3,439 PointsThank you!