Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial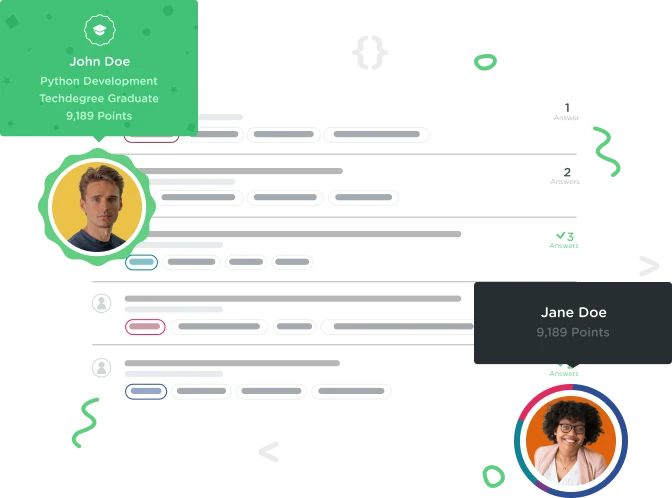
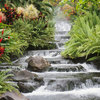
kabir k
Courses Plus Student 18,036 PointsThe argument of the to_s method
Given the Contact class below, what is 'full_name' in the argument of the to_s method? Is it a method? And why is it in quotes?
class Contact
attr_writer :first_name, :middle_name, :last_name
def first_name
@first_name
end
def middle_name
@middle_name
end
def last_name
@last_name
end
def first_last
first_name + " " + last_name
end
def last_first
last_first = last_name
last_first += ", "
last_first += first_name
if !@middle_name.nil?
last_first += " "
last_first += middle_name.slice(0, 1)
last_first += "."
end
last_first
end
def full_name
full_name = first_name
if !@middle_name.nil?
full_name += " "
full_name += middle_name
end
full_name += ' '
full_name += last_name
full_name
end
def to_s(format = 'full_name')
case format
when 'full_name'
full_name
when 'last_first'
last_first
when 'first'
first_name
when 'last'
last_name
else
first_last
end
end
end
jason = Contact.new
jason.first_name = "Jason"
jason.last_name = "Seifer"
puts jason.to_s
puts jason.to_s('full_name')
puts jason.to_s('last_first')
nick = Contact.new
nick.first_name = "Nick"
nick.middle_name = "A"
nick.last_name = "Pettit"
puts nick.to_s('first_last')
2 Answers

Ryan Duchene
Courses Plus Student 46,022 PointsIn that particular method, 'full_name'
is being used as sort of a keyword. The to_s
method will accept 'full_name'
(default), 'last_first'
, 'first'
, or 'last'
and call the corresponding method, and if anything else is passed in, it will default to the first_last
.

Unsubscribed User
11,042 Pointseverything between quotes is a string! :-)
kabir k
Courses Plus Student 18,036 Pointskabir k
Courses Plus Student 18,036 PointsYeah, it's written like you would a method or variable name, yet it's in quotes. That's what I find a bit confusing.
Thanks, by the way.
Ryan Duchene
Courses Plus Student 46,022 PointsRyan Duchene
Courses Plus Student 46,022 PointsSure thing. Glad I could help!