Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial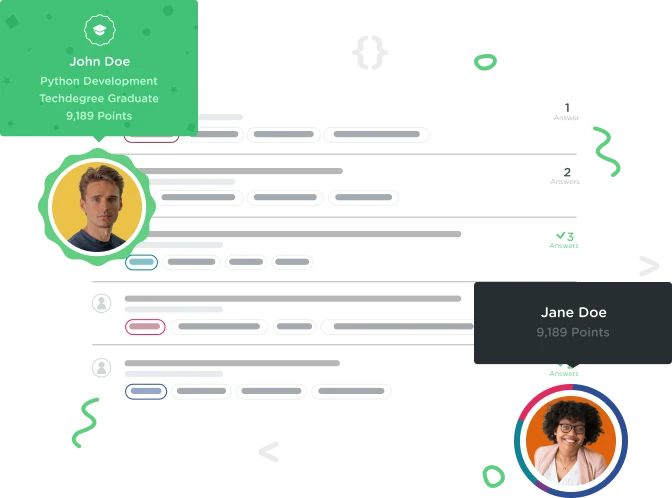
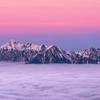
isaacrichardson
14,313 PointsThe attributes 'agile' and 'name' are giving me errors
When I try to access the 'name' attribute on the Thief class it gives me a AttributeError. This is the code from the play.py file:
from thieves import Thief
isaac = Thief("Isaac", sneaky=False)
print(isaac.name)
print(isaac.sneaky)
print(isaac.agile)
print(isaac.hide(8))
I have no idea why, I've doubled checked all the code and it matches one for one to the teachers code.
Also when I print the agile attribute it prints 'Isaac', the name value. Does anybody have any idea why or what is causing this?
This is the rest of the code
import random
from attributes import Agile, Sneaky
from character import Character
class Thief(Agile, Sneaky, Character):
def pickpocket(self):
return self.sneaky and bool(random.randint(0,1))
import random
class Sneaky:
sneaky = True
def __init__(self, sneaky=True):
super().__init__(*args, **kwargs)
self.sneaky = sneaky
def hide(self, light_level):
return self.sneaky and light_level < 10
class Agile:
agile = True
def __init__(self, agile=True, *args, **kwargs):
super().__init__(*args, **kwargs)
self.agile = agile
def evade(self):
return self.agile and random.randint(0, 1)
class Character:
def __init__(self, name="", **kwargs):
if not name:
raise ValueError("'name' is required")
self.name = name
for key, value in kwargs.items():
setattr(self, key, value)
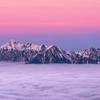
isaacrichardson
14,313 PointsI'm not using Workspaces I'm using VScode.

KRIS NIKOLAISEN
54,967 PointsYou could create a workspace and drag your files to the lower left corner to import.

adrian miranda
13,561 PointsAt the very least, upload the contents of thieves.py
1 Answer
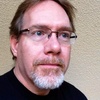
Chris Freeman
Treehouse Moderator 68,423 PointsThe critical factor is a positional argument will be assigned to the first available parameter regardless if it is a positional or keyword parameter.
Given
isaac = Thief("Isaac", sneaky=False)
The first parameters seen are from Agile.__init__
:
(agile=True, *args **kwargs)
Here, βIsaacβ is assigned to agile
and sneaky=False
is added you the **kwargs
dictionary which is passed to Sneaky
The next parameters are from Sneaky.__init__
:
(self, sneaky=True)
Here, the sneaky
keyword argument matches the sneaky
parameter, so it is assigned. This concludes argument to parameter matching.
Note: Sneaky.__init__
does not have an *args
or **kwargs
parameter so no arguments will ever be passed to Character
via the super()
statement.
Post back if you need more help. Good luck!!!
KRIS NIKOLAISEN
54,967 PointsKRIS NIKOLAISEN
54,967 PointsCan you post a snapshot? Click the camera icon in the upper right corner, then 'Take Snapshot', then post the link created here.