Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial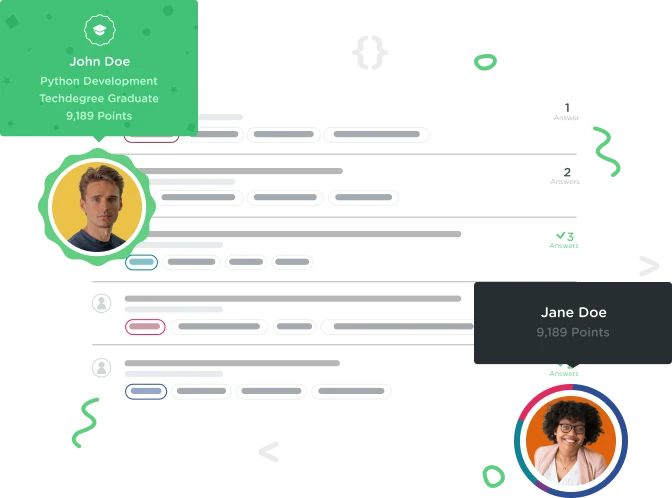
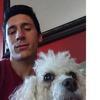
Kevin Grifo
9,824 PointsThe background color and text keeps on getting refreshed to the default values and not the saved values.
When I click the show another fact button a couple of times and then change the orientation of my device, the background color, and fact text continue to change to the default values of green and the ant fact. I'm using a newer version of Android studio, so I believe this may be the problem. I'm assuming that the onRestoreInstanceState and onSavedInstanceState methods are implemented differently or maybe it is the type of device i'm using as well. Any help would truly be appreciated. Please view my FunFactsActivity code below which I downloaded from the github link in this course.
package com.teamtreehouse.funfacts;
import android.app.Activity; import android.graphics.Color; import android.os.Bundle; import android.util.Log; import android.view.View; import android.widget.Button; import android.widget.RelativeLayout; import android.widget.TextView;
public class FunFactsActivity extends Activity {
private TextView mFactLabel;
private Button mShowFactButton;
private RelativeLayout mRelativeLayout;
private static final String KEY_FACT = "KEY_FACT";
private static final String KEY_COLOR = "KEY_COLOR";
private FactBook mFactBook = new FactBook();
private ColorWheel mColorWheel = new ColorWheel();
private String mFact = mFactBook.mFacts[0];
private int mColor = Color.parseColor(mColorWheel.mColors[8]);
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_facts);
// Assign the views from the layout file to the corresponding variables
mFactLabel = (TextView) findViewById(R.id.factTextView);
mShowFactButton = (Button) findViewById(R.id.showFactButton);
mRelativeLayout = (RelativeLayout) findViewById(R.id.relativeLayout);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View v) {
String mFact = mFactBook.getFact();
//update the screen with our new fact.
mFactLabel.setText(mFact);
int mColor = mColorWheel.getColor();
mRelativeLayout.setBackgroundColor(mColor);
mShowFactButton.setTextColor(mColor);
}
};
mShowFactButton.setOnClickListener(listener);
//Toast.makeText(this, "Yay! Our Activity was created", Toast.LENGTH_SHORT).show();
//Log.d(TAG, "We're logging from the onCreate() method!");
}
@Override
protected void onSaveInstanceState(Bundle outState) {
super.onSaveInstanceState(outState);
outState.putString(KEY_FACT, mFact);
outState.putInt(KEY_COLOR, mColor);
}
@Override
protected void onRestoreInstanceState(Bundle savedInstanceState) {
super.onRestoreInstanceState(savedInstanceState);
mFact = savedInstanceState.getString(KEY_FACT);
mFactLabel.setText(mFact);
mColor = savedInstanceState.getInt(KEY_COLOR);
mRelativeLayout.setBackgroundColor(mColor);
mShowFactButton.setTextColor(mColor);
}
}
Thank you in advance.
1 Answer

Seth Kroger
56,414 PointsI want to draw your attention to something in the OnClickListener:
@Override
public void onClick(View v) {
String mFact = mFactBook.getFact(); // <- you are declaring a new string variable here
//update the screen with our new fact.
mFactLabel.setText(mFact);
int mColor = mColorWheel.getColor(); // <- you are declaring a new int variable here
mRelativeLayout.setBackgroundColor(mColor);
mShowFactButton.setTextColor(mColor);
}
Both these new variables are only local to onClick() and are not the member variables of the same names, so their values aren't stored.
Kevin Grifo
9,824 PointsKevin Grifo
9,824 PointsI can't believe I didn't catch that. That was my problem, thank you kind sir.