Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial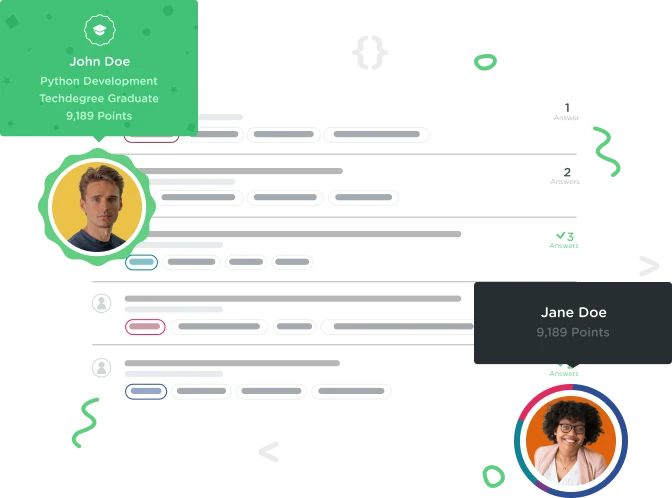

Raveesh Agarwal
2,994 PointsThe Beauty Of Kotlin!!
Hi! Just wanted to share this over here. I followed the course in Kotlin and translated the whole app. By inherent simplicity, I can't help but admire how expressive my code is, and also, how less the number of lines in the code it already is. I will post my app here, I have used a recyclerVIew for this project! Please let me know if posts that are not questions should go somewhere else.
Hole.kt
package `in`.cookytech.golfy
val mHoles = Array<Hole>(18,{ Hole("Hole ${it+1} :", 0) })
data class Hole(
val mHoleLabel:String,
var mHoleStrokeCount: Int)
HolesAdapter.kt
package `in`.cookytech.golfy
import android.support.v7.widget.RecyclerView
import android.view.View
import android.view.ViewGroup
import kotlinx.android.synthetic.main.item.view.*
import org.jetbrains.anko.layoutInflater
import org.jetbrains.anko.sdk25.coroutines.onClick
import org.jetbrains.anko.toast
class HolesAdapter : RecyclerView.Adapter<HolesAdapter.HolesViewHolder>() {
override fun onBindViewHolder(holder: HolesViewHolder, position: Int) {
holder.mPlusButton.onClick {
mHoles[position].mHoleStrokeCount++
notifyDataSetChanged()
}
holder.mMinusButton.onClick {
if (mHoles[position].mHoleStrokeCount > 0) {
mHoles[position].mHoleStrokeCount--
notifyDataSetChanged()
}
else{
holder.mCtx.toast("Can't put negative holes!!")
}
}
holder.mHoleLabel.text = mHoles[position].mHoleLabel
holder.mHoleCount.text = mHoles[position].mHoleStrokeCount.toString()
}
override fun getItemCount(): Int = mHoles.size
override fun onCreateViewHolder(parent: ViewGroup, viewType: Int): HolesViewHolder {
val v = parent
.context
.layoutInflater
.inflate(R.layout.item, parent, false )
return HolesViewHolder(v)
}
class HolesViewHolder(itemView: View) : RecyclerView.ViewHolder(itemView){
val mHoleLabel = itemView.itemHoleNumber!!
val mHoleCount = itemView.itemHoleCount!!
val mPlusButton = itemView.itemPlusButton!!
val mMinusButton = itemView.itemMinusButton!!
val mCtx = itemView.context!!
}
}
I have included the context in the ViewHolder so that I can toast in the onClick subroutiene.
MainActivity.kt
package `in`.cookytech.golfy
import android.annotation.SuppressLint
import android.content.Context
import android.content.SharedPreferences
import android.os.Bundle
import android.support.v7.app.AppCompatActivity
import android.support.v7.widget.LinearLayoutManager
import android.view.Menu
import android.view.MenuItem
import kotlinx.android.synthetic.main.activity_main.*
class MainActivity : AppCompatActivity() {
companion object {
val PREFS_FILE = "in.cookytech.golfy.preferences"
val KEY_STROKECOUNT = "key_strokecount"
}
private lateinit var mSharedPreferences : SharedPreferences
private lateinit var mEditor: SharedPreferences.Editor
@SuppressLint("CommitPrefEdits")
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
mSharedPreferences = getSharedPreferences(PREFS_FILE, Context.MODE_PRIVATE)
mEditor = mSharedPreferences.edit()
for (i in 0 until mHoles.size){
mHoles[i].mHoleStrokeCount = mSharedPreferences.getInt(KEY_STROKECOUNT + i, 0)
}
holesRecyclerView.adapter = HolesAdapter()
holesRecyclerView.layoutManager = LinearLayoutManager(this)
}
override fun onPause() {
super.onPause()
for (i in 0 until mHoles.size){
mEditor.putInt(KEY_STROKECOUNT + i, mHoles[i].mHoleStrokeCount)
}
mEditor.apply()
}
override fun onCreateOptionsMenu(menu: Menu): Boolean {
menuInflater.inflate(R.menu.menu_main,menu)
return true
}
override fun onOptionsItemSelected(item: MenuItem): Boolean {
val id = item.itemId
if (id == R.id.clearAllPreferences){
mEditor.clear()
mEditor.apply()
for (hole in mHoles){
hole.mHoleStrokeCount = 0
}
holesRecyclerView.adapter.notifyDataSetChanged()
}
return true
}
}
And surprisingly, that was all it took to get the job done!
Ralph Emerson Manzano
1,370 PointsRalph Emerson Manzano
1,370 Pointsthis would even be more simplified if you used android's data binding library, great work though, will be exploring Kotlin more very soon!