Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial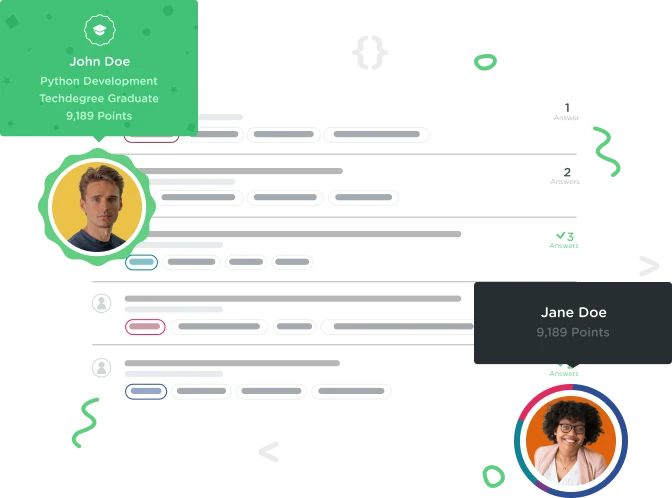

Peter Gess
16,553 PointsThe best way to render data from an API call to a front-end webpage using pug templating with Express and Node
I am able to console out the API call but am not sure how to get the data from the API call onto a Pug Templating engine. Could also do straight HTML if that is easier too. Please advise!
app.js:
const bitcoin = require('./bitcoin.js')
const express = require('express')
const app = express()
app.set('view engine', 'pug')
const mainRoutes = require('./routes')
const routes = require('./routes/index')
app.use(routes)
app.get('/', (request, response) => {
res.send('index')
})
app.listen(3000)
// Display price
const currentPrice = process.argv.slice(2).join("_").replace(' ', '_')
// Pull in module
bitcoin.get(currentPrice)
index.js
const express = require('express')
const router = express.Router()
router.get('/', (req, res) => {
res.render('index', function (err, printBitcoin) {
res.send(printBitcoin)
})
})
module.exports = router
bitcoin.js
const https = require('https');
//Print bitcoin price
function printBitcoin (data) {
const message = `The current price of Bitcoin is $${data}`
console.log(message);
};
// API Call
function get(currentPrice) {
const request = https.get(`https://api.coindesk.com/v1/bpi/currentprice/USD.json`, (res) => {
res.on('data', (d) => {
const data = JSON.parse(d)
printBitcoin(data.bpi.USD.rate);
});
}).on('error', (e) => {
console.error(e);
});
};
// Export to app.js
module.exports.get = get