Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial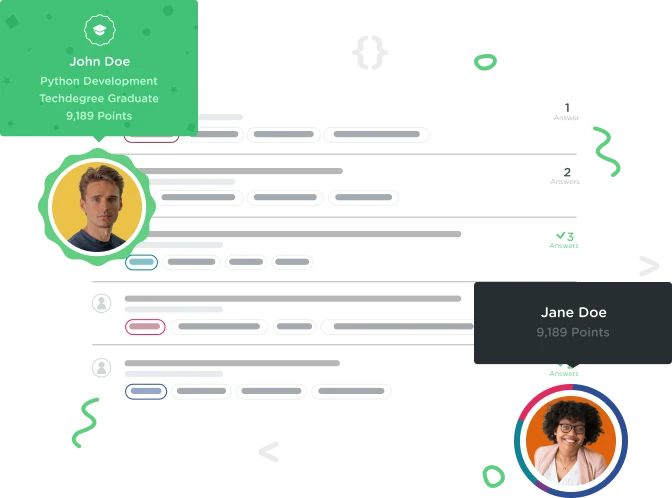

Enrique Mendoza
2,820 PointsThe Bummer! Looks like you didn't present the TreeStory (3), doesn't make sense. I did present it. Could you explain me?
Presenting TreeStory (3) is covered in Main.java line 33 and Prompter.java line 60.
Honestly, I am not sure if I understand the content of that error message or the task I am supposed to do.
package com.teamtreehouse;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.Arrays;
import java.util.List;
public class Main {
public static void main(String[] args) {
Prompter prompter = new Prompter();
try {
prompter.promptStory();
} catch (IOException e) {
e.printStackTrace();
}
String finalString = prompter.run();
prompter.presentStory(finalString);
}
}
package com.teamtreehouse;
import java.io.*;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
public class Prompter {
private BufferedReader mReader;
private Set<String> mCensoredWords;
private String mStory;
public Prompter() {
mReader = new BufferedReader(new InputStreamReader(System.in));
loadCensoredWords();
mStory = "";
}
public void promptStory() throws IOException {
System.out.println("Write an story template: ");
mStory = mReader.readLine();
}
private void loadCensoredWords() {
mCensoredWords = new HashSet<String>();
Path file = Paths.get("resources", "censored_words.txt");
List<String> words = null;
try {
words = Files.readAllLines(file);
} catch (IOException e) {
System.out.println("Couldn't load censored words");
e.printStackTrace();
}
mCensoredWords.addAll(words);
}
public String run() {
Template tmpl = new Template(mStory);
//System.out.println("Write a word for each placeholder: ");
List<String> results = null;
try {
results = promptForWords(tmpl);
} catch (IOException e) {
System.out.println("There was a problem prompting for words");
e.printStackTrace();
System.exit(0);
}
return tmpl.render(results);
}
public void presentStory(String finalString) {
System.out.printf("%n%nThe TreeStory is:%n%n%s", finalString);
}
/**
* Prompts user for each of the blanks
*
* @param tmpl The compiled template
* @return
* @throws IOException
*/
public List<String> promptForWords(Template tmpl) throws IOException {
List<String> words = new ArrayList<String>();
for (String phrase : tmpl.getPlaceHolders()) {
String word = promptForWord(phrase);
words.add(word);
}
return words;
}
/**
* Prompts the user for the answer to the fill in the blank. Value is guaranteed to be not in the censored words list.
*
* @param phrase The word that the user should be prompted. eg: adjective, proper noun, name
* @return What the user responded
*/
public String promptForWord(String phrase) throws IOException {
String answer;
String toCheck;
boolean itIsAGoodWord;
do {
itIsAGoodWord = true;
System.out.printf("Write a word for the placeholder __%s__: ",phrase);
answer = mReader.readLine().toLowerCase().trim();
toCheck = answer.replaceAll("\\s","");
for(String badWord : mCensoredWords) {
if (toCheck.contains(badWord)) {
itIsAGoodWord = false;
}
}
/*
if (itIsAGoodWord == false) {
System.out.printf("We are not allowed to use the word %s for a placeholder.%nTry again.%n",answer);
}*/
} while (!itIsAGoodWord);
return answer;
}
}
# This is essentially what I am testing
1. The user is prompted for a new string template (the one with the double underscores in it).
a. The prompter class has a new method that prompts for the story template, and that method is called.
2. The user is then prompted for each word that has been double underscored.
a. The answer is checked to see if it is contained in the censored words.
User is continually prompted until they enter a valid word
3. The user is presented with the completed story
1 Answer

Simon Coates
28,694 PointsThe following seems to work. I was going to replace your code bit by bit with that of a user who was able to complete the challenge (https://teamtreehouse.com/community/finishing-treestory-maybe-i-have-the-wrong-idea-of-implementation), but the first method i copied (promptForWord) made the problem go away. weird but it spared me a fun game of find the glitch.
package com.teamtreehouse;
import java.io.*;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
public class Prompter {
private BufferedReader mReader;
private Set<String> mCensoredWords;
private String mStory;
public Prompter() {
mReader = new BufferedReader(new InputStreamReader(System.in));
loadCensoredWords();
mStory = "";
}
public void promptStory() throws IOException {
System.out.println("Write an story template: ");
mStory = mReader.readLine();
}
private void loadCensoredWords() {
mCensoredWords = new HashSet<String>();
Path file = Paths.get("resources", "censored_words.txt");
List<String> words = null;
try {
words = Files.readAllLines(file);
} catch (IOException e) {
System.out.println("Couldn't load censored words");
e.printStackTrace();
}
mCensoredWords.addAll(words);
}
public String run() {
Template tmpl = new Template(mStory);
//System.out.println("Write a word for each placeholder: ");
List<String> results = null;
try {
results = promptForWords(tmpl);
} catch (IOException e) {
System.out.println("There was a problem prompting for words");
e.printStackTrace();
System.exit(0);
}
return tmpl.render(results);
}
public void presentStory(String finalString) {
System.out.printf("%n%nThe TreeStory is:%n%n%s", finalString);
}
/**
* Prompts user for each of the blanks
*
* @param tmpl The compiled template
* @return
* @throws IOException
*/
public List<String> promptForWords(Template tmpl) throws IOException {
List<String> words = new ArrayList<String>();
for (String phrase : tmpl.getPlaceHolders()) {
String word = promptForWord(phrase);
words.add(word);
}
return words;
}
public String promptForWord(String phrase) throws IOException {
System.out.printf("Write a word for the placeholder __%s__: ",phrase);
String word = mReader.readLine();
while (mCensoredWords.contains(word)) {
System.out.printf("That word is censored. Please enter another placeholder: ");
word = mReader.readLine();
}
return word;
}
}
A lot of people have been beating their heads against this particular brick wall. I think the verification for this task is very specific (and possibly based on output). If you get stuck, i think there is a way to use tagging to get treehouse to notify craig dennis. He's pretty good at rescuing his students.
Simon Coates
28,694 PointsSimon Coates
28,694 Pointsyeah, I copied the screen output from successful code. I put each line inside a println in main method. I was able to pass the challenge. So verification is string comparison. Anyhow, i did notice that your output ended with
whereas the correct code ended with:
so it's probably that you returned the lower case word, rather than using it for testing and returning the entered word in its original case.
Enrique Mendoza
2,820 PointsEnrique Mendoza
2,820 PointsThank you so much, It worked. I was beating my head with this wall.