Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial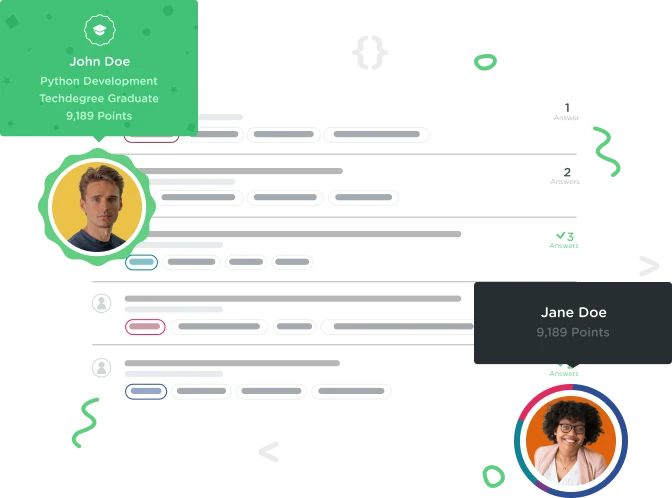

Ganta Hemanth
6,460 Pointsthe camera button not working
When camera button is pressed it should display a list of items. But for some reason it is not displaying.. Here is the code
package hemanth.example.com.connect;
import java.io.File;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.Locale;
import android.app.AlertDialog;
import android.content.DialogInterface;
import android.content.Intent;
import android.net.Uri;
import android.os.Environment;
import android.provider.MediaStore;
import android.support.v4.app.FragmentActivity;
import android.support.v4.app.ListFragment;
import android.support.v7.app.ActionBarActivity;
import android.support.v7.app.ActionBar;
import android.support.v4.app.Fragment;
import android.support.v4.app.FragmentManager;
import android.support.v4.app.FragmentTransaction;
import android.support.v4.app.FragmentPagerAdapter;
import android.os.Bundle;
import android.support.v4.view.ViewPager;
import android.util.Log;
import android.view.Gravity;
import android.view.LayoutInflater;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.view.ViewGroup;
import android.view.Window;
import android.widget.TextView;
import android.widget.Toast;
import com.parse.ParseAnalytics;
import com.parse.ParseUser;
public class MainActivity extends ActionBarActivity implements ActionBar.TabListener {
private Uri mUri;
/**
* The {@link android.support.v4.view.PagerAdapter} that will provide
* fragments for each of the sections. We use a
* {@link FragmentPagerAdapter} derivative, which will keep every
* loaded fragment in memory. If this becomes too memory intensive, it
may be best to switch to a
* {@link android.support.v4.app.FragmentStatePagerAdapter}.
*/
SectionsPagerAdapter mSectionsPagerAdapter;
protected DialogInterface.OnClickListener mlisitner= new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
switch (which){
case 0 :
Intent take_photo = new Intent(MediaStore.ACTION_IMAGE_CAPTURE);
mUri = getFileUri(4);
if(mUri==null)
{
Toast.makeText(MainActivity.this,"Check Your SD Card",Toast.LENGTH_LONG).show();
}
take_photo.putExtra(MediaStore.EXTRA_OUTPUT,mUri);
startActivityForResult(take_photo, 0);
break;
case 1:
break;
case 2:
break;
}
}
private Uri getFileUri(int mediaType) {
if(isExternalStorageAvailable()){
//get the external storage directory
File mediaStorage = new File(Environment.getExternalStoragePublicDirectory(Environment.DIRECTORY_PICTURES),
"Connect");
// create a sub directory for the app
if(!mediaStorage.exists()){
if(! mediaStorage.mkdirs()){
Log.e(MainActivity.class.getSimpleName(),"Failed To create directory");
return null;
}
}
// create file name
//create file
File mediaFile;
Date now = new Date();
String time = new SimpleDateFormat("yyyyMMdd_HHmmss",Locale.UK).format(now);
String path = mediaStorage.getPath() + File.separator;
if(mediaType == 4){
mediaFile = new File(path + "IMG_" + time + ".jpg");
}
else if(mediaType==5){
mediaFile = new File(path + "VID_"+time+".mp4");
}
else {
return null;
}
// return file uri
return Uri.fromFile(mediaFile);
}
else
{
return null;
}
}
private boolean isExternalStorageAvailable(){
String state = Environment.getExternalStorageState();
if(state.equals(Environment.MEDIA_MOUNTED)){
return true;
}
else{
return false;
}
}
};
/**
* The {@link ViewPager} that will host the section contents.
*/
ViewPager mViewPager;
public static final String TAG = MainActivity.class.getSimpleName();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ParseUser currentUser = ParseUser.getCurrentUser();
if (currentUser == null) {
navigateToLogin();
} else {
Log.i(TAG, currentUser.getUsername());
}
// Set up the action bar.
final ActionBar actionBar = getSupportActionBar();
actionBar.setNavigationMode(ActionBar.NAVIGATION_MODE_TABS);
// Create the adapter that will return a fragment for each of the three
// primary sections of the activity.
mSectionsPagerAdapter = new SectionsPagerAdapter(getSupportFragmentManager());
// Set up the ViewPager with the sections adapter.
mViewPager = (ViewPager) findViewById(R.id.pager);
mViewPager.setAdapter(mSectionsPagerAdapter);
// When swiping between different sections, select the corresponding
// tab. We can also use ActionBar.Tab#select() to do this if we have
// a reference to the Tab.
mViewPager.setOnPageChangeListener(new ViewPager.SimpleOnPageChangeListener() {
@Override
public void onPageSelected(int position) {
actionBar.setSelectedNavigationItem(position);
}
});
// For each of the sections in the app, add a tab to the action bar.
for (int i = 0; i < mSectionsPagerAdapter.getCount(); i++) {
// Create a tab with text corresponding to the page title defined by
// the adapter. Also specify this Activity object, which implements
// the TabListener interface, as the callback (listener) for when
// this tab is selected.
actionBar.addTab(
actionBar.newTab()
.setText(mSectionsPagerAdapter.getPageTitle(i))
.setTabListener(this));
}
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if(resultCode == RESULT_OK)
{
//IT IS SUCCESSFUL
//sending broadcast
Intent mediaScanIntent = new Intent(Intent.ACTION_MEDIA_SCANNER_SCAN_FILE);
mediaScanIntent.setData(mUri);
sendBroadcast(mediaScanIntent);
}
else if(resultCode==RESULT_CANCELED){
Toast.makeText(this,"Some error has popped",Toast.LENGTH_LONG).show();
}
}
private void navigateToLogin() {
Intent intent = new Intent(this, login_activity.class);
intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
intent.addFlags(Intent.FLAG_ACTIVITY_CLEAR_TASK);
startActivity(intent);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.menu_main, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
//noinspection SimplifiableIfStatement
switch (id)
{
case R.id.action_logout:
ParseUser.logOut();
navigateToLogin();
break;
case R.id.action_addfriends:
Intent intent = new Intent(this,EditFriendsActivity.class);
startActivity(intent);
break;
case R.array.camera_choices:
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setItems(R.array.camera_choices,mlisitner);
AlertDialog dialog = builder.create();
dialog.show();
break;
}
return super.onOptionsItemSelected(item);
}
@Override
public void onTabSelected(ActionBar.Tab tab, FragmentTransaction fragmentTransaction) {
// When the given tab is selected, switch to the corresponding page in
// the ViewPager.
mViewPager.setCurrentItem(tab.getPosition());
}
@Override
public void onTabUnselected(ActionBar.Tab tab, FragmentTransaction fragmentTransaction) {
}
@Override
public void onTabReselected(ActionBar.Tab tab, FragmentTransaction fragmentTransaction) {
}
/**
* A {@link FragmentPagerAdapter} that returns a fragment corresponding to
* one of the sections/tabs/pages.
*/
public class SectionsPagerAdapter extends FragmentPagerAdapter {
public SectionsPagerAdapter(FragmentManager fm) {
super(fm);
}
@Override
public Fragment getItem(int position) {
// getItem is called to instantiate the fragment for the given page.
// Return a PlaceholderFragment (defined as a static inner class below).
switch (position) {
case 0:
return new inboxfragment();
case 1:
return new Friendsfragment();
}
return null;
}
@Override
public int getCount() {
return 2;
}
@Override
public CharSequence getPageTitle(int position) {
Locale l = Locale.getDefault();
switch (position) {
case 0:
return getString(R.string.title_section1).toUpperCase(l);
case 1:
return getString(R.string.title_section2).toUpperCase(l);
}
return null;
}
}
}
2 Answers
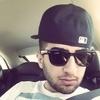
Vrezh Gulyan
11,161 PointsTry removing the break statement after case R.id.action_camera, I don't have one after mine and it might not be the problem but having it there might cause the dialog that should be shown to close right after creation.

Andre Colares
5,437 PointsHere is the functional code (ANDROID STUDIO 1.4):
protected DialogInterface.OnClickListener mDialogListener =
new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
switch (which){
case 0: //Take Picture
Intent takePhotoIntent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE);
// get path to save images
mMediaUri = getOutputMediaFileUri(MEDIA_TYPE_IMAGE);
if(mMediaUri==null){
//display an error
Toast.makeText(MainActivity.this,
R.string.error_external_storage,
Toast.LENGTH_LONG).show();
}else {
takePhotoIntent.putExtra(MediaStore.EXTRA_OUTPUT, mMediaUri);
startActivityForResult(takePhotoIntent, TAKE_PHOTO_REQUEST);
}
break;
case 1: //Take Video
break;
case 2: //Choose Picture
break;
case 3: //Choose Video
break;
}
}
private Uri getOutputMediaFileUri(int mediaType) {
//To be safe, you should check that the SDCard is mounted
//using Environment.getExternalStorageState() before doing this
if(isExternalStorageAvaible()){
//Get the URI
//1. Get the external storage directory
String appName = MainActivity.this.getString(R.string.app_name);
File mediaStorageDir = new File(
Environment.getExternalStoragePublicDirectory(
Environment.DIRECTORY_PICTURES),
getString(R.string.app_name)
);
// create subdirectory
if ( ! mediaStorageDir.exists() ) {
if ( ! mediaStorageDir.mkdirs() ) {
Log.e(TAG, "Failed to create directory");
return null;
}
}
//3.Create a file name
File mediaFile;
//4. Create the file
Date now = new Date();
String timestamp = new SimpleDateFormat("yyyyMMdd_HHmmss", Locale.US).format(now);
String path = mediaStorageDir.getPath() + File.separator;
switch (mediaType) {
case MEDIA_TYPE_IMAGE:
mediaFile = new File(path + "IMG_" + timestamp + ".jpg");
break;
case MEDIA_TYPE_VIDEO:
mediaFile = new File(path + "VID_" + timestamp + ".mp4");
break;
default:
return null;
}
Log.d(TAG, "File: " + Uri.fromFile(mediaFile));
//5. Return the file's Uri
return Uri.fromFile(mediaFile);
}else {
return null;
}
}
private boolean isExternalStorageAvaible(){
String state = Environment.getExternalStorageState();
return state.equals(Environment.MEDIA_MOUNTED) ? true : false;
}
};