Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial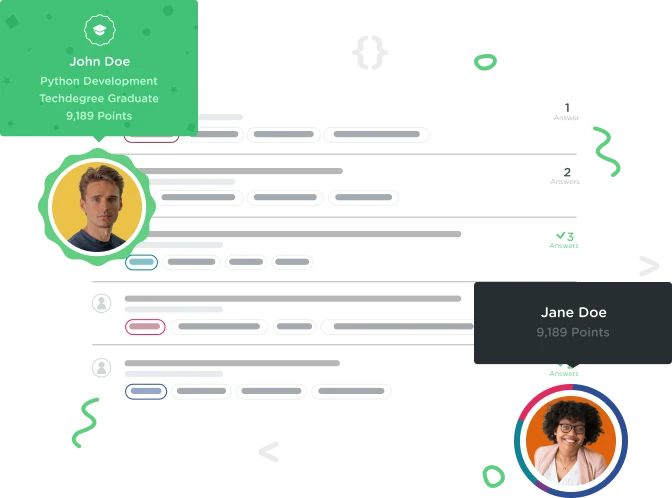
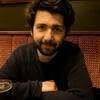
Dylan Cowling
Python Web Development Techdegree Student 2,590 PointsThe challenge is asking me to remove the vowels from a user input. I have done that but I am getting EOF errors.
Also, I'm required to look for uppercase and lowercase too but I'm not sure how to incorporate that into this yet. Either way, my code does its job as far as Workspaces tells me. What am I doing wrong here?
Here's the Task: "OK, I need you to finish writing a function for me. The function disemvowel takes a single word as a parameter and then returns that word at the end.
I need you to make it so, inside of the function, all of the vowels ("a", "e", "i", "o", and "u") are removed from the word. Solve this however you want, it's totally up to you!
Oh, be sure to look for both uppercase and lowercase vowels!"
word = list(input('Enter your word: '))
vowels = ["a", "e", "i" ,"o" ,"u"]
def disemvowel(word):
for vowel in word:
if vowel in vowels:
word.remove(vowel)
return word
print(disemvowel(word))
1 Answer

Cooper Runstein
11,850 PointsYou have a few problems, the most glaring is that there is no string method 'remove', so you're not going to return anything valid unless you change that. Second, right now you're only checking for lower case vowels, because your vowels array only includes lowercase vowels. You could either add uppercase letters to the vowels array, or just use the .lower() method on each character to convert it to a lowercase. Finally, not to do with functionality, but rather the readability of the code, I would highly recommend you don't call the characters 'vowel' unless you know they're actually vowels. When I read through your code, if I'm just skimming it, I would imagine the vowel variable comes from the vowels array, not the word string. I'd use 'char' or 'letter' instead, it won't have any impact on how the code runs, but it will make your code easier to read.
Here's what I would do:
def disemvowel(word):
vowels = ['a', 'e', 'i', 'o', 'u']
disemvowled_word = '' #create an empty string to add all non vowels to
for char in word:
if char.lower() not in vowels: #if the lowercase character is not contained in the vowels array
disemvowled_word+= char #add it to my empty string
return disemvowled_word
Dylan Cowling
Python Web Development Techdegree Student 2,590 PointsDylan Cowling
Python Web Development Techdegree Student 2,590 PointsThank you, this actually makes a lot more sense now! :D