Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial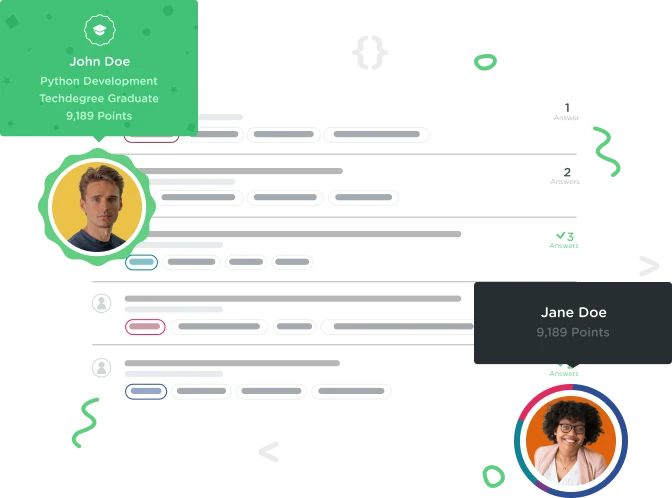
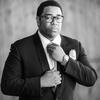
Franklyn Gregory
12,098 PointsThe code below logs all of the even numbers from 2 to 24 to the JavaScript console. However, there's a lot of redundant
I've been at this, and it's killing me I can't figure it out.
for ( i = 2; i <= 25; i++) {
console.log(i);
}
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
9 Answers

Rachel Lev
14,583 PointsYou have to add 2 to i in order to get the even numbers, and i should be less or equal to 24 not 25:
for (i = 2; i <= 24; i += 2) {
console.log(i);
}
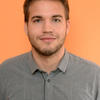
Adam Beer
11,314 PointsNo one learns from the finished code.
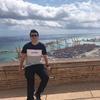
Tadjiev Codes
9,626 PointsWhy do we need += in the end of the code within () ? Thanks

Justyna Szofinska
6,178 Pointsfor ( let i = 2; i <= 24; i += 2) {
console.log ( i );
}
You need to add variable 'let'. I also had problem to finished it because i didn't erase previous code (console.log(2) console.log(4)... ect)
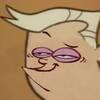
Andrew Duty
4,856 PointsThis is what got it for me, had everything right expect the "let"
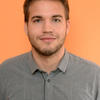
Adam Beer
11,314 PointsChallenge Task 1 of 1
The code below logs all of the even numbers from 2 to 24 to the JavaScript console. However, there's a lot of redundant code here. Re-write this using a loop.
console.log(2);
console.log(4);
console.log(6);
console.log(8);
and so on...
And that's how your code work:
for (var i = 2; i <= 25; i++) { // In other words, i++ equal to i += 1
console.log(i);
}
//the output
console.log(2);
console.log(3);
console.log(4);
console.log(5);
You are so close but it grows badly. This should be corrected and i <= 24. Hope this help.

Gheon Steenkamp
458 PointsNone of these codes work on this discussion

Kyffin Richmond
2,534 PointsSolved: The code uses a loop to display even numbers from 2 to 24. It starts at 2 and adds 2 repeatedly until it reaches 24, showing all the even numbers in between.
for (let i = 2; i <= 24; i += 2) {
console.log(i);
}
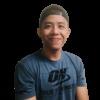
Faiz Hamdan
5,215 Pointsfor ( var I = 2; I <= 24; i+=2 ) {
console.log(i);
}
Hope this help Cheers!
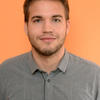
Adam Beer
11,314 PointsAnd again, No one learns from the finished code. Second, your first two I is capitalized, and the last is lowercase, why? Now your code doesn't work.
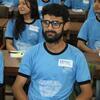
Kamal Ghimire
12,143 PointsHere the problem you should clear the coding area first and then start writing your loop code.

Celia Tawembe
5,598 Pointsfor (let i = 2; i <= 24; i += 2) { console.log(i); }

Sean White
5,537 PointsSyntaxError: script.js: Unexpected token <
(1:18)
I typed this in
for (let i = 2; i <= 24; i += 2) { console.log(i); }
I got the above error every time until I copied and pasted it from Celia's answer. It is exactly the same code, I checked it multiple times. But when I typed it in, I get the above error. Copy and paste it's fine. This is the second question this has happened for.
Dale Sellers
6,613 PointsDale Sellers
6,613 PointsI figured it out with the help of this MDN page: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/for
This is what worked for me:
for (var i = 2; i < 25; i += 2) { console.log(i); }