Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial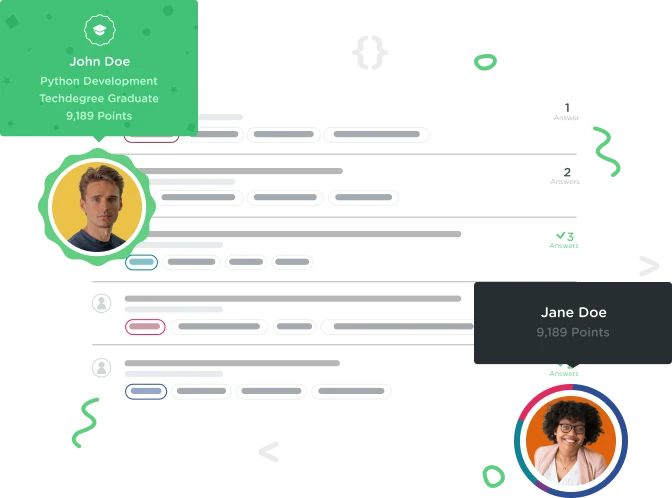

Ula Troc
1,881 PointsThe code does not work. Where did I make a mistake?
const gracze = [
{
name: "Guil",
score: 50,
id: 1
},
{
name: "Treasure",
score: 85,
id: 2
},
{
name: "Ashley",
score: 95,
id: 3
},
{
name: "James",
score: 80,
id: 4
}
]
function Header(props) {
return (
<header>
<h1>{props.title}</h1>
<span className="stats"> Players: {props.playersNumber}</span>
</header>
);
}
const Player = (props1) => {
return (
<div className="player">
<span className="player-name">
{props1.name}
</span>
<Counter counter1={props1.score}/>
</div>
);
}
class Counter extends React.Component {
render() {
return (
<div className="counter">
<button className="counter-action decrement"> - </button>
<span className="counter-score"> {this.counter1} </span>
<button className="counter-action increment"> + </button>
</div>
);
}
}
const App = (alejazda) => {
return (
<div className="scoreboard">
<Header
title="My Scoreboard"
playersNumber={alejazda.poczatkowigracze.length}
/>
{/* Players List */}
{alejazda.poczatkowigracze.map ( gracz =>
<Player
name={gracz.name}
score={gracz.score}
key={gracz.id.toString()}
/>
)}
</div>
);
}
ReactDOM.render(
<App poczatkowigracze={gracze}/>,
document.getElementById('root')
);
3 Answers

Shawn Casagrande
9,501 PointsYour problem is in your Counter class. It should be this.props.counter1 since the class is calling the props property where state is stored.
You can add console.log(this.props) just above the return in the render function to be able to see that info in the Chrome debugger console.

Dom Ss
4,339 PointsThank you Shawn for help! Its been almost a year since my last test on it. Actually, I got a job as a JS Full-Stack Developer. Now when I am looking at my mistakes, its so silly. ahahah
But back then it was a real challange.
I managed to get a job just after doing the JS track here in Treehouse.
It helped me TALK LIKE A PROGRAMMER so I could sell myself during job interview.

M C
12,105 PointsDef right here as far as I see, if you console.log(this) in the class Counter, you will see that these properties are part of the class.
context: {} props: {score: 80} refs: {} state: null updater: {isMounted: ƒ, enqueueSetState: ƒ, enqueueReplaceState: ƒ, enqueueForceUpdate: ƒ} reactInternalFiber: FiberNode {tag: 1, key: null, stateNode: Counter, elementType: ƒ, type: ƒ, …} _reactInternalInstance: {_processChildContext: ƒ} isMounted: (...) replaceState: (...) __proto_: Component
So props has to be used to access the value.
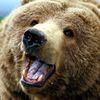
Ran Wang
Full Stack JavaScript Techdegree Graduate 32,191 PointsInteresting question, tried to find answer but I failed, in console, I noticed that the counter elements have their counter1={num} property, which should be ok if referenced by {this.counter1}, but the UI does not show the number.
I cant help you, but I have posted your question on slack. Will give you feedback if someone is able to find the answer.
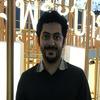
Bader Alsabah
4,738 PointsI believe Shawn's answer above hit the spot. Another way to look at it is that when you write
{this.counter1}
You are NOT referring to any numerical value or string, rather you referring to an object which you had defined earlier as
score: gracz.score
But in this case you want to access the object's value {this.props.counter1}
`
Unlike the previous uses of props in functions, where you can refer to properties as props or props1, props is a keyword within the Class context. So in the class declaration, we need to say "this instance of my class Counter has a props called counter1 and I want to display its' value.

Dom Ss
4,339 PointsThank you for trying this out !
Ula Troc
1,881 PointsUla Troc
1,881 PointsI specifically used different names to understand what is being passed where. Unfortunately Guil is uising the same name for props, name, score etc and at a later stage it becomes troublesome where I am passing what.
In order to understand props and component composition I wanted to use the different names but I am stuck at this point. The code stopped working when I changed the stateless function to a class component - I think, I am not sure. :D
The code brakes at line <span className="counter-score"> {this.counter1} </span>. So my question is, how to I display now the Counter component in Player and use the this.props.counter1 when there is not props in the class component.
Plox help