Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial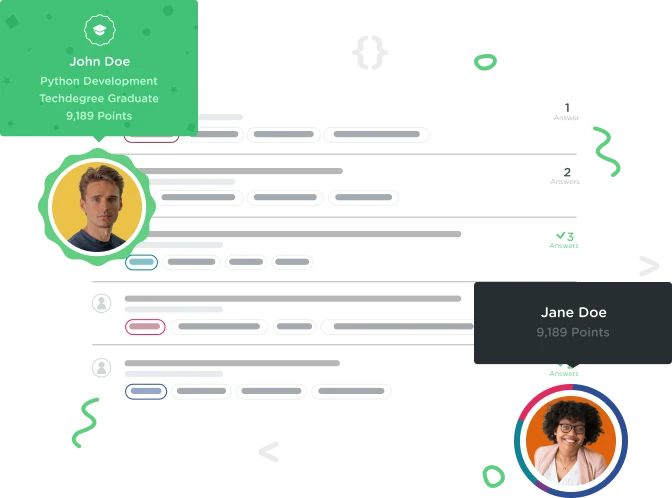
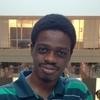
Christopher Jr Riley
35,874 PointsThe code I wrote in the challenge was only considered correct because I changed 'let' to 'var'. Why?
In the code challenge, it asks to add a dequeue method that removes the first element of the array and returns said element. I typed it up in Xcode playgrounds and it didn't complain. The code you see below is what I wrote up:
struct Queue<Element> {
var array: [Element] = []
var isEmpty: Bool {
if array.count == 0 {
return true
} else {
return false
}
}
var count: Int {
return array.count
}
mutating func enqueue(_ value: Element) {
array.append(value)
}
mutating func dequeue() -> Element? {
if array.count == 0 {
return nil
}
let valueToRemove = array.first
array.remove(at: 0)
return valueToRemove
}
}
However, this was considered as incorrect and gave me this reason:
"The stored property needs to be declared as a variable and not a constant"
The only thing that's not a variable is on line 25, shown below:
let valueToRemove = array.first
I changed it to var...
var valueToRemove = array.first
... and, in spite the fact that Xcode playgrounds is telling me to consider changing it back to "let", the code was considered as correct.
I don't understand why this is the case, as it shouldn't matter either way. Why is it now correct, but the previous attempt wasn't?
For reference, here's the code that the Code Challenge considered as correct:
struct Queue<Element> {
var array: [Element] = []
var isEmpty: Bool {
if array.count == 0 {
return true
} else {
return false
}
}
var count: Int {
return array.count
}
mutating func enqueue(_ value: Element) {
array.append(value)
}
mutating func dequeue() -> Element? {
if array.count == 0 {
return nil
}
var valueToRemove = array.first
array.remove(at: 0)
return valueToRemove
}
}