Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial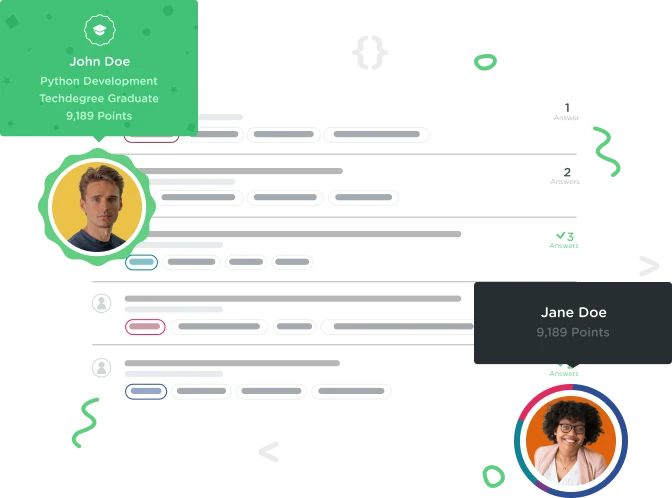

Postil Postil
20,524 PointsThe code is working well, but the system does not accept it.
i have tested this code on workspace and it seems to work well. dont know why it is not taking it as a valid answer
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(a_string):
lower_a_string = a_string.lower()
word_list = []
word_dict = {}
new_word_dict = {}
new_word = ''
count=0
word_list = lower_a_string.split(' ')
for word in word_list:
try:
word_dict.update({word:word_dict[word]+1})
except:
word_dict.update({word:1})
continue
return word_dict
1 Answer
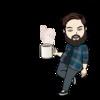
Philip Schultz
11,437 PointsHey Postil, Your code will work if you remove the quotations in the split method. Also, this is how I did the challenge.
Here it is all together-
def word_count(string):
list_of_words = string.lower().split()
dict_words = {}
for word in list_of_words:
dict_words[word] = list_of_words.count(word)
return dict_words
This is how I broke this challenge apart- I first took the string argument and made it all lowercase, by using the 'lower' function, then I put the words into a list by using the split method. Like so,
list_of_words = string.lower().split()
**Note how I'm using the lower method and split method on the same line. if you do it this way make sure that the lower() is before the split(). Also, notice that you don't need quotations in the parenthesis of the split function.
Then I created an empty dict. I notice that you made a couple in yours, but only one is needed.
dict_words = {}
Now creating the for loop. This should be pretty straight-forward now that it is a list. So, when you loop through the list of words, you have all the information you need to make the key and value for the dict.
for word in list_of_words:
dict_words[word] = list_of_words.count(word)
return dict_words
**If you haven't seen the count method yet take a look at this doc to see how it works (https://www.programiz.com/python-programming/methods/string/count)
Let me know if you have questions and I'll try to answer it the best I can.
Grigorij Schleifer
10,365 PointsGrigorij Schleifer
10,365 PointsNice explanation! If you could, I would upvote it twice