Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial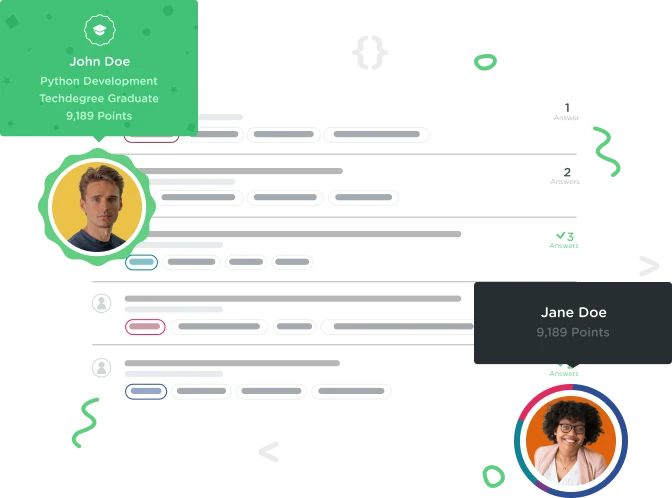

Rahul Nayak
863 Pointsthe code not working for creating a constructor
here is my dice.js code
function dice(sides) {
this.sides = sides;
this.diceRoll = function() {
var randomNumber = Math.floor(Math.random() * this.sides) + 1;
console.log (randomNumber);
}
}
var dice6 = new dice(6);
and here is the ui.js code
function printNumber(number) {
var placeholder = document.getElementById("placeholder");
placeholder.innerHTML = number;
}
var button = document.getElementById("button");
button.onclick = function() {
var result = dice.diceRoll();
printNumber(result);
};
Whenever I click on button, the placeholder displays nothing. Does anyone know where I am going wrong ?
5 Answers
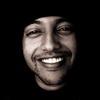
miikis
44,957 PointsHey Rahul,
Your code is kinda all over the place... I'm not really sure where to start because Idk what you already do and don't know. Below is a working implementation of what you're trying to do. Feel free to ask any specific questions you may still have.
Here's an index.html:
<p id="placeholder">Hello World!</p>
<button id="button">Roll the Dice!</button>
<script src="./dice.js"></script>
Here's a dice.js:
const button = document.getElementById('button')
const placeholder = document.getElementById('placeholder')
function Dice(sides) {
this.sides = sides || 6
}
Dice.prototype.roll = function () {
return Math.floor(Math.random() * this.sides) + 1
}
button.addEventListener('click', function () {
const dice = new Dice()
placeholder.innerHTML = dice.roll()
})
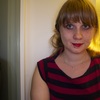
Karolin Rafalski
11,368 Pointsfor your dice.js code: constructor functions are conventionally named with a capitol letter, so it should be named Dice, not dice. I would add a last line, to see if your function is doing what you want, you can delete this once you know you have what you want:
console.log( dice.6sides, dice6.diceroll)
Then, I would also add console.logs for your ui.js files to check to see if what you have written is given what you expected
console.log('this is the button ' + button);
console.log('this is the placeholder ' + placeholder);
button.onclick = function () {
console.log ('button has been clicked!')
...
}
If you see the console.logs not working or not given you what you expected or not firing when expected, you can narrow down where a problem in your code might be. console.log is a great way to start learning to troubleshoot and debug your code. Delete the console.logs when you are done with them to keep your code looking clean.

Cindy Lea
Courses Plus Student 6,497 PointsTry something like this:
function dice(sides) {
this.sides = sides || 6;
this.diceRoll = function () {
return Math.floor(Math.random() * this.sides) + 1;
};
}
var d6 = new Dice(6);
Its a little different from your, but is this the effect you're looking for?

Rodrigo Castro
15,652 PointsHi Rahul,
The problem is that in your dice contructor you are logging the randomNumer when you should be returning it
function dice(sides) {
this.sides = sides; this.diceRoll = function() { var randomNumber = Math.floor(Math.random() * this.sides) + 1; //WRONG///// console.log (randomNumber); //CORRECT//// return randomNumber; } }

Ashi A
2,531 PointsAlso, it looks like you're calling the dice.roll() method on the button on-click event in the UI.js file. You should either match "dice" to your instance name (dice6 in your example) or change the name of the instance to "dice" in order to get the dice.diceRoll() method to work .
Also you named your method diceRoll() but the UI.js still refers to "dice.roll()" Suggest keeping it consistent.
delanobartolomei
Full Stack JavaScript Techdegree Student 14,052 Pointsdelanobartolomei
Full Stack JavaScript Techdegree Student 14,052 PointsI like this snippet, it's awesome!