Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial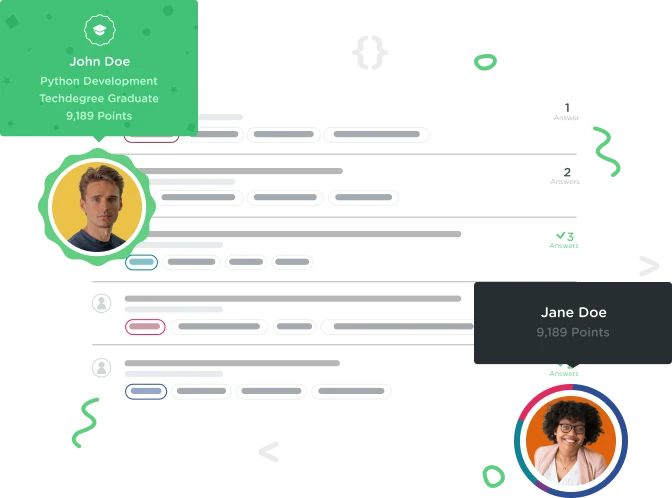

Daniel Springer
Courses Plus Student 5,090 PointsThe code runs twice
Hi All,
I have a small question. I wanted to set this Code up so that I create the function, inside the function I check if the input is NaN, once that passes I collect the inputs, and create an alert. I also wanted to collect all the outputs and create a message on the HTML page displaying all the collected outputs from the functions. For some reason the prompt input collection runs twice and the first input is ignored at the end. Once the end result is correct and the HTML msg is displayed, but i don't understand why the prompt comes twice?
If anyone could give me any hints why this is going on I would really appreciate it Here my code:
// 2. Create a function that calculates the area of a rectangle. // The function should accept the width and height as arguments // and return the area of that rectangle. // The area of a rectangle is the width * height
code
var message;
function area(width, height){
while(isNaN(width)){
width = prompt("what is the width of the circle")
width = parseFloat(width)
if(isNaN(width)){
alert("The width input is not a number, try again")
}
}
while(isNaN(height)){
height = prompt("what is the height of the circle")
height = parseFloat(height)
if(isNaN(height)){
alert("The height input is not a number, try again")
}
}
return width * height
}
alert("The area of the circle is " + area())
message = "<h3>The area of the circle is " + area() + "</h3>";
document.write(message);
6 Answers
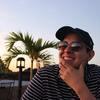
Juan Diaz
7,661 PointsThat seems like a reasonable answer, but be careful. Note that "area" is a variable declared INSIDE the function, therefore it only will work inside that scope, not outside. Reason why your "message" variable cannot reach the result of "area". The solution for this is to declare the function in the "global" scope, in other words. Declare "area" at the beginning and make sure you write area = width * height
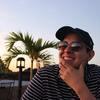
Juan Diaz
7,661 PointsFortunately this is an easy fix, the reason why you're getting this run twice is because you're calling the function area() twice. Once when you want to send the alert and the other when you print the message to the screen. Nothing wrong with the rest of the code.
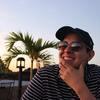
Juan Diaz
7,661 PointsIf you'd like to display the answer in both the alert and the screen, you might want to do this … totalArea = width * height // declare totalArea first alert("The area is " + totalArea) return width * height } … and then run your code to print it on the screen. Hope it was helpful!

Daniel Springer
Courses Plus Student 5,090 PointsHi Juan,
Thanks for the help, that makes sense. I was just going to ask you how I can export the return value of the function. I had tried this before, but that did not work.
var message;
function area(width, height){
while(isNaN(width)){
width = prompt("what is the width of the circle")
width = parseFloat(width)
if(isNaN(width)){
alert("The width input is not a number, try again")
}
}
while(isNaN(height)){
height = prompt("what is the height of the circle")
height = parseFloat(height)
if(isNaN(height)){
alert("The height input is not a number, try again")
}
}
var area width * height
return area
}
alert("The area of the circle is " + area())
message = "<h3>The area of the circle is " + area + "</h3>";
document.write(message);

Daniel Springer
Courses Plus Student 5,090 PointsHi Juan,
I don't know where to put totalArea?
I have tried this :
code
var message;
function area(width, height){
totalArea = width * height
while(isNaN(width)){
width = prompt("what is the width of the circle")
width = parseFloat(width)
if(isNaN(width)){
alert("The width input is not a number, try again")
}
}
while(isNaN(height)){
height = prompt("what is the height of the circle")
height = parseFloat(height)
if(isNaN(height)){
alert("The height input is not a number, try again")
}
}
return totalArea
}
alert("The area of the circle is " + area())
message = "<h3>The area of the circle is " + totalArea + "</h3>";
document.write(message);

Daniel Springer
Courses Plus Student 5,090 PointsHi Juan,
I got it, took me a moment thought.
Thanks for the help!
Here the working code
code
var message;
var totalArea;
function area(width, height){
while(isNaN(width)){
width = prompt("what is the width of the circle")
width = parseFloat(width)
if(isNaN(width)){
alert("The width input is not a number, try again")
}
}
while(isNaN(height)){
height = prompt("what is the height of the circle")
height = parseFloat(height)
if(isNaN(height)){
alert("The height input is not a number, try again")
}
}
return totalArea = width * height
}
alert("The area of the circle is " + area())
message = "<h3>The area of the circle is " + totalArea + "</h3>";
document.write(message);
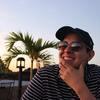
Juan Diaz
7,661 PointsYOU GOT IT! well done. :-)
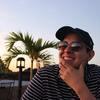
Juan Diaz
7,661 PointsSorry for the misunderstanding. Declaring area should be OUTSIDE the function.
var message;
var area;
function area(width, height){
while(isNaN(width)){
width = prompt("what is the width of the circle")
width = parseFloat(width)
if(isNaN(width)){
alert("The width input is not a number, try again")
}
}
while(isNaN(height)){
height = prompt("what is the height of the circle")
height = parseFloat(height)
if(isNaN(height)){
alert("The height input is not a number, try again")
}
}
area = width * height
return area
}
alert("The area of the circle is " + area())
message = "<h3>The area of the circle is " + area + "</h3>";
document.write(message);