Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial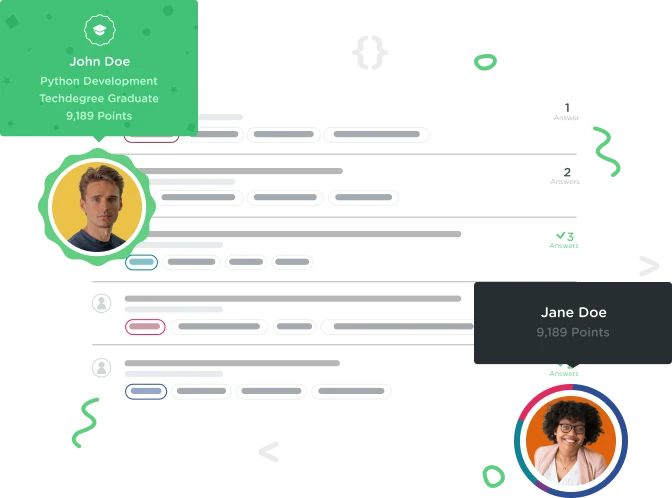

J J
15,775 PointsThe company you work for wants to send an email to its member base. They want the email to contain a complementary offer
The company you work for wants to send an email to its member base. They want the email to contain a complementary offer based on their membership level.
There is a send_offer function ready for you to use that accepts the following arguments: $member_id, $email, $fullname, $level
The data from the database is currently in a PDOStatement object named $results. Loop through those results and pass them to the send_offer function.
Help please
<?php
include "helper.php";
$results->fetchaLL(PDO::FETCH_NUM);
/*
* helper.php contains
* $results->query("SELECT member_id, email, fullname, level FROM members");
*/
7 Answers
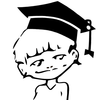
simhub
26,543 PointsHi J,
you could try this:
$user = $results->fetchAll(PDO::FETCH_ASSOC);
foreach ($user as $key)
{
echo send_offer($key['member_id'],$key['email'],$key['fullname'],$key['level']);
}
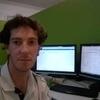
Gavin Mace
30,747 PointsThanks - Just used this and it worked fine.
So annoying that the question says "Tip: we used this method in the last video", when all we did in the previous video was $catalog = $results->fetchAll();
All the code that successfully answered this isn't covered in this course :/
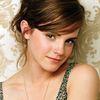
Ogün Murat Kara
3,726 Pointstreehouse why dont you stop asking questions you don't teach?

Alain Readman Valiquette
14,547 Points$members = $results->fetchAll(PDO::FETCH_ASSOC);
foreach ($members as $member) {
$member_id = $member[member_id];
$email = $member[email];
$fullname = $member[fullname];
$level = $member[level];
send_offer($member_id, $email, $fullname, $level);
}
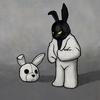
Nikifor Nikolov
8,593 PointsActually this is manipulating with arrays. You should know that. If not you could check again Basic PHP course (https://teamtreehouse.com/library/php-arrays-and-control-structures)
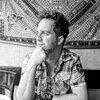
jlampstack
23,932 PointsI understand what the loop is doing and how the send_offer() function works, but I just don't understand the syntax. for $member[id], $member[email], $member[fullname], $member[level]. I understand what is happening and that [id], [email], [fullname], [level] point to the columns we've selected in the SELECT statement. I just don't understand the syntax nor recall learning this?
- Why the square [ ] brackets ?
- Why is these text in the square brackets not not in quotations? ie.) [" "]
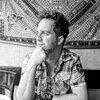
jlampstack
23,932 PointsRevisiting this a year later with a better understanding, it's really quite simple. Try not to overthink it.
- $members is an associative array filled with objects.
- Each $member object is an Associative Array containing key / value pairs
- Square brackets [] are how we access values of indexed array items
In other words....
$member['email'] would access a value of the particular instance of the object it is looping thru. It would return something like "myname@email.net"

Alain Readman Valiquette
14,547 Points$members = $results->fetchAll(PDO::FETCH_ASSOC); foreach ($members as $member) { $member_id = $member[member_id]; $email = $member[email]; $fullname = $member[fullname]; $level = $member[level];
send_offer($member_id, $email, $fullname, $level); }

Alain Readman Valiquette
14,547 Points''' $members = $results->fetchAll(PDO::FETCH_ASSOC); foreach ($members as $member) { $member_id = $member[member_id]; $email = $member[email]; $fullname = $member[fullname]; $level = $member[level];
send_offer($member_id, $email, $fullname, $level); } '''
David Negron
Courses Plus Student 3,669 PointsDavid Negron
Courses Plus Student 3,669 PointsDepending on the fetch mode, you can loop through the results, which come back as an array, like so:
The structure of your data depends on the fetch mode. If you use FETCH_NUM, you'll get a numeric array. If you use FETCH_ASSOC, you'll get an array, with the column names from the db as keys.