Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial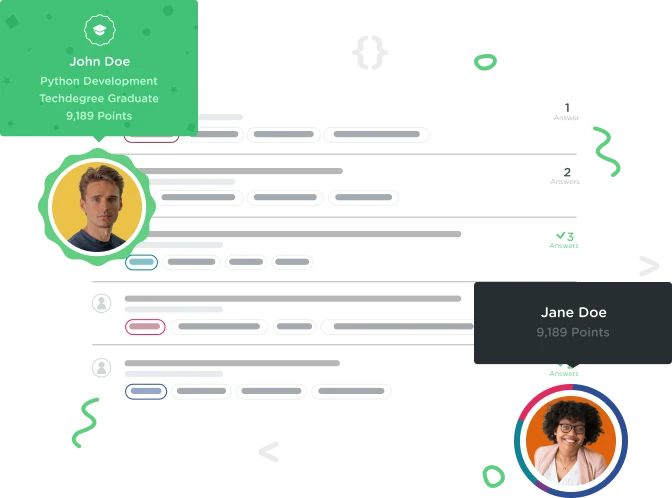

studentswift
9,476 PointsThe correct result doesn't make sense. Please explain why its correct.
Here is the description of the challenge.
Challenge Task 4 of 4
Great job so far! Clearly you know how to add and read items from the array so in this challenge let's try getting rid of a value.
For this task, remove the 6th item from the array and assign the result to a constant named discardedValue.
To remove an item, use the method removeAtIndex() and put the index number in between parentheses that you want to remove.
MY ANSWER:
var arrayOfInts = [10, 20, 30, 40, 50, 60, 70, 80]
arrayOfInts.removeAtIndex(5)
let discardedValue = arrayOfInts.removeAtIndex(5)
// discardedValue is 70
I'm removing 6th item from the array, which is 60 then when I'm assigning result to a constant named discardedValue and this is where things are getting tricky. I checked what "discardedValue" returns and its not 60 it's the following number in the array 70. Why is it the right answer? I'm suppose to assign result to a constant, which is suppose to be the same result of arrayOfInts.removeAtIndex(5) 60. I'm confused.
2 Answers
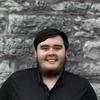
Michael Hulet
47,912 PointsNotice that you're calling removeAtIndex(5)
twice. Before you call it the first time, the array looks as it originally was, like this:
var arrayOfInts = [10, 20, 30, 40, 50, 60, 70, 80]
Then when you call it for the first time, it removes the item at index 5
from the list, which is 60
. When it's done, the array looks like this:
var arrayOfInts = [10, 20, 30, 40, 50, 70, 80] //Notice that 60 is not in the array
After that, you assign to the constant discardedValue
by calling removeAtIndex(5)
, like you did before. The difference is that the item at index 5
is different than it was last time, because you deleted it before, which shifted everything after that down an index. Because of this, 70
is at index 5
when you call removeAtIndex(5)
for the second time, and it thus gets assigned to the constant discardedValue

jag
18,266 Pointsvar arrayOfInts = [10, 20, 30, 40, 50, 60, 70, 80]
print(arrayOfInts) // returns [10, 20, 30, 40, 50, 60, 70, 80]
print(arrayOfInts.removeAtIndex(5)) // returns 60
print(arrayOfInts) // returns [10, 20, 30, 40, 50, 70, 80]
let discardedValue = arrayOfInts.removeAtIndex(5)
print(discardedValue) // returns 70
print(arrayOfInts) // returns [10, 20, 30, 40, 50, 80]
// discardedValue is 70
// removeAtIndex removed the value making the new 6th value 70 and not 60