Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial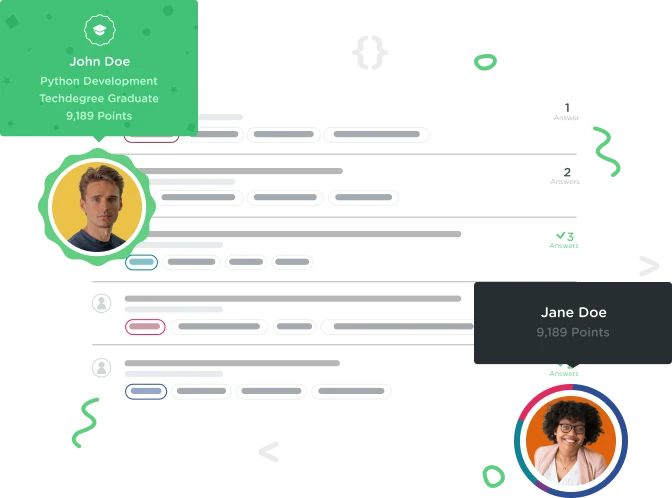
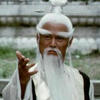
Chris Davis
16,280 PointsThe default switch case seems redundant
The default switch case would never run since we are already testing the value of the grade level in the elseif above the switch. Am i right in my thinking or am i missing something?
This is from the "PHP- Schools Out" lesson. Here is an example code...
<?php
//Switch Statement Project - Student Grade Analysis
$student = "Chris";
$grade = 12;
$average = 69;
$message = "";
if(!$student) {
//If the student name is left empty
echo("Please enter a student name");
}elseif($grade < 9 || $grade > 12) {
//If the student grade is less than 9 or more than 12
echo("Please enter a grade between 9 through 12 only!");
}else {
//If the student average is less than 70
if($average < 70) {
$message = "<p>We are sorry to inform you that you FAILED grade $grade because you are a dingus and your average was only <span style='color: red; font-weight: bold;'>$average</span>!</p>";
echo("<p>Dear $student,</p>");
echo($message);
}else {
//If the student grade is more than 70 construct a congratulatory message based on the student current grade
switch($grade) {
case 9:
$message = "<p>Congratulations! You passed the 9th grade with an average of <span style='color: green; font-weight: bold;'>$average</span>!</p>";
echo("<p>Dear $student,</p>");
echo($message);
break;
case 10:
$message = "<p>Congratulations! You passed the 10th grade with an average of <span style='color: green; font-weight: bold;'>$average</span>!</p>";
echo("<p>Dear $student,</p>");
echo($message);
break;
case 11:
$message = "<p>Congratulations! You passed the 11th grade with an average of <span style='color: green; font-weight: bold;'>$average</span>!</p>";
echo("<p>Dear $student,</p>");
echo($message);
break;
case 12:
$message = "<p>Congratulations! You graduated with an average of <span style='color: green; font-weight: bold;'>$average</span>! Now get outta hear! Lols!</p>";
echo("<p>Dear $student,</p>");
echo($message);
break;
default:
echo("Error, grade level is not 9-12!");
break;
}
}
}
?>
5 Answers

andren
28,558 PointsYou are correct, there is no expected situation in the current program that could cause the default clause to run. Though that is also why the action the default clause takes is to print an error message. Since it is never actually meant to be reached.
While it is a bit overkill for a simple application like this, it is considered to be good practice to always have a default case in switch statements. Even if you account for all of the expected values beforehand.
This is due to the fact that in more complex applications there will almost certainly be times when some kind of bug or mistake will lead things not to go to plan. And for one reason or another a switch statement will end up with a value different from what it was meant to handle. Rather than having the switch statement fail silently, it is better to have it print an error message or do something similar to make it obvious that something unexpected has happened in the code.
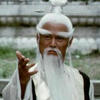
Chris Davis
16,280 PointsThanks so much for the explanation Andren

Glen Jensen
1,233 PointsIn the video she didn't echo out $messageBody in the switch. Does anyone know if this was intentional, or an error? I couldn't get my switch to display anything without echo $messageBody after setting it.
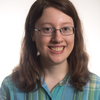
Jessica Foster
6,950 PointsHi Glen,
Yes, she actually did echo out $messageBody, but only once, at the very end of the code. Within the switch statement, she assigned different values to $messageBody based on the case, but she did not echo $messageBody until after the if statements and switch statements had all been processed.
Basically the code means...if the $finalAverage is greater or equal to 70, then determine the appropriate message based on $currentGrade, store that message in $messageBody, and then echo the student's $firstName and $lastName and then the $messageBody.
Cheers, Jess
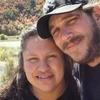
Michael Culler
7,363 Pointsi'm glad i'm not the only one who makes jokes with the code lol makes it a bit more fun to learn i.e. the disgusting student line lol
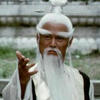
Chris Davis
16,280 PointsLols! We are sorry to inform you that you FAILED grade $grade because you are a dingus!
Shamefully taken from a one Dr. Steve Brule!

Carlos Vieira
Courses Plus Student 1,345 PointsYes. Also the break;
on default is ALWAYS redundant.