Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial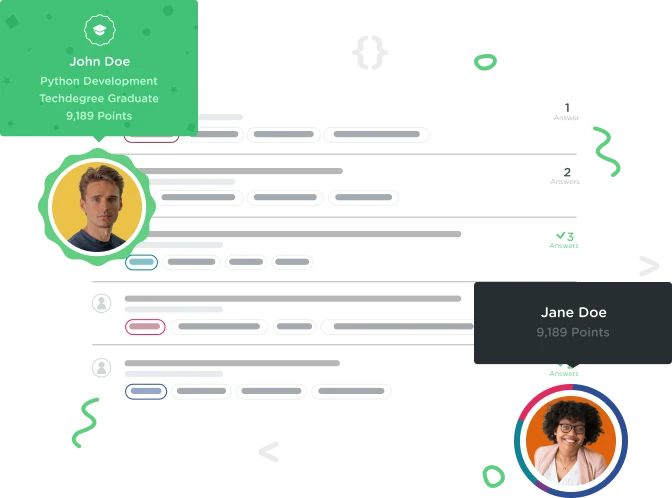
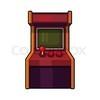
cyber voyager
8,859 PointsThe difference between arrays sets and objects?
This question emphasizes on sets, could you explain the differences between these 3? Why would I use one instead of the other?
Thank you very much, Stavros

Aaron Elliott
11,738 PointsThink of an array as a box.
var array = []
You can put stuff in this box.
array = ['toy', 'cow', 'alien']
array2 = [1, 1.2456, 3.14]
you can select items in an array by it's index; indexes start at 0.
array2[1] == 1.2456
An object is like an array but with a few differences. Instead of selecting a value by an index you select it by a key. It's like having a box of related items and all of those items have a tag to help you find it.
var obj = {key: 'value'}
//console.log( obj.key ) == 'value'
console.log( obj.key )
You can also have an array of objects. This is helpful in keeping related objects organized in you code.
var cars = [{make: 'Honda', model:'civic', engineType: 'gas' }, {make: 'Toyota', model:'Prius', engineType: 'Hybrid/gas' }]
//for each car in cars print the cars make
for(car in cars){
console.log( cars[car].make)
}
https://www.w3schools.com/js/js_object_definition.asp,
https://www.w3schools.com/js/js_arrays.asp
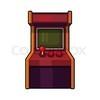
cyber voyager
8,859 Pointsreggie mikel and Aaron Elliot thank you very much for your efforts, both helped a great deal. I hope someone knows about sets, I didn't found anything solid on the web but I am going to try again! Anyways thank's again :)
3 Answers

Alexander La Bianca
15,959 PointsYou state a valid question. In fact, in many programming languages Objects like in javascript are called associative arrays. Meaning - you access the individual properties by 'associating' a value with a key.
Regular arrays are 'Indexed Arrays' where as you already know you access individual properties by their index.
So the major difference between the two is that a regular array is indexed, where an object contains associations.
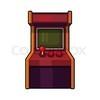
cyber voyager
8,859 PointsAhahaha I can't ask a correct question, my problem is with the (Map, Set) what are those? I know they are some form of an object but what exactly are them. I am so sorry for making you type all of this :/ I will try to make my questions more clear from now on. Thanks again.. Stavros :)

Alexander La Bianca
15,959 PointsYou are correct, Map and Set are both objects. However, an Array is technically an object as well. You can think of them as data structures. Array, Map and Set differ in the way you can store data and retrieve data.
//Array - just a list of things
var shoppingList = new Array();
shoppingList = ["eggs", "milk", "oj"];
//Set - an object with some useful methods to add/remove from the Set such as .add() and .has()
//A set can only contain UNIQUE values.
var mySet = new Set();
mySet.add(1); //mySet = {1}
mySet.add(2); // mySet = {1,2}
mySet.has(1); //true
mySet.add(1); //mySet = {1,2} note how not another 1 was added as it already existed
//Map - probably the closest thing to an object that you already know as you can store key and value pairs
//In fact, they are so similar that just good old regular objects have been used in place of a Map in the past
//Now we can use Maps as it is a built in object in javascript now with some usefull methods.
When you can't decide between a Map and a regular object ask yourself the following questions: Are keys usually unknown until run time? Do you need to look them up dynamically? Do all values have the same type? Can they be used interchangeably? Do you need keys that aren't strings? Are key-value pairs frequently added or removed? Do you have an arbitrary (easily changing) number of key-value pairs? Is the collection iterated?
If 'Yes' to the above questions, then it may be helpful to use a Map.
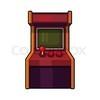
cyber voyager
8,859 PointsThank you so much, I can't express my gratitude enough. Thank you again :) I understood it.
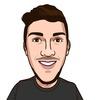
Daniel Kilders
36,793 PointsThanks for the explanation! This should be marked as best answer :)
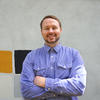
Tom Geraghty
24,174 PointsTo add on to what others have stated, this is just the language developing to more intuitively handle complex interactions. As software development deals with harder problems, people who write software end up finding patterns in the logic of their solutions. These patterns become recognized and used widely by other developers to make their software creation easier.
As these patterns get reused over and over, the people who decide what goes into a language sometimes incorporate those patterns and they become part of the core of the language's capabilities.
All computer programs do, essentially, is flip switches on and off (1 and 0). But we don't write programs by writing out huge series of 1s and 0s (at least, most people don't). These higher level abstractions like Boolean, Number, Array, Associative Array, Set, and so on are the evolution of the programming languages dealing with more and more complex problems.
It is certainly easier to write `var x = 5' than to write out the huge string of binary digits that the computer's transistors ultimately encode. It is also easier to use these higher level abstractions of data types (Number, Array, etc) to write solutions to more complex problems.
Long story short: Sets are used to store data types that should NOT be duplicated. There are a lot of math operations that rely on sets and knowing about those can help you design systems that use large amounts of data reliably.
MDN quote:
The Set object lets you store unique values of any type, whether primitive values or object references.

Aaron Elliott
11,738 PointsIf you have not completed it yet I would recommend the Javascript loops arrays and objects course.
https://teamtreehouse.com/library/javascript-loops-arrays-and-objects
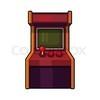
cyber voyager
8,859 PointsI have completed it but thanks, my question was more of what is the difference between sets and objects/arrays. and something else.. why do we use Map Set while we have arrays and objects? if we do console.log(typeof Map === Object) so why bother having all of these alternatives and where do they benefit? What are the differences between these? I hope you understand what I am trying to say, I am not that good with English.
Thanks
Reggie Williams
Treehouse TeacherReggie Williams
Treehouse TeacherI've noticed arrays to typically use one type of data, like an array of strings, while objects are used to hold multiple data types (strings, ints, methods). Not sure about sets but I hope that helps some