Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial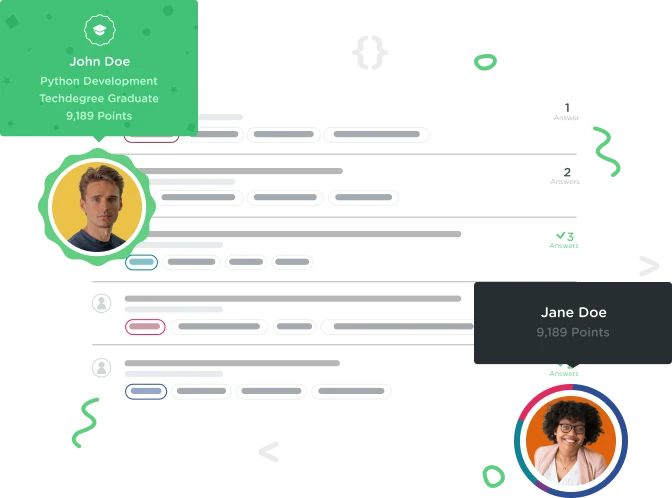
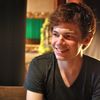
Andre Kovac
5,588 PointsThe different meanings of the * symbol
Just to get this straight, given the following code
function do_sth(*x) {
printf("Hello %p", x);
*x = *x - 3;
}
int a = 100;
do_sth(&a);
In *x
in the first line *
tells me that the parameter of the function has to be an address, right?
But that confuses me because I thought *x
either instantiates a pointer as in e.g.
NSString *some_string
or dereferences a pointer as e.g.
*some_string = "Hello";
So my question: What does *x
as a parameter of a function really tell me?
2 Answers

Gabe Nadel
Treehouse Guest TeacherWell, a pointer just points to an address in memory, so perhaps you aren't as confused as you think :)
Here is a blurb from a blogpost from a couple years back that covers the topic, full link below.
The asterisk () can be a little confusing. This is not part of the variable name. It can be placed anywhere between the data type and the variable name, so the following are all equivalent: NSString title; NSString * title; NSString *title; The * symbol is actually an operator that is used to de-reference a pointer. De-what a what now? Pointers are pretty much what they sound like. They βpointβ to a location in memory where the actual data is stored. That location in memory is the true container that holds the data. Pointers are a part of regular C and are used because they are more efficient. When we use pointers in our programs, we only need to store and copy a simple pointer, which is really just an address for a space in memory. Itβs a relatively small piece of data. If we instead had to store and copy the data being pointed to, we might very quickly run into problems of not having enough memory. For example, itβs much more efficient to simply point to the location for a large video file and use that pointer multiple times in code than to actually have to use all the data of that large video file every time we access it in code. Okay, so back to the asterisk: what does it mean to de-reference a pointer? It simply means that we obtain the value stored in the memory where the pointer is pointing to.
http://blog.teamtreehouse.com/the-beginners-guide-to-objective-c-language-and-variables

Gabe Nadel
Treehouse Guest TeacherHey Andre,
I think checking out this video will explain it better than I could just by typing. Fi that doesn't help, let me know.
https://teamtreehouse.com/library/objectivec-basics/beyond-the-basics/pointers-part-1
Andre Kovac
5,588 PointsAndre Kovac
5,588 PointsThanks for the link, Gabe! A nice article. However, I believe I understand WHAT a pointer is, I just get confused by the SYNTAX by which it is represented in different context. To me this syntax sometimes seems contradictory.
For example, in objective c, the following two variable declarations are equivalent, but to me they are very confusing:
Here in
sentence1 = @"Hello";
The NSString value is assigned to the address sentence1 (because in the lineNSString *sentence1;
the variable sentence1 is declared as a pointer and in the second line it is not dereferenced).NSString *sentence2 = @"Hello";
But here the NSString value is assigned to the dereferenced value, i.e. to *sentence2
So, I am not looking for a general explanation of pointers, but an explanation of this example and the one I mentioned in my above question.