Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial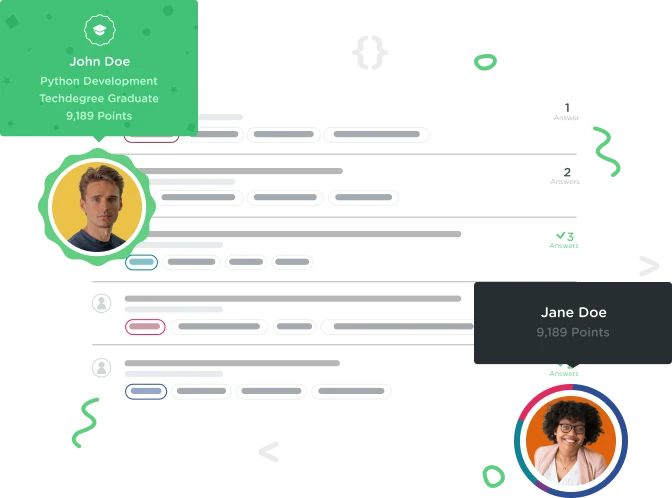
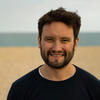
Matt Roberts
5,173 PointsThe elements are not changing purple or red respectively and I don't know why
I'm not seeing any errors in the console so hard to debug
app.js
const myList = document.getElementsByTagName('li');
for (let i = 0; i < myList.length; i += 1) { myList[i].style.color = 'purple'; }
const errorNotPurple = document.getElementsByClassName('error-not-purple');
for (let i = 0; i < errorNotPurple.length; i += 1) { errorNotPurple[i].style.color = 'red'; }
html <!DOCTYPE html> <html> <head> <title>JavaScript and the DOM</title> <link rel="stylesheet" href="css/style.css"> </head> <body> <h1 id="myHeading">JavaScript and the DOM</h1> <p>Making a web page interactive</p> <input type="text" id="myTextInput1"> <button id="myButton">change headline colour</button> <input type="text" id="myTextInput2"> <button id="myButton2">change button colour</button> <script src="app.js"></script> <p>Things that are purple:</p> <ul> <li>grapes</li> <li class="error-not-purple"> oranges</li> <li>amethyst</li> <li class="error-not-purple"> firetruck</li> <li>lavender</li> <li class="error-not-purple"> computer </li> <li>plums</li> </ul>
</body> </html>
4 Answers
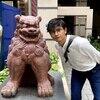
Carlos Chavez
5,691 PointsI found that the position of your script element was not at the end of you html file. This also caused problem for me before.
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
<link rel="stylesheet" href="css/style.css">
</head>
<body>
<h1 id="myHeading">JavaScript and the DOM</h1>
<p>Making a web page interactive</p>
<input type="text" id="myTextInput1">
<button id="myButton">change headline colour</button>
<input type="text" id="myTextInput2">
<button id="myButton2">change button colour</button>
<p>Things that are purple:</p>
<ul>
<li>grapes</li>
<li class="error-not-purple"> oranges</li>
<li>amethyst</li> <li class="error-not-purple"> firetruck</li>
<li>lavender</li> <li class="error-not-purple"> computer </li>
<li>plums</li>
</ul>
<script src="app.js"></script>
</body>
</html>
Here's another thread about it: https://teamtreehouse.com/community/how-does-the-order-in-which-you-place-your-html-elements-matter

Alzubair Alshehhi
2,792 PointsI have copied your code exactly and it worked, maybe you didn't save the code before preview?
HTML
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
<link rel="stylesheet" href="css/style.css">
</head>
<body>
<h1 id="myHeading">JavaScript and the DOM</h1>
<p>Making a web page interactive</p>
<p>Things that are purple:</p>
<ul>
<li>grapes</li>
<li class="error-not-purple"> oranges</li>
<li>amethyst</li>
<li class="error-not-purple"> firetruck</li>
<li>lavender</li>
<li class="error-not-purple"> computer </li>
<li>plums</li>
</ul>
<script src="app.js"></script>
</body>
</html>
app.js
const myList = document.getElementsByTagName('li');
for (let i = 0; i < myList.length; i += 1) {
myList[i].style.color = 'purple';
}
const errorNotPurple = document.getElementsByClassName('error-not-purple');
for (let i = 0; i < errorNotPurple.length; i += 1) {
errorNotPurple[i].style.color = 'red';
}
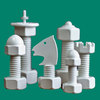
Steven Parker
231,268 PointsUnless I'm overlooking something because the code was posted without formatting, this works fine as-is.
A better way to share code and make your issue easy to replicate is to make a snapshot of your workspace and post the link to it here.

Harinder Singh
4,214 PointsI fixed it by changing all
<li class="error-not-purple">
to
<li class="errorNotPurple">
so it matches the way we wrote it in app.js