Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial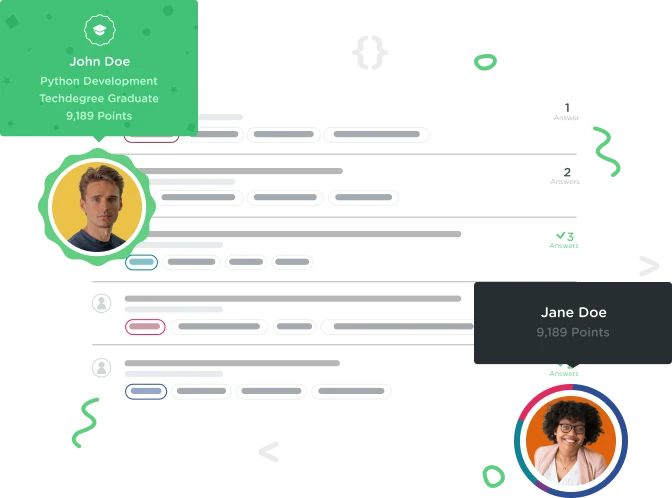

Timothy McCune
6,744 PointsThe exercise only allows users to use boolean keyword (lowercase b) but Javac and java-repl allow for Boolean.
While performing the "Harnessing the Power of Objects" code challenge, I kept getting an error when using: public Boolean isBatteryEmpty() { return mBarsCount == 0; }
Come to find out that it wouldn't accept Boolean (capital B). It was expecting boolean. However, the java compiler allows for Boolean & the java-repl returns java.lang.Boolean when typing Boolean expressions. I guess what I am saying is, if the java compiler and java-repl both allow for uppercase B or lowercase B, which one is the proper one? I was beating my head against the desk trying to figure out what was wrong with my code. I have been using capital Boolean since the beginning of this track. I guess I started because String has a capital S.
If both versions are accepted by java compiler and java-repl, perhaps the code challenge code should allow for it as well?
Thanks Tim
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void charge() {
mBarsCount = MAX_BARS;
}
}
2 Answers
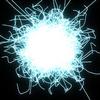
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHey There Timothy,
It accepts both in a java compiler, however the lower case b which the challenge wants you to use is correct.
The reason you can get around this is because boolean is both a primitive data type, and a pre-Defined class with methods built into it such as equals() and compare().
Treehouse blocks it because Craig is trying to teach us proper Syntax
The reason the compiler lets it go through is because it doesn't cause a syntax error.
let's say for instance you need to set a boolean variable up
boolean isTrue = true;
you use the lower case boolean because you are instantiating a primitive data type in Java, and Java Syntax is always to have primitive data types be lowercase.
However if you wanted to call a method from the Boolean class on your boolean object.
Boolean.toString(isTrue);
this would return the value of your boolean (true) back as a string, note that we aren't declaring a primitive variable in this, we are just calling the method from the Boolean Class, to string.
Think of it like the primitive data type char and class Character
char letter = "a";
but to call a method form the Character
Character.isLowerCase(letter); (Notice that these are not interchangable, the syntax will not allow char.isLowerCase(letter)
( this would throw a compiler error, because char isn't a class)
we'd actually have to use the uppercase representation, as primitive data types don't have methods on them.
As to why they named it Boolean and boolean and didn't short hand it to book is probably because they tried to make the class similar to people with a c programming language syntax.
Thanks, let me know if this doesn't help.

Craig Dennis
Treehouse TeacherHi Timothy,
Yeah this is one of those confusing parts of Java, you are definitely not alone here. I tried to not dive to deep to confuse things, but Boolean
and boolean
are different concepts. A boolean
is a primitive data type like int
and char
. Boolean
with the capital B is a wrapper type, there are also wrappers for Integer
and Character
. Wrappers are used like Rob stated to provide handy functions as well as to be used in places that require an Object
. Java provides a handy way of going between primitives and object references called Autoboxing.
You really should only use the Uppercased wrapper types when you need them, we'll touch a bit on them in Java Data Structures course, and we used Integer.parseInt
in Java Basics.
Hope that makes sense, and sorry for the confusion!