Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial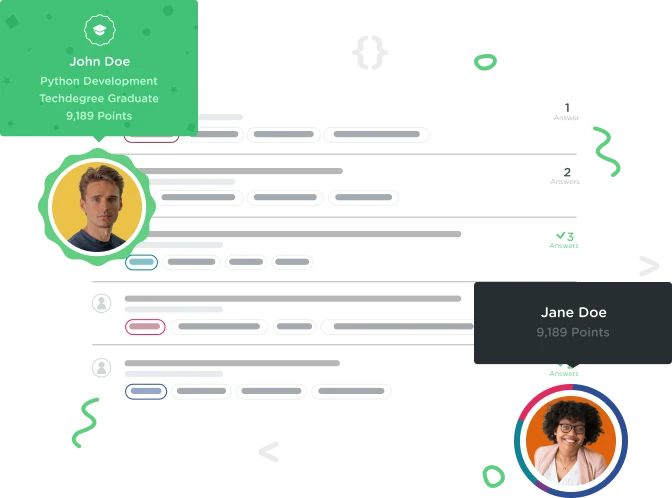

Sudip Paudel
2,203 PointsThe existing list items are removed when clicked on them but not the newly added items.
There are three list items that are defined in HTML. When I click on each item they are removed from the list. But, when I add new items to the list and click on them they don't get removed.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8"/>
</head>
<body>
<div id="main">
<li>grapes</li>
<li>apples</li>
<li>mango</li>
</div>
</br>
<input type ="text" id = "inputItem">
<input type ="button" id = "addItem" value ="Add Item">
<input type ="button" id = "removeLastItem" value ="Remove Last Item">
<script>
var inputItem = document.getElementById("inputItem");
var addItem = document.getElementById("addItem");
//Adds new item
addItem.addEventListener('click',function(){
var childLi = document.createElement("li");
var parentDiv = document.getElementById('main');
parentDiv.appendChild(childLi);
childLi.innerHTML=inputItem.value;
inputItem.value="";
});
//Remove the last item
removeLastItem.addEventListener('click',function(){
var lastItem = document.querySelector("li:last-child");
var parentDiv = document.getElementById('main');
parentDiv.removeChild(lastItem);
});
//Delete an existing element
var li = document.querySelectorAll('li');
for(var i= 0;i<li.length;i++){
li[i].addEventListener('click',function(){
var parentDiv = document.getElementById('main');
parentDiv.removeChild(this);
});
}
</script>
</body>
</html>

Sudip Paudel
2,203 PointsIt's my first question and had no idea. I will fix it now. Thank you,
2 Answers

Umesh Ravji
42,386 PointsHi Sudip, are you following the course exactly? I'm having a bit of trouble finding the part in the video with the code you are working on.
// Delete an existing element
var li = document.querySelectorAll('li');
for(var i= 0;i<li.length;i++){
li[i].addEventListener('click',function(){
var parentDiv = document.getElementById('main');
parentDiv.removeChild(this);
});
}
This code iterates over all of the list items and attaches a click event listener on each item. When the item is clicked, the item is removed. The problem is when new items are added to the list, the newly added items don't have this event listener attached to them, so clicking on them cannot remove them.
var main = document.getElementById('main');
main.addEventListener('click', function(event) {
if (event.target.tagName === 'LI') {
main.removeChild(event.target);
}
});
The best way to ensure that added items can also be removed is to add the click event listener to the parent of the list items, not the items themselves. This works by checking to see if the element that was clicked on was a list item, and if so, remove it. You learn about this later on in the course.
<ul id="main">
<li>grapes</li>
<li>apples</li>
<li>mango</li>
</ul>
Change the <div>
element to an unordered list <ul>
element too.

Sudip Paudel
2,203 PointsUmesh,
Thanks a lot for your help. Yes, I am following the course. I was just trying to something extra.

Umesh Ravji
42,386 PointsThat's cool, I was just wondering why I couldn't find it. Keep trying extra things for sure :)
Umesh Ravji
42,386 PointsUmesh Ravji
42,386 PointsIt's backticks you use to indicate markup, not quotes :)