Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial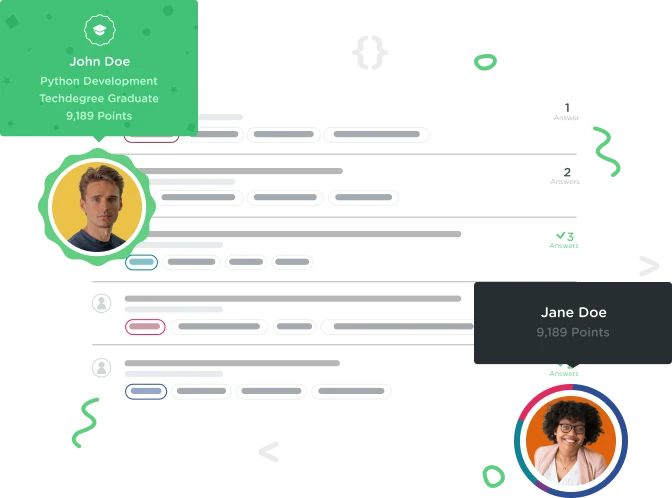

Adam Sturchio
3,500 PointsThe "final" project in the downloaded files for 2-convert-promise-handling-to-async-await
I think some of the source material for this video may have changed. In the promises section, one of the astronaughts listed had his wikipedia information removed, but since we were using promise.all, it wouldn't display anything. I figured that was a lone error, but when i moved on to this section i experienced something similar. I figured it was my own bad coding causing the error, so i decided to load up the "final project" to see what its suppose to look like and compare it to my code.
The problem:
It looks like Hazzaa Ali Almansoori is no longer valid in the wikipedia url. It caused breaking errors in the "promises" section, but only causes minor errors in this lesson. Is there some conditional code we can use to prevent the error? Or catch the error if there is no wiki for that person? I think it would go a long way to help prevent confusion in the future.
2 Answers

KRIS NIKOLAISEN
54,971 PointsThere is a wiki page but the spelling of the name is different. Instead of Hazzaa Ali Almansoori it is Hazza Al Mansouri. From the video's workspace I modified getProfiles
to use the new spelling.
function getProfiles(json) {
const profiles = json.people.map( person => {
const craft = person.craft;
var fetchurl = wikiUrl + person.name
if (person.name == "Hazzaa Ali Almansoori") {
fetchurl = "https://en.wikipedia.org/api/rest_v1/page/summary/Hazza_Al_Mansouri"
}
return fetch(fetchurl)
.then( response => response.json() )
.then( profile => {
return { ...profile, craft };
})
.catch( err => console.log('Error Fetching Wiki: ', err) )
});
return Promise.all(profiles);
}
I also changed generateHTML
based on this previous post to:
function generateHTML(data) {
data.map(person => {
const section = document.createElement('section');
peopleList.appendChild(section);
if (person.type === "standard") {
section.innerHTML = `
<img src=${person.thumbnail.source}>
<h2>${person.title}</h2>
<p>${person.description}</p>
<p>${person.extract}</p>`;
} else {
section.innerHTML = `
<h2>${person.title}</h2>
<p>No description available</p>`;
}
})
}
Hope it helps.

KRIS NIKOLAISEN
54,971 PointsAfter re-reading your post I downloaded the project files but it is the same workaround. In async-await.js I updated getPeopleInSpace
async function getPeopleInSpace(url) {
const peopleResponse = await fetch(url);
const peopleJSON = await peopleResponse.json();
const profiles = peopleJSON.people.map( async (person) => {
const craft = person.craft;
var fetchurl = wikiUrl + person.name
if (person.name == "Hazzaa Ali Almansoori") {
fetchurl = "https://en.wikipedia.org/api/rest_v1/page/summary/Hazza_Al_Mansouri"
}
const profileResponse = await fetch(fetchurl);
const profileJSON = await profileResponse.json();
return { ...profileJSON, craft };
});
return Promise.all(profiles);
}
and used the same code in my previous post for generateHTML