Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial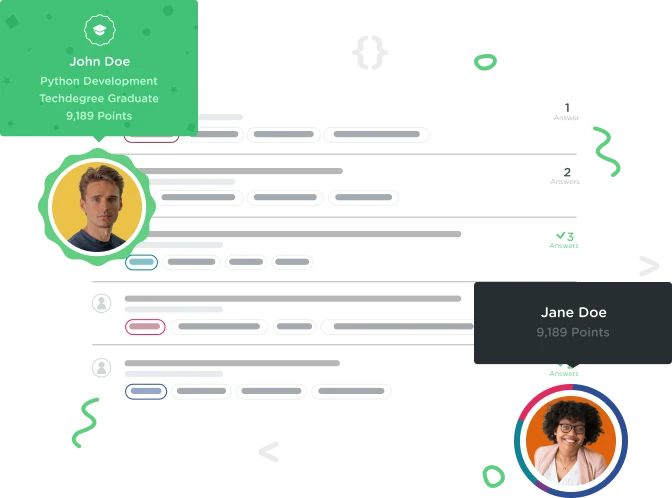

Marcatus X
834 PointsThe For Loop
class Prime {
public static void main(String args[]) {
int i, j;
boolean isprime;
for (i = 2; i < 100; i++) {
isprime = true;
//See if the number is evenly disible
for (j = 2; j <= i / j; j++) // if it is , then it's not prime.
{
if ((i % j) == 0) {
isprime = false;
}
}
if (isprime) {
System.out.println(i + " is prime.");
}
}
}
}
Alright, could someone please explain this code to me. I kinda understand it and ran it through the command prompt. But I still have some confusion with the for loop "for ( j=2; j <= i/j; j = ++)"...why do we have to divide i over j?
Running though the first sequence of the code: did j increment? I suppose not cause the condition j <= i/j---2<= 2/2 is not true. Correct me if I'm wrong...does i increment when the whole program is finished or before j? Please help!
2 Answers
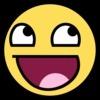
Michael Hess
24,512 PointsHi Marcatus X,
Well first of all, we know that a prime number is a number that is divisible by only one and itself -- and greater than 1. All of the prime numbers, except for 2, are odd numbers.
In the algorithm the first for-loop is looping through all numbers from 2 - 100. The variable i represents the number.
The for-loop nested inside of the first loop divides i by j, then the if statement is using a modulus to see if the remainder of i divided by j is equal to zero. If it is equal to zero the number is not prime.
If the number is prime it is printed to the console, if not, it is not printed to the console. This cycle will continue until all of the numbers in the first loop 2-100 have been checked to be prime.
A key part of this is understanding how nested for-loops work. This is a link to a nested for-loop tutorial on youtube: nested for loops tutorial
This is a really good problem to know. Being asked to create a method to check prime numbers is a common interview question.
If you have any other questions -- shout them out! Hope this helps!

Marcatus X
834 PointsThank You Michael. This sure helped me understand it better.
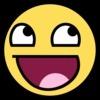
Michael Hess
24,512 PointsGlad I could help!