Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial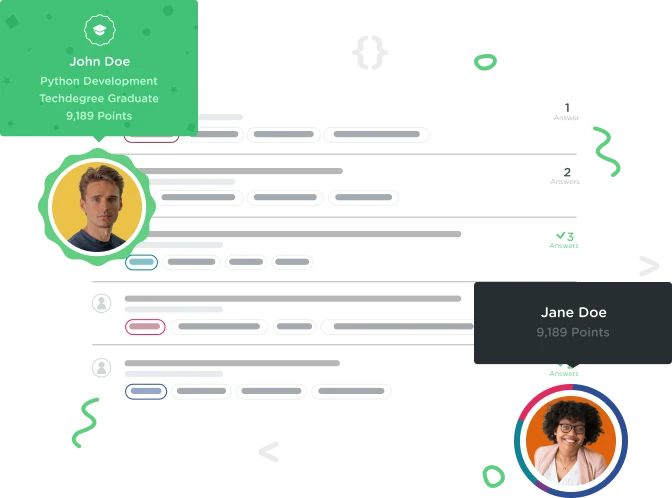

mo ode
4,352 PointsThe for loop cycles over list items and applies a color to each item using the values stored in the colors array.
The for loop cycles over list items and applies a color to each item using the values stored in the colors array. HELP
var listItems;
var colors = ["#C2272D", "#F8931F", "#FFFF01", "#009245", "#0193D9", "#0C04ED", "#612F90"];
for(var i = 0; i < colors.length; i ++) {
listItems[i].style.color = colors[i];
}
<!DOCTYPE html>
<html>
<head>
<title>Rainbow!</title>
</head>
<body>
<ul id="rainbow">
<li>This should be red</li>
<li>This should be orange</li>
<li>This should be yellow</li>
<li>This should be green</li>
<li>This should be blue</li>
<li>This should be indigo</li>
<li>This should be violet</li>
</ul>
<script src="js/app.js"></script>
</body>
</html>
11 Answers
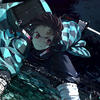
Spencer Bosko
2,000 PointsI know they mentioned going through teachers notes if we WANTED a more in=depth look at this. However. I reviewed all the videos when I encountered this challenge and not once were we introduced to using .children to create a list of objects. I had to google and find this page to get the answer. Wish they would have given us a challenge that we were prepared to complete without cheating.
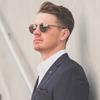
Cameron Sprague
11,272 PointsJust in case anyone else is still finding this thread here is the full JavaScript code below.
var listItems = document.getElementById('rainbow').children;
var colors = ["#C2272D", "#F8931F", "#FFFF01", "#009245", "#0193D9", "#0C04ED", "#612F90"];
for(var i = 0; i < colors.length; i ++) {
listItems[i].style.color = colors[i];
}
Best, Cameron Sprague

Mauricio Hernandez
7,208 PointsThank you and have a blessed week.

Linas Mackonis
7,071 PointsHi mo,
Your code should look like this:
var listItems = document.getElementsByTagName('li');
var colors = ["#C2272D", "#F8931F", "#FFFF01", "#009245", "#0193D9", "#0C04ED", "#612F90"];
for(var i = 0; i < colors.length; i ++) {
listItems[i].style.color = colors[i];
}

mo ode
4,352 PointsThanks. But I have to say THIS IS VERY HARD. HTML check CSS check JavaScript HELP!
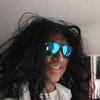
dahmeethook
7,165 Points@Linas Mackonis, the code you've shared above was not acceptable in the treehouse system. I tried to solve this on my own and couldn't.

Valeriya Kamenetska
15,832 PointsI am not sure why this comment is marked down. First of all, document.getElementsByTagName() has been discussed just prior to this challenge so it makes sense they would want us to use this solution. Unlike all previously suggested solutions that require using .children when this was not taught in previous material. Thank you Linas for this solution, much appreciated! :)
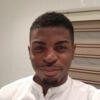
Nnanna Okoli
Front End Web Development Techdegree Graduate 19,181 PointsIt's 2022 and I believe Treehouse updated their question to this challenge for this answer to be correct assuming you have watched all the pre-requisite videos leading up to this challenge.
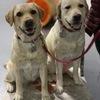
Alex Koumparos
Python Development Techdegree Student 36,887 PointsThe challenge is specifically testing the ability to work with a collection using the concept of children. As such, to pass the challenge you need to explicitly select the li
elements that are children of the ul
with the id
"rainbow". Here is the clue text from the challenge:
The collection should contain all list items in the
<ul>
element with the ID ofrainbow
.
We can do this by selecting the element with the specified id, and then modifying that selection to refer to its children:
var listItems = document.getElementById('rainbow').children;
Cheers,
Alex

Tony B
Front End Web Development Techdegree Student 10,702 PointsAh, ty! I wasn't sure how it wanted us to do it until I read this. I ended up doing a querySelectorAll on 'li' because that's what made sense in my head, and I just wanted to make it rainbow like it said. It passes, but it's not how they wanted it.
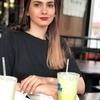
Azal Khaled
Full Stack JavaScript Techdegree Graduate 19,273 Pointsyou could have done this in a mutiple of ways because some element selectors do function the same. It just depends on preference! The key to getting the answer correctly are:
- defining the variable 'ListItems' using const
- setting listItems to the global variable document
- and using .childrent element selector before the semicolon (;)
Your choice of JS element selector is up to your preference...
Here is my answer:
const listItems = document.querySelector('#rainbow').children;

Quincey Hollman
1,923 PointsFinally found the info I needed to complete this code challenge the way I wanted to. Thank you so much for posting this.
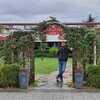
Hassan Al Manawy
24,859 PointsActually, the twist here is not with the code itself as this challenge could be addressed in multiple ways as shown below. It's just that you should declare the var listItems and your code before the "for loop", otherwise you would get this error.
In other words, the system exits the loop and then works on your code which has some unidentified objects. However, I think it should work fine with other text editors :)
Bummer: There was an error with your code: TypeError: 'undefined' is not an object (evaluating 'listItems[i]').
Correct Answers: var listItems = document.querySelector('#rainbow').children; var listItems = document.getElementById('rainbow').children; var listItems = document.getElementsByTagName('li');
just write any of the correct answers anywhere above the for loop (to be more specific; line 1 by declaring value within or anywhere between lines 1-3).
Hope that helps.
Regards, H

Joseph M.
2,057 PointsHassan Al Manawy, Your explanation is very helpful. Thanks

Ron Del Rosario
3,331 PointsSame thing I watched all the videos but did not check the teacher notes, since this is the first time they put on a challenge with the solution not on the video.
Anyways, still thankful for teamtreehouse active community of students. :)

Griffin McKesson
2,805 PointsWhere does .children come from? And what does it mean?

colin Peters
5,895 PointsI agree this challenge was a little off topic from the videos i was a little lost and thrown off with this one. don't get how somehow landed on the .child part either don't remember that being explained
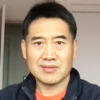
William Ma
Front End Web Development Techdegree Graduate 12,958 PointsI use below code, can get the good result, but the quiz said: Bummer: There was an error with your code: TypeError: 'undefined' is not an object (evaluating 'listItems[i]') my code as blew: //var listItems; var colors = ["#C2272D", "#F8931F", "#FFFF01", "#009245", "#0193D9", "#0C04ED", "#612F90"];
const listItems=document.querySelectorAll('li');
for(var i = 0; i < colors.length; i ++) {
listItems[i].style.color = colors[i];
}
the browser shows the text below with the correct colors: This should be red This should be orange This should be yellow This should be green This should be blue This should be indigo This should be violet
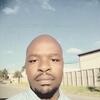
Kudakwashe Jama
7,676 Pointsvar const = document.getElementById('rainbow').children;

Alan Lane
9,156 PointsThis is the code I used to pass the challenge:
var listItems = document.querySelectorAll('#rainbow li'); var colors = ["#C2272D", "#F8931F", "#FFFF01", "#009245", "#0193D9", "#0C04ED", "#612F90"];
for(var i = 0; i < colors.length; i ++) {
listItems[i].style.color = colors[i];
}
Benjamin Kennerly
5,489 PointsBenjamin Kennerly
5,489 PointsHere for the same reason. Always fun when this happens...
Michael Brown
17,651 PointsMichael Brown
17,651 PointsYou can find it if you look at the Mozilla Developer Network, but I agree. Sometimes the questions/ challenges are not always explained well. Still love Treehouse though!