Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial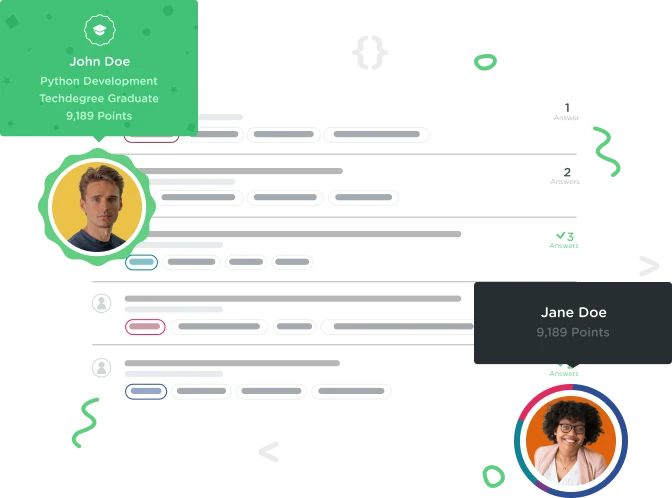

Javier Leal
248 PointsThe frog does not move when i press the arrow keys
using UnityEngine; using System.Collections;
public class PayerMovement : MonoBehaviour {
private Animator playerAnimator;
private float moveHorizontal;
private float moveVertical;
private Vector3 movement;
// Use this for initialization
void Start () {
playerAnimator = GetComponent<Animator> ();
}
// Update is called once per frame
void Update () {
moveHorizontal = Input.GetAxisRaw ("Horizontal");
moveVertical = Input.GetAxisRaw ("Vertical");
movement = new Vector3 (moveHorizontal, 0.0f, moveVertical);
}
void FixedUpdate () {
if (movement != Vector3.zero) {
playerAnimator.SetFloat ("speed", 3F);
} else {
playerAnimator.SetFloat ("speed", 0f);
}
}
10 Answers
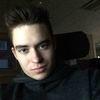
Simon Andersson
6,880 PointsDoes the animation play? The script looks good. Make sure the script is attached to the player game object.
The game object should have these components:
Transform
Animator
Rigidbody
Box Collider
Player Movement (script)
Referenced this as C# code in order to enhance readability since new line didn't work here. They should be attached to your Player GameObject.
Also, make sure the player gameobject is not stuck in the ground. Try lifting it up in the Y axis a little bit and see if it moves.
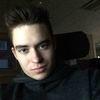
Simon Andersson
6,880 PointsYou are actually really close. There is just one tiny thing you need to change in the FixedUpdate() method.
void FixedUpdate () { if (movement != Vector3.zero) { playerAnimator.SetFloat ("speed", 3F); } else { playerAnimator.SetFloat ("speed", 0f); } }
In these two lines where you set the float, you have to use a capital S in the variable "Speed", such as
void FixedUpdate () { if (movement != Vector3.zero) { playerAnimator.SetFloat ("Speed", 3f); } else { playerAnimator.SetFloat ("Speed", 0f); } }

Javier Leal
248 Pointsi changed it and it still does not move

Javier Leal
248 Pointsusing UnityEngine; using System.Collections;
public class PayerMovement : MonoBehaviour {
private Animator playerAnimator;
private float moveHorizontal;
private float moveVertical;
private Vector3 movement;
// Use this for initialization
void Start () {
playerAnimator = GetComponent<Animator> ();
}
// Update is called once per frame
void Update () {
moveHorizontal = Input.GetAxisRaw ("Horizontal");
moveVertical = Input.GetAxisRaw ("Vertical");
movement = new Vector3 (moveHorizontal, 0.0f, moveVertical);
}
void FixedUpdate () {
if (movement != Vector3.zero) {
playerAnimator.SetFloat ("Speed", 3f);
} else {
playerAnimator.SetFloat ("Speed", 0f);
}}
}
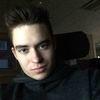
Simon Andersson
6,880 PointsIf the animation still plays but it doesnt move when you have lifted it up, check your Animator component in the inspector, and see if the Avatar field has any value. If not, set it to frogAvatar.
If the animation doesnt play at all, check the Animator component again and see if the Comtroller field has a value, if not, set this to PlayerController. You do this by clicking the small circle next to the field.

Javier Leal
248 Pointsthe animation plays but the frog does not move

Javier Leal
248 Pointsit says parameter speed does not exist
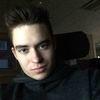
Simon Andersson
6,880 PointsSounds weird. Try deleting the parameters and then write them back. Like this
void FixedUpdate()
{
if (movement != Vector3.zero)
{
playerAnimator.SetFloat
}
else
{
playerAnimator.SetFloat
}
}
Then Save Script, then write them back
void FixedUpdate()
{
if (movement != Vector3.zero)
{
playerAnimator.SetFloat ("Speed", 3f);
}
else
{
playerAnimator.SetFloat ("Speed", 0f);
}
}
And Save script again
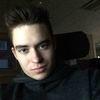
Simon Andersson
6,880 PointsAlso, sometimes in Unity the warning messages in the corner doesn't go away immediately when you fix them. I opened Unity and put together the script and components. It should look like this.
http://gropio.com/stek/file/fmx9kv
The script works as well and the frog moves forward.

Javier Leal
248 Pointsusing UnityEngine; using System.Collections;
public class PayerMovement : MonoBehaviour {
private Animator playerAnimator;
private float moveHorizontal;
private float moveVertical;
private Vector3 movement;
// Use this for initialization
void Start () {
playerAnimator = GetComponent<Animator> ();
}
// Update is called once per frame
void Update () {
moveHorizontal = Input.GetAxisRaw ("Horizontal");
moveVertical = Input.GetAxisRaw ("Vertical");
movement = new Vector3 (moveHorizontal, 0.0f, moveVertical);
}
void FixedUpdate () {
if (movement != Vector3.zero) {
playerAnimator.SetFloat ("speed", 3F);
} else {
playerAnimator.SetFloat ("speed", 0f);
}}
}

Javier Leal
248 Pointsi did what you said and mine is the same as yours but it does not move
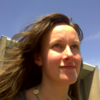
Annet de Boer
900 Points"speed" should be with capital S: "Speed"
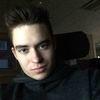
Simon Andersson
6,880 PointsLooks like you're missing a '}' in the last script you posted. However, try replacing you're code with this.
private Animator playerAnimator;
private float moveHorizontal;
private float moveVertical;
private Vector3 movement;
// Use this for initialization
void Start()
{
playerAnimator = GetComponent<Animator>();
}
// Update is called once per frame
void Update()
{
moveHorizontal = Input.GetAxisRaw("Horizontal");
moveVertical = Input.GetAxisRaw("Vertical");
movement = new Vector3(moveHorizontal, 0.0f, moveVertical);
}
void FixedUpdate()
{
if (movement != Vector3.zero)
{
playerAnimator.SetFloat("Speed", 3f);
}
else
{
playerAnimator.SetFloat("Speed", 0f);
}
}
}
If it doesn't work, it's not the script. I guess you've already tried restarted Unity. If you use this script, attach it to your Player gameobject (the frog), make sure the frog is not stuck in the ground and it still doesn't work, it sounds like its either a bug or that one of your components is missing something or has a incorrect value of something.

Javier Leal
248 Pointsit says
Can't add script component 'PlayerMovement' because the script class cannot be found. Make sure that there are no compile errors and that the file name and class name match.

Cameron Button
1,084 PointsI'm having similar problem
```using UnityEngine; using System.Collections;
public class PlayerMovement : MonoBehaviour {
private Animator playerAnimator;
private float moveHorizontal;
private float moveVertical;
private Vector3 movement;
// Use this for initialization
void Start () {
playerAnimator = GetComponent<Animator> ();
}
// Update is called once per frame
void Update () {
moveHorizontal = Input.GetAxisRaw ("Horizontal");
moveVertical = Input.GetAxisRaw ("Vertical");
movement = new Vector3 (moveHorizontal, 0.0f, moveVertical);
}
void FixedUpdate () {
if (movement != Vector3.zero) {
playerAnimator.SetFloat("Speed", 3f);
} else {
playerAnimator.SetFloat("Speed", 0f);
}
}
}```
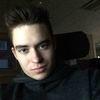
Simon Andersson
6,880 PointsOh, that's probably because you're missing this at the top of the script
using UnityEngine; using System.Collections;
public class PayerMovement : MonoBehaviour {

Dylan Carter
4,780 Pointseveryone its okay ive arrived with the answer.
change the players y value, it may be "running into" the terrain. I set mine to 1.25 and it works good

Lucas Khor
1,888 PointsI checked the Apply Root Motion under Animator component and it works.
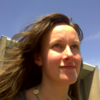
Annet de Boer
900 Points"speed" should be with capital S: "Speed" and I'm guessing 3F is not the same as 3f: playerAnimator.SetFloat("Speed", 3f);