Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial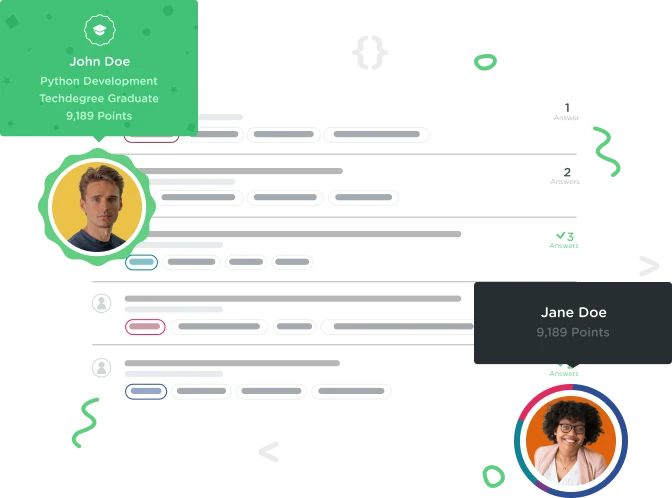
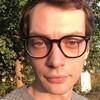
Tomasz Grodzki
8,130 PointsThe function addNewTeachers() works without any parameters. So, why should I create any parameters in this function?
I deleted a parameter newTeachers from addNewTeachers() function and it works the same like with this parameter. So why should I bother about adding any parameter?
let newTeachers = [
{
name: 'James',
topicArea: 'Javascript'
},
{
name: 'Treasure',
topicArea: 'Javascript'
}
];
const teachers = [
{
name: 'Ashley',
topicArea: 'Javascript'
}
];
const courses = ['Introducing JavaScript',
'JavaScript Basics',
'JavaScript Loops, Arrays and Objects',
'Getting Started with ES2015',
'JavaScript and the DOM',
'DOM Scripting By Example'];
const i = courses.length;
function addNewTeachers() {
for ( let i = 0; i < newTeachers.length; i++ )
teachers.push ( newTeachers[i] );
}
function printTreehouseSummary() {
// TODO: fix this function so that it prints the correct number of courses and teachers
for (let i = 0; i < teachers.length; i++) {
console.log(`${teachers[i].name} teaches ${teachers[i].topicArea}`);
}
console.log(`Treehouse has ${i} JavaScript courses, and ${teachers.length} Javascript teachers`);
}
addNewTeachers();
printTreehouseSummary();
1 Answer
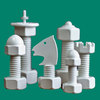
Steven Parker
231,269 PointsThe advantage of the parameter would be that when you had several arrays of teachers to add, you could call it once for each one, passing it as the argument.
It would also be useful in situations where the array to be added is not in the global scope, so the function cannot access it directly.
Tomasz Grodzki
8,130 PointsTomasz Grodzki
8,130 PointsOh I see. So in this case I can leave the function without this parameter and it isn't mistake? Or maybe are there some rules which says that I should anyway put a parameter in parentheses?
Steven Parker
231,269 PointsSteven Parker
231,269 PointsAs you already discovered, the parameter isn't necessary to make this particular case work. But you should have one because that's what the assignment specifically asked for.