Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial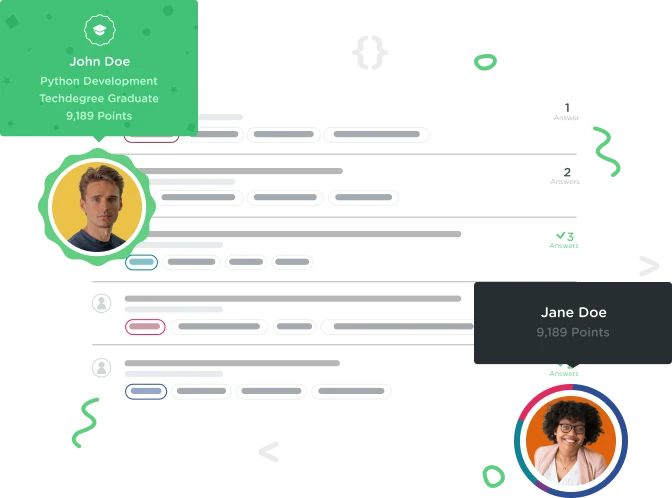
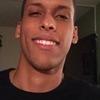
Christian A. Castro
30,501 PointsThe function disemvowel takes a single word as a parameter and then returns that word at the end.What is wrong??
What might be wrong?
def disemvowel(word):
word = ["e", "i", "o", "u"]
for vocals in word:
if vocals.lower() or vocals.upper() in word:
word.remove(vocals)
return word
2 Answers

Luke Strama
6,928 PointsThere's a few things to address here. To start, the 'word' argument should not be equal to the vowels (which should have 5 chars, you only have 4). Given that word will be a string and strings aren't iterable, you'll need to convert the word that passes in into something that would iterable (think list of letters...).
Once you have both of those, you can start looking at ways to run a for loop to compare the two lists. There's another question answered much better than I can that can help you work through that.
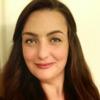
Jennifer Nordell
Treehouse TeacherHi there! Luke Strama has left some good tips for you! However, I'd like to point out that strings are iterable.
That being said, he's correct. You're writing over the value that Treehouse is sending in. The variable word
will be assigned whatever they send. On your very first line, you write over it with a list containing 4 of the 5 vowels. You are missing the "a" from that list.
That being said, if you want to use the remove
function, you will have to turn word
into a list. The remove
function is not available on strings. Keep in mind, though, that if you choose to use the remove
function it is almost never a good idea to mutate the thing you are iterating over. You might consider making a copy and iterating over the copy while mutating the original. If you do this, you will have to use the join
method to turn your list back into a string as the challenge requires a string to be returned.
Keep in mind that there are several valid solutions to this problem and there's at least one that doesn't require the use of a list at all.
Hope this helps!
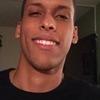
Christian A. Castro
30,501 PointsThank you so much for your quick response, Jennifer Nordell !!! It makes totally sense..
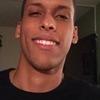
Christian A. Castro
30,501 PointsJennifer Nordell following your advise, I made a few corrections however I still receiving an error message. Any feedback or any particular method I should call? I think that I'm skipping certain iterable elements, but I'm not quite sure ..
def disemvowel(word):
newList = list(word)
vowels = ["a", "e", "i", "o", "u"]
for letter in newList:
if letter.lower() in vowels:
newList.remove(letter)
word = join(newList)
return word