Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial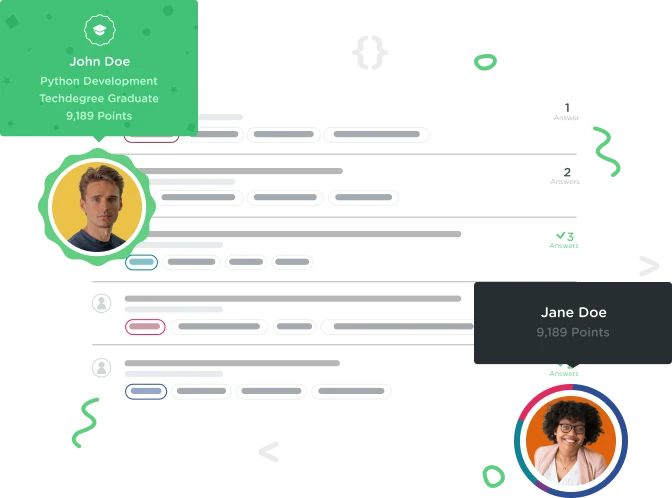

Justine Lam
8,785 PointsThe function example is pretty confusing
Okay, so I think I understand what's going on now. I think it's just confusing that he re-uses this function at the top and bottom:
int funky_math(int a, int b);
Doesn't seem very DRY to have that repeated at the bottom. I commented out the top portion and moved the bottom function up to the top:
int funky_math(int a, int b) {
return a + b + 343;
}
int main()
{
int foo = 24;
int bar = 35;
printf("funky math %d\n", funky_math(foo, bar));
return 0;
}
2 Answers

Justine Lam
8,785 PointsThis is what the code looked like originally:
int funky_math(int a, int b);
int main()
{
int foo = 24;
int bar = 35;
printf("funky math %d\n", funky_math(foo, bar));
return 0;
}
int funky_math(int a, int b) {
return a + b + 343;
}
So I need to have the initial function declared and then repeat it at the bottom with the actual math?
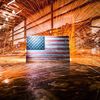
Andrew Shook
31,709 PointsIt depends on the compiler and version of the compiler you are using. I believe you can get away with declaring and defining all in one step with some, but your program may not run on all computers if you do that. However, if you want to maintain "best practices" then yes you need to declare it at the top and define it at the bottom.

Stone Preston
42,016 Pointsif you dont want to include the function prototype (this line is the prototype: int funky_math(int a, int b); ) then you would have to define your function before using it like so:
int funky_math(int a, int b) {
return a + b + 343;
}
int main()
{
int foo = 24;
int bar = 35;
printf("funky math %d\n", funky_math(foo, bar));
return 0;
}
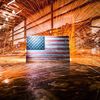
Andrew Shook
31,709 PointsJustine Lam, he isn't reusing the code he is declaring it. This has to do with the way a C compiler works. In order to set aside enough memory for a program the compiler needs to know what kinda of data it is working with. So all data must be declared( usually at the top of a file) before it can be defined. It has been a while since I programmed anything in C but I believe they changed this in the 2011 standards. Also, its kind of tradition in C to write a program that way.
Stone Preston
42,016 PointsStone Preston
42,016 Pointscan you post the code that is being used?