Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial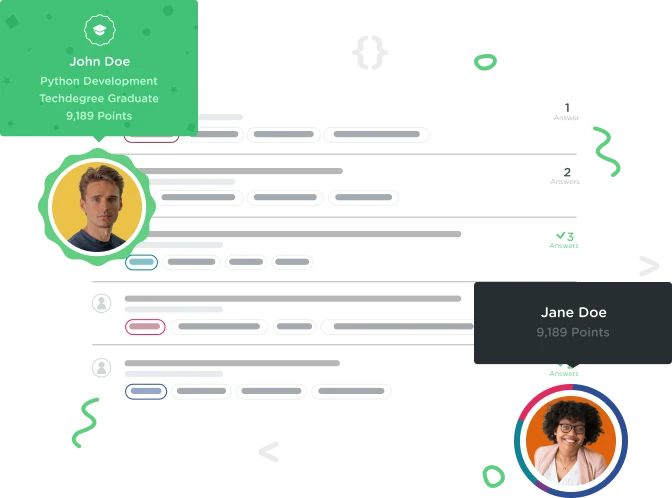

Audra Ogilvy
3,142 PointsThe function finds the variable in the global scope?
After taking var out of the function, making person = 'Lilah' instead of var person = 'Lilah', Dave says, "the function is looking for a variable in the global scope." At 2:40 in the video, Dave says, "It finds one". But it looks like it finds it in the local scope, not the global scope. Confusing. Also, how is person (without var in front of it) still a variable? In a prior question someone said the equals sign turns the object to its left into a variable. But it sounds as though by removing the var, the word "person", which is inside the function, becomes a GLOBAL variable instead of function variable, just because the var designation is gone, even though it's still within the function. Is that correct? This needed more explanation and more time spent on the lesson to make it clear enough.
4 Answers

Enemuo Felix
1,895 PointsFrom Marcin's explanations above , specifically the last example var person = 'Lilah'; //global variable
function callName() { person = 'George'; //now you set value 'George' to global variable person alert(person); } callName(); //alert with name George alert(person); //alert with name George I was wondering why the code alert(person); didn't pick the global variable (Lilah) but instead George which is in a local scope. Can anybody explain this?

Marcin Czachor
9,641 PointsHey, it happens like this because you do not declare another variable (in 2nd function you do not have 'var' keyword), you just set new value to person variable declared in global scope. I hope it helps. :)

Enemuo Felix
1,895 PointsThanks George. Yes I know the function is now global because of the exclusion of the VAR keyword but the var person = Lilah; at the top is also a global scope but why will the alert function run the former since both are global?

Marcin Czachor
9,641 PointsFirst of all you have to check what is happening in 2nd example. Lets consider only this:
var person = 'Lilah'; //global variable
function callName() {
person = 'George'; //now you set value 'George' to global variable person
alert(person);
}
callName(); //alert with name George
alert(person); //alert with name George
You define global variable person with value of 'Lilah'. Then you declare a function which changes value of person to 'George' and alert it. Then you invoke function which alert George. Last step you alert person variable which value has already been changed to 'George'.

Enemuo Felix
1,895 PointsOk. If i understand you correctly, you mean by calling the function( callName; ) first before the alert makes it run the Global variable inside the function(George) and using the alert function without calling the function( callName; ) makes it run the Global variable outside the function(Lilah) ?

Marcin Czachor
9,641 PointsYes, exactly. Order of invoked function callName and alert matter, because calling a function with parentheses forces javascript interpreter to do what is within the body of the function callName.

Enemuo Felix
1,895 PointsThanks Mate
Marcin Czachor
9,641 PointsMarcin Czachor
9,641 PointsHey there! Functions seeks variables first in local scope (inside function), if variable is not found then it searches in global scope. If you define variable in a function and call it in function then that variable exists only in that function. If you omitt var keyword in variable declaration inside a function then it is just setting new value to the variable defined in global scope.