Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial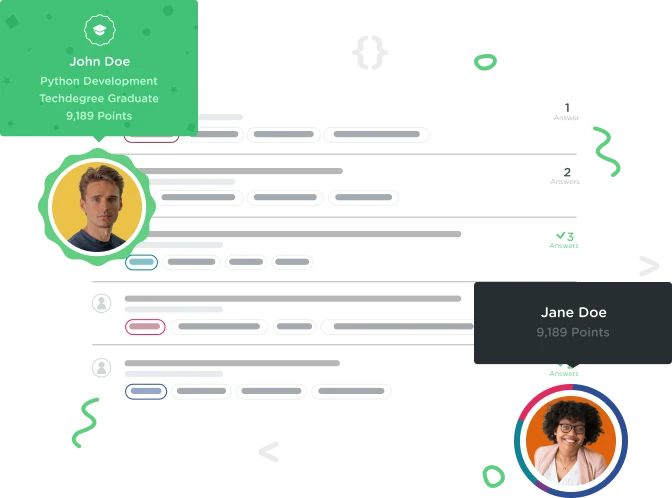

Alan Mills
31,712 Points"The given key was not present in the dictionary."
Excuse the multiple writing to the console. But, I was trying to confirm that I was searching by the key (string) to obtain (& update) the value.
It feels like I'm accessing and updating the value. But, I don't understand what I need to fix.
Thanks!
using System;
using System.Collections.Generic;
namespace Treehouse.CodeChallenges
{
public class LexicalAnalysis
{
public Dictionary<string, int> WordCount = new Dictionary<string, int>();
public void AddWord(string word)
{
int newCount = WordCount[word] + 1;
Console.Write(word);
Console.Write(WordCount[word]);
if(WordCount.ContainsKey(word))
{
WordCount[word] = newCount;
Console.Write(WordCount[word]);
// WordCount[word] = newCount;
}
}
}
}
3 Answers
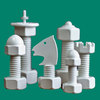
Steven Parker
231,545 PointsYou probably don't want to create "newCount" until after you check that the word exists in the dictionary. If not, trying to access the current value causes the error you are seeing.
Then, if the value does not already exist you'll want to add a new item with a value of 1.

Alan Mills
31,712 PointsAhhhhhh. Didn’t think of that! Will try it when I have a moment. Thanks!

Alan Mills
31,712 PointsUnfortunately, this didn't solve it. But I also figured it would. I'm somehow not actually accessing the dictionary keys. Same error prompt. I think it's in the if statement...
====================== using System.Collections.Generic; using System;
namespace Treehouse.CodeChallenges { public class LexicalAnalysis { public Dictionary<string, int> WordCount = new Dictionary<string, int>();
public void AddWord(string word)
{
int newCount = 0;
if (WordCount.ContainsKey(word))
{
newCount = WordCount[word] + 1;
WordCount[word] = newCount;
}
WordCount.Add(word, newCount);
Console.WriteLine(WordCount[word]);
Console.WriteLine(word);
}
}
}
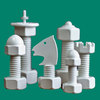
Steven Parker
231,545 PointsYou won't need to add an item again if it exists. But if the value does not already exist you still need to add a new item with a value of 1. So you probably need an "else" to go with the "if".