Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial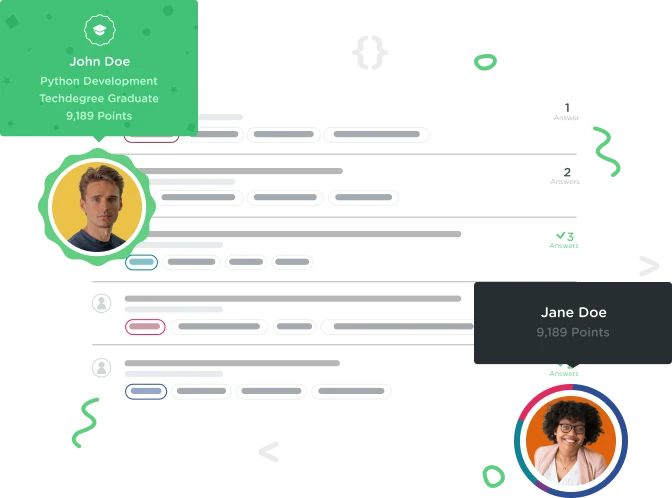
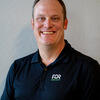
Matthew Turner
Full Stack JavaScript Techdegree Graduate 16,968 PointsThe if/else block doesn't work. Only using the switch allows a new user to be created. Typos in the code, beware.
Everything was going great until I got to the very end. When Reggie logs himself out and then signs Guil up as a new user, I tried that as well in my app. I am using the if/else statement as opposed to the switch that Reggie used. I copied the if/else statement from the teachers notes. In order to use the if/else statement, you must correct a few typos. Going by line, on line 13 in UserSignUp.js
, make sure that your code looks as follows:
const [username, setUser] = useState('');
Next, from line 16 - 27 (when using the if/else statement), should be this:
const change = (event) => {
const value = event.target.value;
if (event.target.name === "name") {
setName(value);
} else if (event.target.name === "username") {
setUser(value);
} else if (event.target.name === "password") {
setPass(value);
} else {
return;
}
}
username
was written as userName
in the teacher's notes and password
was written as name
.
That should do it unless you possibly wrote userName
anywhere else in your code. Double check if it's still not working for you. I got mine to run and here is the code in its entirety:
import React, { useState, useContext} from 'react';
import { Link, useHistory} from 'react-router-dom';
import Form from './Form';
import Context from '../Context'
export default function UserSignUp() {
const context = useContext(Context.Context)
let history = useHistory();
const [name, setName] = useState('');
const [username, setUser] = useState('');
const [password, setPass] = useState('');
const [errors,setErrors] = useState([]);
const change = (event) => {
const value = event.target.value;
if (event.target.name === "name") {
setName(value);
} else if (event.target.name === "username") {
setUser(value);
} else if (event.target.name === "password") {
setPass(value);
} else {
return;
}
}
const submit = () => {
// Create user
const user = {
name,
username,
password,
};
context.data.createUser(user)
.then( errors => {
if (errors.length) {
setErrors(errors)
} else {
context.actions.signIn(username, password)
.then(() => {
history.push('/authenticated');
});
}
})
.catch((err) => {
console.log(err);
// context.history.push('/error');
});
}
const cancel = () => {
context.history.push('/');
}
return (
<div className="bounds">
<div className="grid-33 centered signin">
<h1>Sign Up</h1>
<Form
cancel={cancel}
errors={errors}
submit={submit}
submitButtonText="Sign Up"
elements={() => (
<React.Fragment>
<input
id="name"
name="name"
type="text"
onChange={change}
placeholder="Name" />
<input
id="username"
name="username"
type="text"
onChange={change}
placeholder="User Name" />
<input
id="password"
name="password"
type="password"
onChange={change}
placeholder="Password" />
</React.Fragment>
)} />
<p>
Already have a user account? <Link to="/signin">Click here</Link> to sign in!
</p>
</div>
</div>
);
}
cc @Reggie
2 Answers
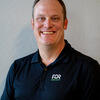
Matthew Turner
Full Stack JavaScript Techdegree Graduate 16,968 PointsThank you for the Exterminator badge. I will do my best to continue to spot any other issues as I progress through the TechDegree.
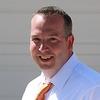
Lee Vaughn
Treehouse TeacherHi Matthew Turner!
Kudos on spotting these issues and for coming up with a solution. I have passed the typos along to our Education team and we will work on getting them fixed asap.
As a thank you for pointing out these bugs we have awarded you the exclusive Exterminator badge! Thanks again!
Lee