Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial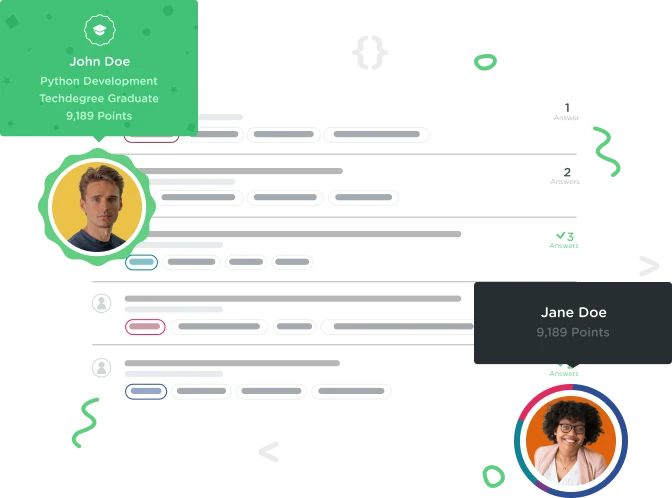

Sayuri Katz
6,148 Pointsthe keyboard doesn't show up.
import UIKit
class ViewController: UIViewController {
@IBOutlet weak var nameTextField: UITextField!
@IBOutlet weak var textFieldBottomConstraint: NSLayoutConstraint!
override func viewDidLoad() {
super.viewDidLoad()
NotificationCenter.default.addObserver(self, selector: #selector(ViewController.keyboardWillShow(_:)), name: Notification.Name.UIKeyboardWillShow, object: nil)
NotificationCenter.default.addObserver(self, selector: #selector(ViewController.keyboardWillHide(_:)), name: .UIKeyboardWillHide, object: nil)
func keyboardWillHide(_ notification: Notification) {
textFieldBottomConstraint.constant = 40
UIView.animate(withDuration: 0.8) {
self.view.layoutIfNeeded()
}
}
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
override func prepare(for segue: UIStoryboardSegue, sender: Any?) {
if segue.identifier == "startAdventure" {
do {
if let name = nameTextField.text {
if name == "" {
throw AdventureError.nameNotProvided
} else {
guard let pageController = segue.destination as? PageController else { return }
pageController.page = Adventure.story(withName: name)
}
}
} catch AdventureError.nameNotProvided {
let alertController = UIAlertController(title: "Name Not Provided", message: "Provide a name to start the story", preferredStyle: .alert)
let action = UIAlertAction(title: "OK", style: .default, handler: nil)
alertController.addAction(action)
present(alertController, animated: true, completion: nil)
} catch let error {
fatalError("\(error.localizedDescription)")
}
}
}
func keyboardWillShow(_ notification: Notification) {
if let info = notification.userInfo, let keyboardFrame = info [UIKeyboardFrameEndUserInfoKey] as? NSValue {
let frame = keyboardFrame.cgRectValue
textFieldBottomConstraint.constant = frame.size.height + 10
UIView.animate(withDuration: 0.8) {
self.view.layoutIfNeeded()
}
}
}
func keyboardWillHide(_ notification: Notification) {
textFieldBottomConstraint.constant = 40
UIView.animate(withDuration: 0.8) {
self.view.layoutIfNeeded()
}
}
deinit {
NotificationCenter.default.removeObserver(self)
}
}
extension ViewController: UITextFieldDelegate { func textFieldShouldReturn(_ textField: UITextField) -> Bool { textField.resignFirstResponder() return true } }
1 Answer
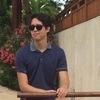
Luis Corvalan
iOS Development Techdegree Student 1,752 PointsHi! What is working for me is to go to override func viewDidLoad() { } and inside it write, yourTextField.becomeFirstResponder()