Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial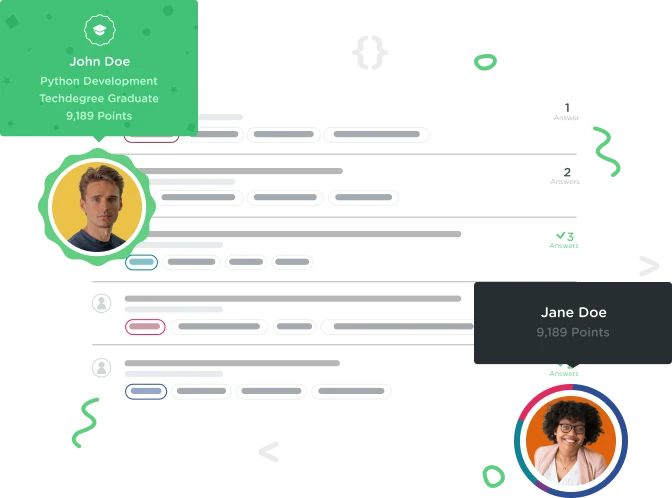

Christian Pineault
7,291 PointsThe last Object-Oriented PHP Basics code challenge.
I'm having trouble with the last step of the code challenge. The instructions are: Return an array containing the public lot parameter of each house object whose public roof_color OR wall_color match the passed color parameter.
Now I created a public $houses parameter but I have no idea where the lot parameter of each house object comes from. I don't know how to create that array. I'm pretty sure there is a foreach loop involved but other than that I don't know.
Any ideas? Thanks
2 Answers

filipepacheco
Full Stack JavaScript Techdegree Student 21,416 PointsHi, Christian Pineault, I also had a hard time on this one.
Imagine that the $houses array you had to create on the first step is an array with many house objects. Now imagine that each house object has some public properties, and that some of these properties are $house->lot, $house->roof_color and $house->wall_color.
So to return an array containing the public lot property of each house object, as you said, you first need a foreach loop to get each house object from the $houses array you created. Remember to create an array of matched colors houses.
public $houses = [];
public function filterHouseColor($color)
{
$matchedColorsHouses = [];
foreach ($this->houses as $house) {
}
}
Now you need to see if the $color parameter from the method matches the public properties roof_color or wall_color from the $house object you just got, then assign the lot property to the $matchedColorsHouses array and return it, outside the foreach loop.
public $houses = [];
public function filterHouseColor($color)
{
$matchedColorsHouses = [];
foreach ($this->houses as $house) {
if($house->roof_color == $color || $house->wall_color == $color){
$matchedColorsHouses[] = $house->lot;
}
}
return $matchedColorsHouses;
}

Christian Pineault
7,291 PointsThank you, when I finally understood that $houses was an array of $house objects pretty much everything clicked. Thanks again.
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsHi Filipe,
The logic for your
if
condition isn't quite right. On the right side of the logical or operator you only have a string which is the color. This string will evaluate to true which causes the whole condition to always be true. Essentially you're adding every house lot to the array.It should be more like this:
if($house->roof_color == $color || $house->wall_color == $color){
I think something is wrong with the tester and it's allowing incorrect answers to pass.
filipepacheco
Full Stack JavaScript Techdegree Student 21,416 Pointsfilipepacheco
Full Stack JavaScript Techdegree Student 21,416 PointsHi, Jason Anello,
I had no clue about that. I even recreated everything to test and understand it better, and could see what you meant. I just don't understand why it would always return true. Does it mean that just by the fact of having an string on the right side of that logical, it would return true, regardless of the value? Then should I always use the parameter on the right side?
Thank you very much for your help.
I've edited and corrected my answer.
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsI think you originally had something like
if($color == $house->roof_color || $house->wall_color){
When we read that it seems like it would work out. We want to know if the color is equal to either the roof_color or the wall_color but this is not how the php interpreter sees it.
It has to follow a certain order of operations. See Operator Precedence Looking at that you can see that the
==
operator has higher precedence than the||
operator so it has to do the equal operator first.That will be true or false depending on whether the color matches the roof color.
If the left side is true then the whole thing is true because it's logical or, if the left side is false then it has to evaluate the right side of the logical or. But it's just a string like "white"
Only the empty string and the string "0" are false when converted to boolean. All other strings are true. See here http://php.net/manual/en/language.types.boolean.php for a list of everything that is considered false in php.
The non-false string on the right side insures that the whole condition is always true.
filipepacheco
Full Stack JavaScript Techdegree Student 21,416 Pointsfilipepacheco
Full Stack JavaScript Techdegree Student 21,416 PointsI kind of got an idea now. So it doesn't have anything to do with the order of the parameter, but the fact that I added the || operator in that comparison. So adding the || makes the conditional evaluate if it's true or false, regardless of the value. Then of course, only an empty string and 0 would return false.
I think I came across a similar situation when I was doing some exercises on my own and couldn't figure out what was going on. Now I get it.
Thank you very much for taking time and helping. I appreciate it.