Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial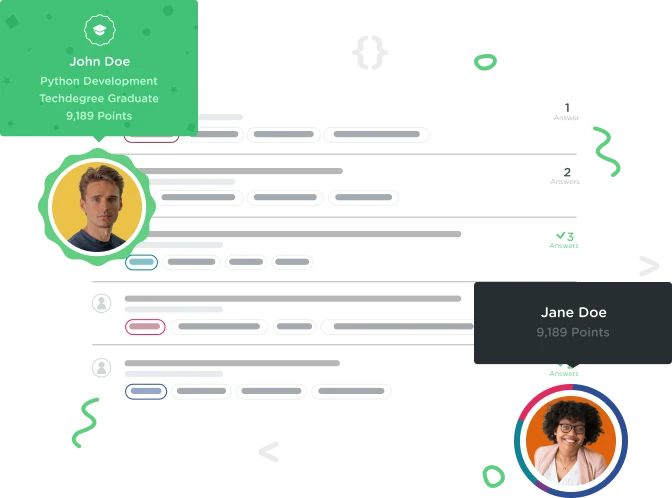

Nate Drexler
1,883 PointsThe last part of this code challenge has me a little confused.
I believe I have the first two constants in correct syntax. And I think I have the product constant correct. I'm just not sure how to make these a string literal.
// Enter your code below
let firstValue: Int = 10
let secondValue: Int = 20
let product: Int = firstValue * secondValue
let output =
1 Answer
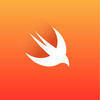
Steven Deutsch
21,046 PointsHey Nate Drexler,
A string literal is just the actual representation of a String, a String itself. To create the output message we will create a string literal and then use String Interpolation to extract the values from our variables above. To create a String literal you just put some text between two quotes. To use string interpolation you wrap the name of the variable/constant in parentheses and then proceed it with a backslash. This will take whatever value is stored inside that variable or constant and add it into the String literal as a String.
// Enter your code below
let firstValue = 10
let secondValue = 20
let product = firstValue * secondValue
let output = "The product of \(firstValue) times \(secondValue) is \(product)"
Also note, you don't have to explicitly define the type for your variables and constants. Swift has great type inference. If you assign a String literal to a variable/constant, Swift can infer that the type is String. Same goes with other types such as Ints, Doubles, Floats, Bool, Custom types, etc. In some cases you will want to explicitly define a type, however, in most cases its just repetitive.
Good Luck
Nate Drexler
1,883 PointsNate Drexler
1,883 PointsThis is great. Thanks! The reason I'm defining types is because I'm told that it's good practice, at least at the start.
Steven Deutsch
21,046 PointsSteven Deutsch
21,046 PointsYeah its not bad if you're just starting to learn Swift and want to visually understand whats going on. It's not best practice though. The best practice is to let the compiler infer as much as it can.