Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial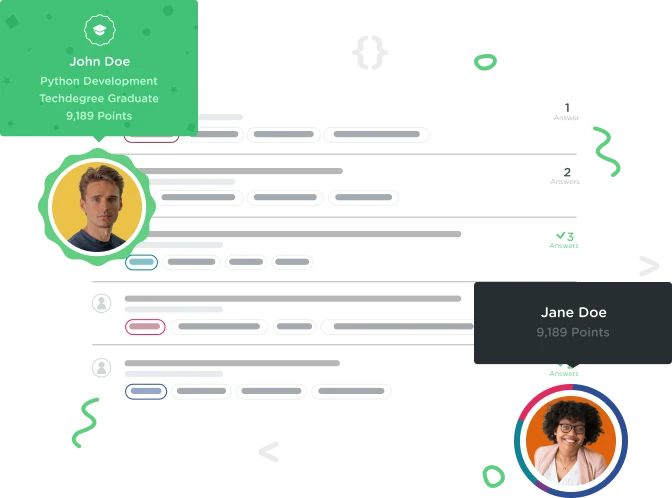
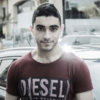
Abdullah Alsowaygh
8,719 PointsThe list is empty or (not displaying any information), and the string "There is no display" is the only thing displayed.
I am unable to make the daily list include the daily data. I have also tried to debug and found that when calling "intent.putExtra(DAILY_FORECAST, mForecast.getDailyForcast());" the getDailyForcast returns information and is not null.
From the layout perspective, I have tried to add constraints to the ListView inside activity_daily_forecast layout, still not helping.
Can someone help please?
1 Answer
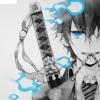
Maximilian Hertelendy
18,148 PointsI had the same issue. I forgot these changes in the DayAdapter
@Override
public int getCount() {
return mDays.length; // <---Check this
}
@Override
public Object getItem(int position) {
return mDays[position]; // <---Check this
}
Abdullah Alsowaygh
8,719 PointsAbdullah Alsowaygh
8,719 Pointspackage stormy.abdullah.stormy.ui;
import android.content.Context; import android.content.Intent; import android.graphics.drawable.Drawable; import android.net.ConnectivityManager; import android.net.NetworkInfo; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.util.Log; import android.view.View; import android.widget.ImageView; import android.widget.ProgressBar; import android.widget.TextView; import android.widget.Toast;
import org.json.JSONArray; import org.json.JSONException; import org.json.JSONObject;
import java.io.IOException;
import butterknife.BindView; import butterknife.ButterKnife; import butterknife.OnClick; import okhttp3.Call; import okhttp3.Callback; import okhttp3.OkHttpClient; import okhttp3.Request; import okhttp3.Response; import stormy.abdullah.stormy.R; import stormy.abdullah.stormy.weather.Current; import stormy.abdullah.stormy.weather.Day; import stormy.abdullah.stormy.weather.Forecast; import stormy.abdullah.stormy.weather.Hour;
public class MainActivity extends AppCompatActivity {
// 24.7136; // 46.6753;
}
package stormy.abdullah.stormy.ui;
import android.app.ListActivity; import android.content.Intent; import android.os.Parcelable; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.util.Log; import android.widget.ArrayAdapter;
import java.util.Arrays;
import stormy.abdullah.stormy.R; import stormy.abdullah.stormy.adapters.DayAdapter; import stormy.abdullah.stormy.weather.Day;
public class DailyForecastActivity extends ListActivity {
}
package stormy.abdullah.stormy.weather;
import stormy.abdullah.stormy.R;
/**
public class Forecast { private Current mCurrent; private Hour[] mHourlyForecast; private Day[] mDailyForcast;
}
package stormy.abdullah.stormy.weather;
import android.os.Parcel; import android.os.Parcelable;
import java.text.SimpleDateFormat; import java.util.Date; import java.util.TimeZone;
import javax.xml.transform.Source;
/**
public class Day implements Parcelable{ private long mTime; private String mSummary; private double mTemperatureMax; private String mIcon;
}