Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial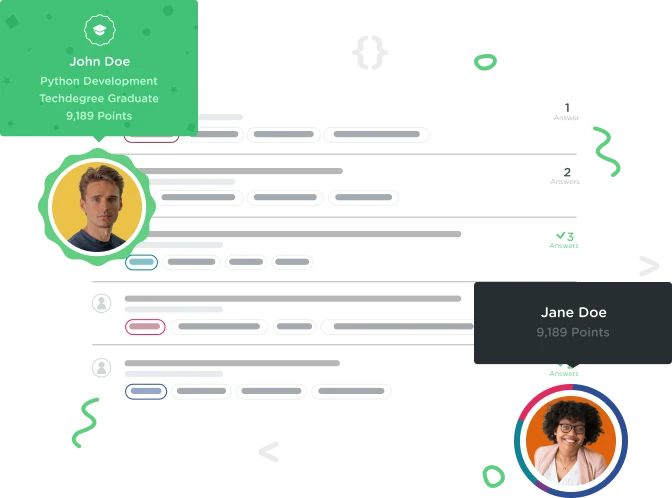
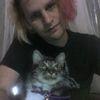
Bryan Wiedeman
10,203 PointsThe login button, it does nothing.
When I click the login button, I don't get an error or any feedback, but it also doesn't log me in. Where am I going wrong?
package me.paxana.alerta;
import android.app.Activity;
import android.app.AlertDialog;
import android.content.Intent;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import com.parse.LogInCallback;
import com.parse.ParseException;
import com.parse.ParseUser;
import com.parse.SignUpCallback;
import butterknife.Bind;
import butterknife.ButterKnife;
import butterknife.OnClick;
public class LoginActivity extends AppCompatActivity {
@Bind(R.id.usernameField) EditText mUsername;
@Bind(R.id.passwordField) EditText mPassword;
@Bind(R.id.loginButton) Button mLoginButton;
@Bind(R.id.signupButton) TextView mSignupButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_login);
ButterKnife.bind(this);
mSignupButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent = new Intent(LoginActivity.this, SignupActivity.class);
startActivity(intent);
mLoginButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String username = mUsername.getText().toString();
String password = mPassword.getText().toString();
username = username.trim();
password = password.trim();
if (username.isEmpty() || password.isEmpty()) { //if they leave a field blank give them an error
AlertDialog.Builder builder = new AlertDialog.Builder(LoginActivity.this);
builder.setMessage(R.string.Login_Error_Message)
.setTitle(R.string.Login_Error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();}
else { //if every field is accounted for, log in.
ParseUser.logInInBackground(username, password, new LogInCallback() {
@Override
public void done(ParseUser user, ParseException e) {
if (e == null) { //if no errors return
Intent intent = new Intent(LoginActivity.this, MainActivity.class); // then we logged in, start the app!
intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
intent.addFlags(Intent.FLAG_ACTIVITY_CLEAR_TASK);
startActivity(intent);} // success }
else {
AlertDialog.Builder builder = new AlertDialog.Builder(LoginActivity.this); //something went wrong logging in
builder.setMessage(e.getMessage()) // get a meaningful error message from parse
.setTitle(R.string.Login_Error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
}
}
});
}
});
}
}
1 Answer

Daniel Hartin
18,106 PointsHi Bryan
Wow that's some crazy long onClick() method bud. I think what has happened here is you have nested the separate onClick methods inside one another when they should be separated.
I have tried my best in the below but please beware that I haven't tested anything.
package me.paxana.alerta;
import android.app.Activity;
import android.app.AlertDialog;
import android.content.Intent;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import com.parse.LogInCallback;
import com.parse.ParseException;
import com.parse.ParseUser;
import com.parse.SignUpCallback;
import butterknife.Bind;
import butterknife.ButterKnife;
import butterknife.OnClick;
public class LoginActivity extends AppCompatActivity {
@Bind(R.id.usernameField) EditText mUsername;
@Bind(R.id.passwordField) EditText mPassword;
@Bind(R.id.loginButton) Button mLoginButton;
@Bind(R.id.signupButton) TextView mSignupButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_login);
ButterKnife.bind(this);
mSignupButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent = new Intent(LoginActivity.this, SignupActivity.class);
startActivity(intent);
}
});
mLoginButton.setOnClickListener(new View.OnClickListener() { //This method here should NOT be nested
@Override
public void onClick(View v) {
tryLogin();
}
});
}
private void tryLogin(){
String username = mUsername.getText().toString();
String password = mPassword.getText().toString();
username = username.trim();
password = password.trim();
if (username.isEmpty() || password.isEmpty()) { //if they leave a field blank give them an error
AlertDialog.Builder builder = new AlertDialog.Builder(LoginActivity.this);
builder.setMessage(R.string.Login_Error_Message)
.setTitle(R.string.Login_Error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}else { //if every field is accounted for, log in.
ParseUser.logInInBackground(username, password, new LogInCallback() {
@Override
public void done(ParseUser user, ParseException e) {
if (e == null) { //if no errors return
Intent intent = new Intent(LoginActivity.this, MainActivity.class); // then we logged in, start the app!
intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
intent.addFlags(Intent.FLAG_ACTIVITY_CLEAR_TASK);
startActivity(intent);
} else { //something went wrong logging in
AlertDialog.Builder builder = new AlertDialog.Builder(LoginActivity.this);
builder.setMessage(e.getMessage()) // get a meaningful error message from parse
.setTitle(R.string.Login_Error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
}
}
}
I separated out the login function into a separate function to try and make the onClick function neater as anonymous inner classes look a little messy anyway imo (try using lambda expressions if you want to make them look neater). This is only to make the code easier to handle in the future as part of the refactoring exercise and you could refactor it further to split out the userinput check and the login step.
Hope this works for you
Let me know if it doesn't and we can try and work through some things
Daniel