Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial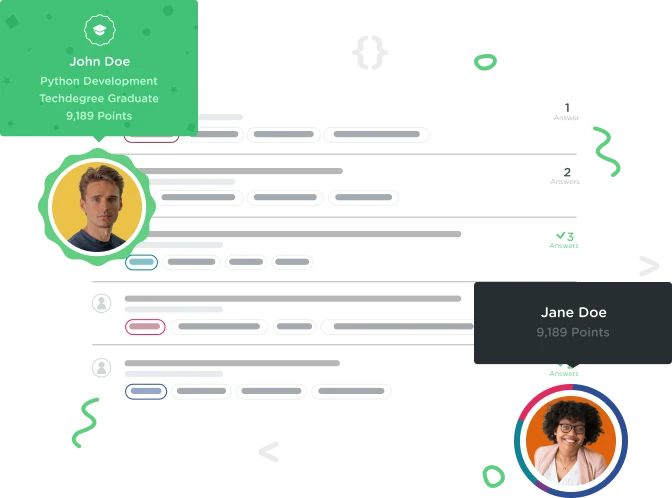

Unsubscribed User
Full Stack JavaScript Techdegree Student 870 Pointsthe max function challenge where I'm supposed to print the larger of the two numbers, getting syntax error?
What am I missing here?
I reckon it could be that the else if statement is redundant, but I don't really know?
function max(one, two){
return one + two;
} if (one > two) {
return one;
} else if (two > one) {
return two;
}
max(2, 4);
3 Answers
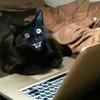
Owen Bell
8,060 PointsThere are a couple of problems here. I'm going to explain what's wrong with your current solution, but see the second half of this response for a more succinct approach.
Firstly, notice that bracket before the if
keyword? That's actually closing off the code block for your function, leaving a lone if/else statement that's attempting to look for the one
and two
variables that have not been defined within its local scope. That's where you're getting your syntax errors. Take the bracket before the if
keyword, and move it to the bottom of the function definition. This will give you a syntactically correct function definition.
Next, the return
statement immediately terminates the code block it's in once its value has been resolved. So once the conditional code has been properly reworked, you still only really have:
function max(one, two){
return one + two;
}
You'll need to remove this line to proceed further into the code block. This leaves us with:
function max(one, two){
if (one > two) {
return one;
} else if (two > one) {
return two;
}
}
Which is a valid submission. Note that your second conditional statement actually isn't necessary for the code in its current form - you could rewrite the else if
as just else
, which would also allow the code to handle cases where the same number is provided as both arguments. In fact, your function will actually fail to return anything when called such that one == two
, so if
/else
would be more suitable than if
/else if
.
Finally, although this is a cheese solution to the challenge, be aware that the JavaScript Math
object has a static method called max
that returns the maximum of (a minimum of) two number-like arguments. This actually does exactly the same thing has the function you're being asked to create here, but can accept more than two arguments, so you could write this:
function max(one, two) {
return Math.max(one, two);
}
to produce the same result. If you were to do this, the only value you would gain from actually writing the function at all in this case is that you can limit the number of arguments to two, and the ability to call a function instead of a method on a global object (and it gets you past the challenge). You might want to do something like this when defining your own object and class methods, however.

Kevin Gates
15,053 PointsHi there, the issue is that you are returning an addition operation, not the max of those 2 numbers. Let's look again: Your original code:
function max(one, two){
return one + two; // HERE you are returning the function with a SUM. Not a max. Everything below this never executes.
} if (one > two) {
return one;
} else if (two > one) {
return two;
}
max(2, 4);
If you get rid of that initial return statement, you should be good.
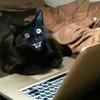
Owen Bell
8,060 PointsActually, everything in this code does try to execute. The condition actually isn't within the function body at all - the stray bracket before the if
statement closes off that function definition. The syntax errors are arising due to the if
statement not having access to one
and two
variables within its own scope due to this fact.
That said, you're completely right that return one + two
should not be there.

Kevin Gates
15,053 PointsAh, yeah, you're right. I sped through this one and missed the closing bracket. Good catch, Owen.

Unsubscribed User
Full Stack JavaScript Techdegree Student 870 PointsOh, I see! I removed the return one + two and moved the closing {} to the end to make it look the following and it worked.
function max(one, two){ if (one > two) { return one; } else { return two; } } max(2, 4);
Thanks, Kevin, I appreciate it.

Unsubscribed User
Full Stack JavaScript Techdegree Student 870 PointsOkay, looking at another course, it seems to me I need to create a variable, but I'm still at a loss :/
Unsubscribed User
Full Stack JavaScript Techdegree Student 870 PointsUnsubscribed User
Full Stack JavaScript Techdegree Student 870 PointsThank you, Owen! This was really helpful as you not only broke down why it didn't execute as intended, but also added the point of the redundant else if I had there.
I feel like I'm doing okay - not good, just okay - at understanding and executing the functions, ifs and returns. I feel like I'm guessing my way forward (see the comment where I thought I maybe had to add a variable, which wasn't going to help me solve the challenge) and this isn't really optimal. Do you know how I can learn this properly?
Maybe I'm objecting and being insecure a bit too early on in this part of the course and perhaps they break it down for me clearer later on, but I thought maybe you have some input on where I can turn to learn this a bit more or better?
Owen Bell
8,060 PointsOwen Bell
8,060 PointsHi, Muhammad. I'm glad my answer was useful to you, thank you for taking the time to write such a kind response :)
As with pretty much every form of structured learning, there will be concepts you encounter that don't necessarily make sense to you right away - one example of this may have been where I referred to "scope" in my answer, something that the course doesn't appear to begin discussing until the next video after this challenge. It's one reason why reviewing what you've learned is important - you can enhance the basics once you've grasped more difficult concepts.
Typically, I feel that Treehouse's HTML/CSS/JS courses and tracks have been pretty well curated (I can't say the same for other topics), and for my experience the only time where I felt like something was missed or unclear was mostly due to me rushing through it. I'd advise you to revisit old challenges and videos if you hit a roadblock like this, to refresh your understanding and maybe pick up something new that didn't click the first time around - trying to glean hints from the wrong sources can lead to further confusion, so exercise some caution there.
Also, be sure to create some of your own Workspaces to play around with the concepts you already know - I find that doing this lets me learn some new things about the language or code design in general - for example, you might write out something similar to the example code above, but leave that first return statement in. By running that code, you'd have learned that the return statement is causing the block to exit straightaway, and you'd have learned something new about how the return statement works!
Programming can become more difficult if you don't have confidence in yourself - remember, when testing your own code you need to trust in your knowledge not only for writing the code initially but also to come up with solutions to any problems that appear. Fortunately, you can have as many goes as you want writing, testing, rewriting and retesting code. Try to make the most of each iteration. If you can't get something to work, take a break, maybe even look at other code for inspiration. The Community is also always here to provide help where we can.
I like to view it this way - in some way, everyone here is practising computer science, and the basis of science is experimentation. It's only through our experimentation, our guessing, that knowledge and understanding can begin to grow. What feels like a random guess is just one small piece of the puzzle - a step towards grasping the complete picture. So don't feel discouraged by guessing - it's an important tool in the learner's arsenal.
TL;DR: don't be scared or discouraged by guessing, keep at it, review your knowledge regularly by revisiting track/course contents, and know that you've got the community to fall back on for support. Best of luck! :)