Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial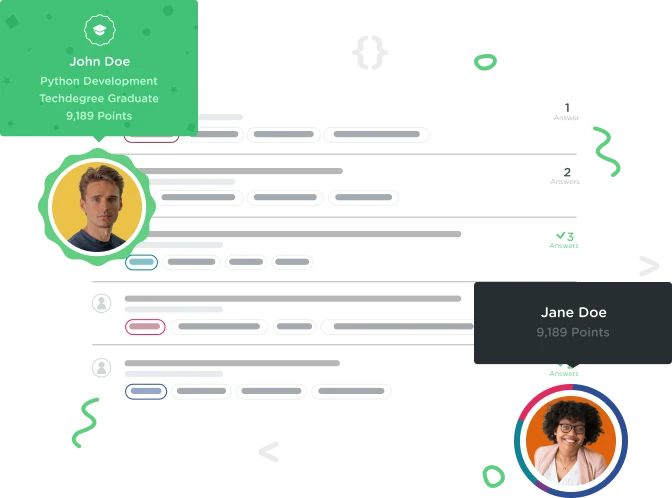

William McManus
14,819 Pointsthe max(x,y) Challenge
I have no idea what to do here. Here is my code:
function max (numOne, numTwo)
if (numOne > numTwo); {
return numOne;
}
else if (numOne < numTwo); {
return numTwo;
}
}
}
max(2,4)
My basic question is even if I get the logic right, how do I get the function to return an integer? I keep getting the error that the function doesn't return a number.
Thanks!
3 Answers
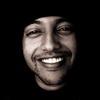
miikis
44,957 PointsHey William,
You have a typo with the opening and closing brackets on your function. You have:
function max(numOne, numTwo)
}
}
When you should have:
function max(numOne, numTwo) {
}
That's probably what's causing the error.
As far as returning an integer, this max() function is going to check the values of numOne and numTwo and return the value of whichever argument has a "greater value". If this operation doesn't make sense, for whatever reason, the function will return nothing — or rather, it will return undefined.

William McManus
14,819 PointsSo what you are saying is that my logic isn't correct and once it is the "return numOne" will essentially be returning a number? Im just not sure how far this wants me to go, and since it is always looking for something specific, not just something that works, I feel like I'm chasing my tail.
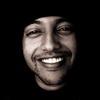
miikis
44,957 PointsI actually just went and did the challenge; it turns out you also forgot to call alert() like it asked. This is the correct answer:
function max(numOne, numTwo) {
if (numOne > numTwo) {
return numOne;
} else if (numTwo > numOne) {
return numTwo;
}
}
alert(max(2, 4));
So, your logic was entirely correct; but there are syntax errors:
- Forgot an opening bracket for the function max()
- You added one extra closing bracket for the function max()
And you forgot to call alert().

William McManus
14,819 PointsOK thanks, The brackets get lost sometimes with al the nesting. I'm still pretty new. I appreciate the help, it turned into one of those feedback errors where I didnt know if it was a logic or syntax error, so one kept being wrong I guess. anyways, thanks!

Bo Christensen
5,924 PointsYou can always add a console.log("Entering function max");, console.log("If statement runs"); Then you can see if something is being skipped in the console.